Question
I have been able to complete the code through step 9, but am having trouble with step 10. My code so far is at the
I have been able to complete the code through step 9, but am having trouble with step 10. My code so far is at the bottom.
In Visual Basic, perform the following:
- Create a form divided vertically in two halves with right and left panels
- In the left panel create the following controls: (the label or the value of the control will be in "" and the type of the control will in []
- "First Number" [textbox]
- "Second number" [textbox]
- "Result" [textbox] - read only
- "+" [push button] "-" [push button] "/" [push button] "*" [push button]
- "Save" [push button] "Display" [push button]
- The user will enter two numbers
- the "First Number"
- the "Second Number",
- Then press the "+" for addition, "-" for subtraction, "*" for multiplication and "/" for division
- The result will be displayed in the result [textbox],
- If the user clicked "[save]" then the operation can be displayed in the right panel when the user press [Display]
- Create two main classes to perform the operations above:
- Create a class for the math ( "+","-") operations -- name the class "MathOp"
- Extend the class using inheritance to include ("*","/") name the class "MathOp2"
- Create the following class/function:
- when a line from the list box is selected, the user should be able to modify the values/operations and save it to the listbox/database Lets say the user clicked on 1+3=4 , the values 1,3 should be displayed in the input text boxes.
- Create the following class/function:
- Add "clear" [push button] on the right panel to clear the content in the results listbox
- Trap all the errors and set the focus to the appropriate control after the error occurred
- The result in the listbox should contain the operations, I.E. the listbox results should look something like this 1+1=2 1*2=2 and so on
- Save and retrieve the operations in Ms. Access database
- Create a table to save the operations
- Create the necessary fields for the table
- Create a button to save the content of the listbox to the database
- Create a button to retrieve the content of the table into the listbox
- The table in the database should not exceed 10 records. When saved, the table should be cleared and replaced with the new operations
- When a line from the list box is selected, the user should be able to modify the values/operations and save it to the listbox/database
- In the left panel create the following controls: (the label or the value of the control will be in "" and the type of the control will in []
My code so far:
Option Explicit On
Option Strict Off
Option Infer Off
Public Class Calculator
'variables
Dim mathOps As MathOp2 = New MathOp2()
Dim int1stNum As Integer = 0
Dim int2ndNum As Integer = 0
Dim intResult As Integer = 0
Dim strSymbol As String
Private Sub btnAdd_Click(sender As Object, e As EventArgs) Handles btnAdd.Click
'Error checking
If (txtFirstNumber.Text Is "" Or txtSecondNumber.Text Is "") Then
MessageBox.Show("Please enter the values")
Else
int1stNum = Integer.Parse(txtFirstNumber.Text)
strSymbol = "+"
int2ndNum = Integer.Parse(txtSecondNumber.Text)
'Calls the class
intResult = mathOps.Add(int1stNum, int2ndNum)
txtResult.Text = intResult.ToString()
End If
End Sub
Private Sub btnSubtract_Click(sender As Object, e As EventArgs) Handles btnSubtract.Click
'Error checking
If (txtFirstNumber.Text Is "" Or txtSecondNumber.Text Is "") Then
MessageBox.Show("Please enter the values")
Else
int1stNum = Integer.Parse(txtFirstNumber.Text)
strSymbol = "-"
int2ndNum = Integer.Parse(txtSecondNumber.Text)
'Calls the class
intResult = mathOps.Subtract(int1stNum, int2ndNum)
txtResult.Text = intResult.ToString()
End If
End Sub
Private Sub btnMultiply_Click(sender As Object, e As EventArgs) Handles btnMultiply.Click
'Error checking
If (txtFirstNumber.Text Is "" Or txtSecondNumber.Text Is "") Then
MessageBox.Show("Please enter the values")
Else
int1stNum = Integer.Parse(txtFirstNumber.Text)
strSymbol = "*"
int2ndNum = Integer.Parse(txtSecondNumber.Text)
'Calls the class
intResult = mathOps.Multiply(int1stNum, int2ndNum)
txtResult.Text = intResult.ToString()
End If
End Sub
Private Sub btnDivide_Click(sender As Object, e As EventArgs) Handles btnDivide.Click
'Error checking
If (txtFirstNumber.Text Is "" Or txtSecondNumber.Text Is "") Then
MessageBox.Show("Please enter the values")
Else
int1stNum = Integer.Parse(txtFirstNumber.Text)
strSymbol = "/"
int2ndNum = Integer.Parse(txtSecondNumber.Text)
If (int2ndNum = 0) Then
MessageBox.Show("Cannot Divide By Zero")
txtSecondNumber.Clear()
txtSecondNumber.Focus()
Else
'Calls the class
intResult = mathOps.Divide(int1stNum, int2ndNum)
txtResult.Text = intResult.ToString()
End If
End If
End Sub
Private Sub btnClear_Click(sender As Object, e As EventArgs) Handles btnClear.Click
'Clears the form
lstCalculations.Items.Clear()
txtFirstNumber.Clear()
txtSecondNumber.Clear()
txtResult.Clear()
txtFirstNumber.Focus()
End Sub
Private Sub btnSave_Click(sender As Object, e As EventArgs) Handles btnSave.Click
Dim strResultant As String
strResultant = int1stNum.ToString & " " & strSymbol & " " & int2ndNum & " = " & intResult.ToString
If (lstCalculations.Items.Count() > 9) Then
MessageBox.Show("Please clear the list to continue")
End If
End Sub
Private Sub btnDisplay_Click(sender As Object, e As EventArgs) Handles btnDisplay.Click
Dim strResultant As String
strResultant = int1stNum.ToString & " " & strSymbol & " " & int2ndNum & " = " & intResult.ToString
lstCalculations.Items.Add(strResultant)
'Clears the form
txtFirstNumber.Clear()
txtSecondNumber.Clear()
txtResult.Clear()
txtFirstNumber.Focus()
End Sub
Private Sub input_validation(sender As Object, e As KeyPressEventArgs) Handles txtFirstNumber.KeyPress, txtSecondNumber.KeyPress
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> "." AndAlso e.KeyChar <> ControlChars.Back Then
e.Handled = True
End If
End Sub
End Class
' MathOp class
Public Class MathOp
Public Function Add(ByVal value1 As Integer, ByVal value2 As Integer) As Integer
Return (value1 + value2)
End Function
Public Function Subtract(ByVal value1 As Integer, ByVal value2 As Integer) As Integer
Return (value1 - value2)
End Function
End Class
'MathOp2 class
Public Class MathOp2 : Inherits MathOp
Public Function Multiply(int1stNum As Integer, int2ndNum As Integer) As Integer
Return int1stNum * int2ndNum
End Function
Public Function Divide(int1stNum As Integer, int2ndNum As Integer) As Integer
If int2ndNum > 0 Then
Return int1stNum / int2ndNum
Else
Return -9999
End If
End Function
End Class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
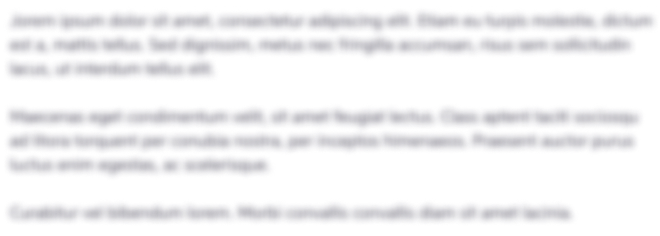
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started