Question
I have been struggling with the use of command line arguments in Java, any help would be appreciated, thank you! Modify the program to accept
I have been struggling with the use of command line arguments in Java, any help would be appreciated, thank you!
Modify the program to accept the values for the key as command line arguments, assuming the arguments are integers. A message should be printed if there are no arguments. If there is at least one argument, the average of all arguments should be computed and printed. You must use the parseInt method of the integer class to extract integer values from the strings that are passed in. Non-integer values passed in will inevitably produce an error, which is fine at this point.
Here is my code so far:
//Quizzes.java //Grades multiple choice quizzes
package quizzes;
//Import for use of the Scanner class import java.util.Scanner;
//Import for use of the Number Format class import java.text.NumberFormat;
public class Quizzes { //Reads in the number of questions followed by the key, then reads in //each student's answers and calculates the number and percent correct
public static void main(String[] args) { //Declaration of variables int numQuestions, answer; int numCorrect = 0; String anotherQuiz; NumberFormat percent = NumberFormat.getPercentInstance(); Scanner scan = new Scanner(System.in); System.out.println("Quiz grading"); System.out.println(); System.out.print("Enter the number of questions on the quiz: "); numQuestions = scan.nextInt(); System.out.println(); //Create the array for the key int[] key = new int[numQuestions]; //Load the array with input from the user System.out.println("Enter the answer key: "); String input = scan.nextLine(); // for(int index = 0; index < key.length; index++) // { // System.out.println("Enter the answer for question " // + (index + 1) + ": "); // key[index] = scan.nextInt(); // } System.out.println(); String response; //Outer loop to allow the user to enter grades fo do { System.out.println("Enter the student's answers: "); for (int index = 0; index < key.length; index++) { System.out.println("Enter the student's answer for question " + (index + 1) + ": "); answer = scan.nextInt(); if (key[index] == answer) { numCorrect++; } } System.out.println(); //Display the number of correct answers and percent System.out.println("Number of correct answers: " + numCorrect + "/" + numQuestions); System.out.println("Percentage earned: " + percent.format((double)numCorrect / (double)numQuestions)); System.out.println(); System.out.println("Grade another quiz? (y/n)"); response = scan.next(); System.out.println(); } while (response.equals("y") || response.equals("Y")); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
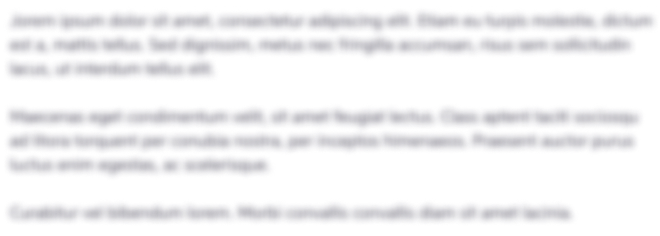
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started