Question
I have been trying to figure this assignment but I can't. can this be solved and explained? A2Driver.java import java.util.Arrays; public class A2Driver { public
I have been trying to figure this assignment but I can't. can this be solved and explained?
A2Driver.java
import java.util.Arrays;
public class A2Driver {
public static void main(String[] args) {
int N = 10;
System.out.println("N = "+N);
String[] ans = A2Work.sum0(N);
System.out.println("Sum0:");
System.out.println((ans[0].equals("f(N) = 5N+4"))?"f(N) Correct (0.5pts)":ans[0]+" : f(N) = 5N+4");
System.out.println((ans[1].equals("O(N) = N"))?"O(N) Correct (0.5pts)":ans[1]+" : O(N) = N");
long count = Long.parseLong(ans[2]);
long correct = 54;
double accuracy = Math.abs(correct-count)/(double)correct*100;//allow up to 5% off
System.out.println((accuracy <= 5)?"opCount Correct (0.5pts)":ans[2]+" : 504");
System.out.println();
System.out.println("Sum0:"+Arrays.toString(A2Work.sum0(N)));
System.out.println("Sum1:"+Arrays.toString(A2Work.sum1(N)));
System.out.println("Sum2:"+Arrays.toString(A2Work.sum2(N)));
System.out.println("Sum3:"+Arrays.toString(A2Work.sum3(N)));
System.out.println("Sum4:"+Arrays.toString(A2Work.sum4(N)));
System.out.println("Sum5:"+Arrays.toString(A2Work.sum5(N)));
System.out.println("Sum6:"+Arrays.toString(A2Work.sum6(N)));
System.out.println();
System.out.println("N = "+N);
System.out.println("Alg 1");
Object[] answer = A2Work.algorithm1(N);
System.out.println("Array Data Check:"+checkArray((int[])answer[0]));
System.out.println(Arrays.toString((String[])answer[1]));
System.out.println("Alg 2");
answer = A2Work.algorithm2(N);
System.out.println("Array Data Check:"+checkArray((int[])answer[0]));
System.out.println(Arrays.toString((String[])answer[1]));
System.out.println("Alg 3");
answer = A2Work.algorithm3(N);
System.out.println("Array Data Check:"+checkArray((int[])answer[0]));
System.out.println(Arrays.toString((String[])answer[1]));
System.out.println();
}
public static boolean checkArray(int[] arr)
{
Arrays.sort(arr);
int size = arr.length;
for(int i = 1; i < size; i++)
{
if(arr[i] == arr[i-1])//all values must be unique
{
return false;
}
if(arr[i] >= size)//no value larger than the size, use zero to N-1
{
return false;
}
}
return true;
}
}
A2Work.java
import java.util.Random;
public class A2Work {
/*
* NOTES:
* Include notes about f(N) for partial credit, similar to what I put in sum0()
* your f(N) does not need to produce the exact answer as your op count for all sums, but it should be close
* use estimates based on rules we discussed to determine f(N)
* For algorithm 1-3, assume random number generator never produces the same value twice in a given loop, but that it does produce the worst possible order of values
*
*/
/*
* Grading:
* THIS IS AN EXAMPLE
*/
public static String[] sum0(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = 5N+4";//sum assignment, loop, and return combined
String On = "O(N) = N";//Growth rate of function as value of N changes - Linear
//BEGIN opCounts
long sum = 0;
opCount++;//assignment of sum
opCount++;//assignment of i - happens as loop starts
opCount++;//comparison - happens as loop starts
for(int i = 0; i < N; i++)//5N+2 - +2 for components that happen as loop starts
{
//all work inside of loop is 5 units
//loop runs N times
//internal work of loop is 5N
sum++;
opCount+=2;//sum++ :: sum=sum+1 - addition and assignment
opCount+=2;//i++ :: i=i+1 - addition and assignment at end of each iteration of loop
opCount++;//comparison - happens at end of loop
}
opCount++;//return - don't need to count construction of return array for this assignment
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum1(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 0; i < N*N; i++)
{
sum++;
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum2(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N; j++)
{
sum++;
}
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum3(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N*N; j++)
{
sum++;
}
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum4(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 0; i < N; i++)
{
for(int j = 0; j < i; j++)
{
sum++;
}
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum5(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 0; i < N; i++)
{
for(int j = 0; j < i*i; j++)
{
for(int k = 0; k < j; k++)
{
sum++;
}
}
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static String[] sum6(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
long sum = 0;
for(int i = 1; i < N; i++)//i starts at 1 to prevent division error in if statement
{
for(int j = 0; j < i*i; j++)
{
if(j%i == 0)
{
for(int k = 0; k < j; k++)
{
sum++;
}
}
}
}
return new String[] {fn, On, opCount+""};
}
/*
* Grading:
* Correctly follow the described algorithm to complete the method - 1.5pt
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static Object[] algorithm1(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
int[] arr = new int[N];
Random randGen = new Random(123456789);
/*
* Use the following method to fill the array
* For each position in the array, generate a random number between zero and N
* - If N = 10, random numbers should be 0-9
* Check if that random number is used in any previous position in the array
* - If it is used anywhere else, generate a new number and try again
* - If it is not used anywhere else, place it into the position and move forward
*/
return new Object[] {arr, new String[] {fn, On, opCount+""}};
}
/*
* Grading:
* Correctly follow the described algorithm to complete the method - 1.5pt
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static Object[] algorithm2(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
int[] arr = new int[N];
boolean[] used = new boolean[N];
Random randGen = new Random(123456789);
/*
* Use the following method to fill the array
* For each position in the array, generate a random number between zero and N
* - If N = 10, random numbers should be 0-9
* Check if that used[random] is true
* - If it is, generate a new number and try again
* - If it is not, place it into the position, set used[random] = true, and move forward
*/
return new Object[] {arr, new String[] {fn, On, opCount+""}};
}
/*
* Grading:
* Correctly follow the described algorithm to complete the method - 1.5pt
* Add operation counts - 0.5pt
* f(N) formula (show your work) - 0.5pt
* O(N) reduction - 0.5pt
*/
public static Object[] algorithm3(int N)
{
//DO NOT COUNT IN opCount
long opCount = 0;
String fn = "f(N) = [answer]";
String On = "O(N) = [answer]";
//BEGIN opCounts
int[] arr = new int[N];
Random randGen = new Random(123456789);
/*
* Use the following method to fill the array
* Fill the arr with zero to N-1 in order
* Run a loop through each position
* - For each position, swap that position and a randomly chosen position
*/
return new Object[] {arr, new String[] {fn, On, opCount+""}};
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
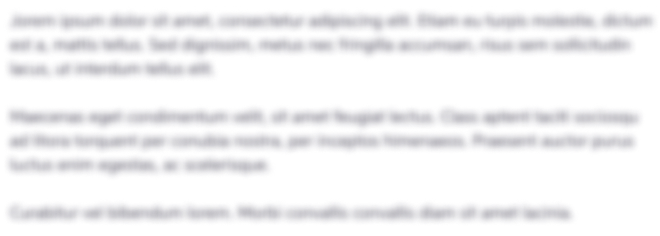
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started