Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I have created this code as a practice. This code works for control the flights in an airport, it has seven diferent classes. This is
I have created this code as a practice. This code works for control the flights in an airport, it has seven diferent classes. This is the copiable code //Tiempo.h #ifndef TIEMPO_H #define TIEMPO_H #pragma once #include using namespace std; //Clase Tiempo: Creada para determinar los tiempos de entrada y salida de los vuelos class Tiempo { public: Tiempo(); Tiempo(int hora, int minuto, int segundo); Tiempo(int hora, int minuto); Tiempo(int hora); string toString(); private: int hora; int minuto; int segundo; }; //Tiempo.cpp #endif // TIEMPO_H #include "Tiempo.h" #pragma once #include //Cdigo constructor de Tiempo using namespace std; Tiempo::Tiempo() { hora = minuto = segundo = 0; } Tiempo::Tiempo(int hora, int minuto, int segundo) { this->hora = hora; this->minuto = minuto; this->segundo = segundo; } Tiempo::Tiempo(int hora, int minuto) { this->hora = hora; this->minuto = minuto; this->segundo = 0; } Tiempo::Tiempo(int hora) { this->hora = hora; this->minuto = 0; this->segundo = 0; } string Tiempo::toString() { return to_string(hora) + ":" + to_string(minuto) + ":" + to_string(segundo); } //Pasajeros.h #ifndef PASAJEROS_H #define PASAJEROS_H #pragma once #include /* Clase Pasajeros que contiene atributos de codigo, nombre, precio del tickete, total de impuestos ademas de las funciones que calculan el pago total. */ using namespace std; class Pasajeros { public: Pasajeros(); Pasajeros(string codigo, string nombre, double precio_ticket, double imp); double getPago_total(); void setPago_total(double pay); void print(); private: string codigo; string nombre; double precio_ticket; double imp; double pago_total; }; #endif // PASAJEROS_H //Pasajeros.cpp #include "Pasajeros.h" #pragma once #include #include //Codigo constructor de la clase Pasajeros using namespace std; Pasajeros::Pasajeros() { } Pasajeros::Pasajeros(string codigo, string nombre, double precio_ticket, double imp) { this->codigo = codigo; this->nombre = nombre; this->precio_ticket = precio_ticket; this->imp = imp; pago_total = precio_ticket + imp * precio_ticket; } double Pasajeros::getPago_total() { return pago_total; } void Pasajeros::setPago_total(double pay) { this->pago_total = pay; } void Pasajeros::print() { cout cout cout cout cout } //Vuelo.h #ifndef VUELO_H #define VUELO_H #pragma once #include "Tiempo.h" using namespace std; //Clase Vuelo: esta contiene los atributos del nmero de vuelo, hora de salida y llegada de cada vuelo // Esta tambin trabaja en conjunto con la clase tiempo para establecer los tiempos class Vuelo { public: Vuelo(string num_vuelo); Vuelo(string num_vuelo, Tiempo hora_llegada, Tiempo hora_salida); string getnum_vuelo(); Tiempo gethora_salida(); Tiempo gethora_llegada(); void print(); private: string num_vuelo; Tiempo hora_salida; Tiempo hora_llegada; }; #endif // VUELO_H Vuelo.cpp #include "Vuelo.h" #include #include using namespace std; //Cdigo constructor de vuelo, este utiliza las funciones get para asi devolver correctamente lo solicitado. Vuelo::Vuelo(string num_vuelo) { this->num_vuelo = num_vuelo; } Vuelo::Vuelo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada) { this->num_vuelo = num_vuelo; this->hora_salida = hora_salida; this->hora_llegada = hora_llegada; } string Vuelo::getnum_vuelo() { return num_vuelo; } Tiempo Vuelo::gethora_salida() { return hora_salida; } Tiempo Vuelo::gethora_llegada() { return hora_llegada; } void Vuelo::print() { cout cout cout } //VueloLocal.h #ifndef VUELOLOCAL_H #define VUELOLOCAL_H #pragma once #include "Vuelo.h" #include "PasajerosFrecuentes.h" /* Clase Vuelo Local, trabaja en conjunto con la clase Vuelo con todos sus atributos adems de los nuevos; categoria, minimo de pasajeros */ class VueloLocal :public Vuelo { public: VueloLocal(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, int min_pasajeros, string categoria); VueloLocal(string num_vuelo, int min_pasajeros, string categoria); int getMin_Pasajeros(); string getCategoria(); void addPasajero(PasajerosFrecuentes pasajeros_frecuentes); void printPasajeros(); void print(); private: int min_pasajeros; string categoria;//dosmetica, comercial PasajerosFrecuentes arr[200]; int totalPasajeros; }; #endif // VUELOLOCAL_H //VueloLocal.cpp #include "VueloLocal.h" #pragma once #include #include "VueloLocal.h" //Cdigo constructor de Vuelo Local using namespace std; VueloLocal::VueloLocal(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, int min_pasajeros, string categoria) :Vuelo(num_vuelo, hora_salida, hora_llegada) { this->min_pasajeros = min_pasajeros; this->categoria = categoria; totalPasajeros = 0; } VueloLocal::VueloLocal(string num_vuelo, int min_pasajeros, string categoria) :Vuelo(num_vuelo) { this->min_pasajeros = min_pasajeros; this->categoria = categoria; totalPasajeros = 0; } int VueloLocal::getMin_Pasajeros() { return min_pasajeros; } string VueloLocal::getCategoria() { return categoria; } void VueloLocal::addPasajero(PasajerosFrecuentes frecuentespasajeros) { arr[totalPasajeros++] = frecuentespasajeros; } void VueloLocal::printPasajeros() { cout for(int i = 0; i { arr[i].print(); cout } } void VueloLocal::print() { Vuelo::print(); cout cout cout } //VueloInternacional.h #ifndef VUELOINTERNACIONAL_H #define VUELOINTERNACIONAL_H #pragma once #include #include "Vuelo.h" #include "Pasajeros.h" using namespace std; /* Clase Vuelo Internacional que trabaja en conjunto con la clase vuelo contiene sus atributos ademas del atributo de pais de destino. */ class VueloInternacional :protected Vuelo { public: VueloInternacional(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, string pais_destino); VueloInternacional(string num_vuelo, string pais_destino); string getPaisDestino(); void printPasajeros(); void addPasajeros(Pasajeros frecuentesPasajeros); void print(); private: string pais_destino; Pasajeros arr[200]; int totalPasajeros; }; #endif // VUELOINTERNACIONAL_H //VueloInternacional.cpp #include "VueloInternacional.h" #pragma once #include #include #include "VueloInternacional.h" //Codigo constructor de la Clase Vuelo Internacional. using namespace std; VueloInternacional::VueloInternacional(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, string pais_destino) :Vuelo(num_vuelo, hora_salida, hora_llegada) { this->pais_destino = pais_destino; totalPasajeros = 0; } VueloInternacional::VueloInternacional(string num_vuelo, string pais_destino) :Vuelo(num_vuelo) { this->pais_destino = pais_destino; totalPasajeros = 0; } string VueloInternacional::getPaisDestino() { return pais_destino; } void VueloInternacional::printPasajeros() { cout for (int i = 0; i { arr[i].print(); cout } } void VueloInternacional::addPasajeros(Pasajeros frecuentesPasajeros) { arr[totalPasajeros++] = frecuentesPasajeros; } void VueloInternacional::print() { Vuelo::print(); cout cout } //VueloCargo.h #ifndef VUELOCARGO_H #define VUELOCARGO_H #pragma once #include "Vuelo.h" /* Clase Vuelo de Cargo, trabaja en conjunto con la clase Vuelo, contiene todos sus atributos ademas del atributo peso maximo y el de destino de cliente. */ class VueloCargo :protected Vuelo { public: VueloCargo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, double peso_max, string destino_cliente); VueloCargo(string num_vuelo, double peso_max, string destino_cliente); double getPesoMax(); string getDestinoCliente(); private: double peso_max; string destino_cliente; }; #endif // VUELOCARGO_H VueloCargo.cpp #include "VueloCargo.h" #pragma once //Codigo constructor de la clase de Vuelo Cargo VueloCargo::VueloCargo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, double peso_max, string destino_cliente) :Vuelo(num_vuelo, hora_salida, hora_llegada) { this->peso_max = peso_max; this->destino_cliente = destino_cliente; } VueloCargo::VueloCargo(string num_vuelo, double peso_max, string destino_cliente) :Vuelo(num_vuelo) { this->destino_cliente = destino_cliente; } double VueloCargo::getPesoMax() { return peso_max; } string VueloCargo::getDestinoCliente() { return destino_cliente; } //PasajerosFrecuentes.h #ifndef PASAJEROSFRECUENTES_H #define PASAJEROSFRECUENTES_H #pragma once #include "Pasajeros.h" /* Clase de Pasajeros Frecuentes que trabaja en conjunto con la clase Pasajeros, esta contiene sus atributos ademas de un atributo descuento. */ class PasajerosFrecuentes :public Pasajeros { public: PasajerosFrecuentes(); PasajerosFrecuentes(string codigo, string nombre, double precio_ticket, double imp); private: double discount; }; #endif // PASAJEROSFRECUENTES_H //PasajerosFrecuentes.cpp #pragma once #include "PasajerosFrecuentes.h" //Codigo constructor de la clase Pasajeros Frecuentes, con la funcin set calcula el descuento que aplica. PasajerosFrecuentes::PasajerosFrecuentes() { } PasajerosFrecuentes::PasajerosFrecuentes(string codigo, string nombre, double precio_ticket, double imp): Pasajeros(codigo, nombre, precio_ticket, imp) { discount = 0.20; setPago_total(getPago_total()- getPago_total() * discount); } //main.cpp #include #include "VueloLocal.h" #include "VueloInternacional.h" /* Codigo principal */ using namespace std; int main() { Tiempo t11(12, 30); Tiempo t12(13, 30); Tiempo t21(9, 00); Tiempo t22(11, 30); VueloLocal vuelolocal("A109", t11, t12, 70, "domestico"); VueloInternacional vuelointernacional("B637", t21, t22, "UK"); Pasajeros p1("CD897", "Andrey Porras", 450, 3); Pasajeros p2("FG736", "Kendra Solis", 450, 3); PasajerosFrecuentes fp1("DE721", "Marco Ramirez", 670, 4.3); PasajerosFrecuentes fp2("JU981", "Oscar Fallas", 670, 4.3); vuelolocal.addPasajero(fp1); vuelolocal.addPasajero(fp2); vuelointernacional.addPasajeros(p1); vuelointernacional.addPasajeros(p2); cout vuelolocal.print(); cout vuelolocal.printPasajeros(); cout vuelointernacional.print(); cout vuelointernacional.printPasajeros(); return 0; } And this is an output
Now I need to modify this code with the following instructions: Using "friend" functions add two new classes; "UpdateVuelos" that is through class inheritance and that allows adding istream ostream for the entry and visualization of new flights using the cout command, in the same way add the class "UpdatePasajeros" that will work through inheritance with the Passengers class to add new passengers to the flights and that can be viewed as in the original code. Modify main () so that the data entry and update of the new passengers is from the keyboard and towards the console, the cin command can be used to enter the data. Please someone help me with that, it is important!!!!!! Copiable Code in english language: //==Time.h================= #pragma once #include using namespace std; class Time{ private: int hour; int minute; int second; public: Time(); Time(int hour, int minute, int second); Time(int hour, int minute); Time(int hour); string toString(); }; //==end of Time.h //==Time.cpp================= #pragma once #include "Time.h" #include using namespace std; Time::Time() { hour = minute = second = 0; } Time::Time(int hour, int minute, int second) { this->hour = hour; this->minute = minute; this->second = second; } Time::Time(int hour, int minute) { this->hour = hour; this->minute = minute; this->second = 0; } Time::Time(int hour) { this->hour = hour; this->minute = 0; this->second = 0; } string Time::toString() { return to_string(hour) + ":" + to_string(minute) + ":" + to_string(second); } //==end of Time.cpp //==Passenger.h================== #pragma once #include using namespace std; class Passenger{ private: string code; string name; double ticket_price; double tax_percentage; double total_payable; public: Passenger(); Passenger(string code, string name, double ticket_price, double tax_percentage); double getTotalPayable(); void setTotalPayable(double payable); void print(); }; //==end of Passenger.h============= //==Passenger.cpp================== #pragma once #include #include #include "Passenger.h" using namespace std; Passenger::Passenger() { } Passenger::Passenger(string code, string name, double ticket_price, double tax_percentage) { this->code = code; this->name = name; this->ticket_price = ticket_price; this->tax_percentage = tax_percentage; total_payable = ticket_price + tax_percentage * ticket_price; } double Passenger::getTotalPayable() { return total_payable; } void Passenger::setTotalPayable(double payable) { this->total_payable = payable; } void Passenger::print() { cout cout cout cout cout } //==end of Passenger.cpp============= //==Flight.h====================== #pragma once #include #include "Time.h" using namespace std; class Flight{ private: string flight_number; Time departure_time; Time arrival_time; public: Flight(string flight_number); Flight(string flight_number, Time departure_time, Time arrival_time); string getFlightNumber(); Time getDepartureTime(); Time getArrivalTime(); void print(); }; //===end of Flight.h============== //==Flight.cpp======================== #include #include #include "Flight.h" using namespace std; Flight::Flight(string flight_number) { this->flight_number = flight_number; } Flight::Flight(string flight_number, Time departure_time, Time arrival_time) { this->flight_number = flight_number; this->departure_time = departure_time; this->arrival_time = arrival_time; } string Flight::getFlightNumber() { return flight_number; } Time Flight::getDepartureTime() { return departure_time; } Time Flight::getArrivalTime() { return arrival_time; } void Flight::print() { cout cout cout } //==end of Flight.cpp=============== //CargoFlight.h===================== #pragma once #include "Flight.h" class CargoFlight :protected Flight{ private: double maximum_weight; string destination_client; public: CargoFlight(string flight_number, Time departure_time, Time arrival_time, double maximum_weight, string destination_client); CargoFlight(string flight_number, double maximum_weight, string destination_client); double getMaximumWeight(); string getDestinationClient(); }; //==end of CargoFlight.h============ //CargoFlight.cpp===================== #pragma once #include "CargoFlight.h" CargoFlight::CargoFlight(string flight_number, Time departure_time, Time arrival_time, double maximum_weight, string destination_client) :Flight(flight_number, departure_time, arrival_time) { this->maximum_weight = maximum_weight; this->destination_client = destination_client; } CargoFlight::CargoFlight(string flight_number, double maximum_weight, string destination_client) :Flight(flight_number) { this->destination_client = destination_client; } double CargoFlight::getMaximumWeight() { return maximum_weight; } string CargoFlight::getDestinationClient() { return destination_client; } //==end of CargoFlight.cpp============ //===LocalFlight.h====================== #pragma once #include "Flight.h" #include "FrequentPassengers.h" class LocalFlight :public Flight { private: int minimum_no_of_passengers; string category;//domestic, commercial FrequentPassengers arr[200]; int totalPassenger; public: LocalFlight(string flight_number, Time departure_time, Time arrival_time, int minimum_no_of_passenger, string category); LocalFlight(string flight_number, int minimum_no_of_passenger, string category); int getMinimumNoOfPassengers(); string getCategory(); void addPassenger(FrequentPassengers frequentPassenger); void printPassenger(); void print(); }; //==end of LocalFlight.h============== //===LocalFlight.cpp====================== #pragma once #include #include "LocalFlight.h" using namespace std; LocalFlight::LocalFlight(string flight_number, Time departure_time, Time arrival_time, int minimum_no_of_passenger, string category) :Flight(flight_number, departure_time, arrival_time) { this->minimum_no_of_passengers = minimum_no_of_passenger; this->category = category; totalPassenger = 0; } LocalFlight::LocalFlight(string flight_number, int minimum_no_of_passenger, string category) :Flight(flight_number) { this->minimum_no_of_passengers = minimum_no_of_passenger; this->category = category; totalPassenger = 0; } int LocalFlight::getMinimumNoOfPassengers() { return minimum_no_of_passengers; } string LocalFlight::getCategory() { return category; } void LocalFlight::addPassenger(FrequentPassengers frequentPassenger) { arr[totalPassenger++] = frequentPassenger; } void LocalFlight::printPassenger() { cout for (int i = 0; i arr[i].print(); cout } } void LocalFlight::print() { Flight::print(); cout cout cout } //==end of LocalFlight.cpp============== //==InternationalFlight.h==================== #pragma once #include #include "Flight.h" #include "Passenger.h" using namespace std; class InternationalFlight :public Flight{ private: string destination_country; Passenger arr[200]; int totalPassenger; public: InternationalFlight(string flight_number, Time departure_time, Time arrival_time, string destination_country); InternationalFlight(string flight_number, string destination_country); string getDestinationCountry(); void printPassenger(); void addPassenger(Passenger frequentPassenger); void print(); }; //==end of InternationalFlight.h============= //==InternationalFlight.cpp==================== #pragma once #include #include #include "InternationalFlight.h" using namespace std; InternationalFlight::InternationalFlight(string flight_number, Time departure_time, Time arrival_time, string destination_country) :Flight(flight_number, departure_time, arrival_time) { this->destination_country = destination_country; totalPassenger = 0; } InternationalFlight::InternationalFlight(string flight_number, string destination_country) :Flight(flight_number) { this->destination_country = destination_country; totalPassenger = 0; } string InternationalFlight::getDestinationCountry() { return destination_country; } void InternationalFlight::printPassenger() { cout for (int i = 0; i arr[i].print(); cout } } void InternationalFlight::addPassenger(Passenger frequentPassenger) { arr[totalPassenger++] = frequentPassenger; } void InternationalFlight::print() { Flight::print(); cout cout } //==end of InternationalFlight.cpp============= //==FrequentPassengers.h================= #pragma once #include "Passenger.h" class FrequentPassengers :public Passenger{ private: double discount; public: FrequentPassengers(); FrequentPassengers(string code, string name, double ticket_price, double tax_percentage); }; //==end of FrequentPassengers.h============= //==FrequentPassengers.cpp================= #pragma once #include "FrequentPassengers.h" FrequentPassengers::FrequentPassengers() { } FrequentPassengers::FrequentPassengers(string code, string name, double ticket_price, double tax_percentage) : Passenger(code, name, ticket_price, tax_percentage) { discount = 0.20; setTotalPayable(getTotalPayable() - getTotalPayable() * discount); } //==end of FrequentPassengers.cpp============= // Main.cpp : //This file contains the 'main' function. Program execution begins and ends there. #include #include "LocalFlight.h" #include "InternationalFlight.h" using namespace std; int main(){ Time t11(12, 30); Time t12(13, 30); Time t21(9, 00); Time t22(11, 30); LocalFlight localflight("A109", t11, t12, 70, "domestic"); InternationalFlight internationalflight("B637", t21, t22, "UK"); Passenger p1("CD897", "Raman Sha", 450, 3); Passenger p2("FG736", "Jack Dawson", 450, 3); FrequentPassengers fp1("DE721", "Jenifer Ken", 670, 4.3); FrequentPassengers fp2("JU981", "David Dey", 670, 4.3); localflight.addPassenger(fp1); localflight.addPassenger(fp2); internationalflight.addPassenger(p1); internationalflight.addPassenger(p2); cout localflight.print(); cout localflight.printPassenger(); cout internationalflight.print(); cout internationalflight.printPassenger(); return 0; Codigo: DE721 Nombre: Marco Ramirez Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Codigo: JU981 Nombre: Oscar Fallas Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Vuelo Internacional Detalles: Numero de Vuelo: B637 Hora de salida: 9:0:0 Hora de llegada: 11:30:0 Pais destino: UK Total de Pasajeros: 2 Detalles de pasajeros: Codigo: CD897 Nombre: Andrey Porras Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: FG736 Nombre: Kendra Solis Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: DE721 Nombre: Marco Ramirez Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Codigo: JU981 Nombre: Oscar Fallas Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Vuelo Internacional Detalles: Numero de Vuelo: B637 Hora de salida: 9:0:0 Hora de llegada: 11:30:0 Pais destino: UK Total de Pasajeros: 2 Detalles de pasajeros: Codigo: CD897 Nombre: Andrey Porras Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: FG736 Nombre: Kendra Solis Precio ticket: 450 Total de impuestos: 3 Pago total: 1800
//Tiempo.h
#ifndef TIEMPO_H
#define TIEMPO_H
#pragma once
#include
using namespace std;
//Clase Tiempo: Creada para determinar los tiempos de entrada y salida de los vuelos
class Tiempo
{
public:
Tiempo();
Tiempo(int hora, int minuto, int segundo);
Tiempo(int hora, int minuto);
Tiempo(int hora);
string toString();
private:
int hora;
int minuto;
int segundo;
};
//Tiempo.cpp
#endif // TIEMPO_H
#include "Tiempo.h"
#pragma once
#include
//Cdigo constructor de Tiempo
using namespace std;
Tiempo::Tiempo()
{
hora = minuto = segundo = 0;
}
Tiempo::Tiempo(int hora, int minuto, int segundo)
{
this->hora = hora;
this->minuto = minuto;
this->segundo = segundo;
}
Tiempo::Tiempo(int hora, int minuto)
{
this->hora = hora;
this->minuto = minuto;
this->segundo = 0;
}
Tiempo::Tiempo(int hora)
{
this->hora = hora;
this->minuto = 0;
this->segundo = 0;
}
string Tiempo::toString()
{
return to_string(hora) + ":" + to_string(minuto) + ":" + to_string(segundo);
}
//Pasajeros.h
#ifndef PASAJEROS_H
#define PASAJEROS_H
#pragma once
#include
/*
Clase Pasajeros que contiene atributos de codigo, nombre, precio del tickete, total de impuestos
ademas de las funciones que calculan el pago total.
*/
using namespace std;
class Pasajeros
{
public:
Pasajeros();
Pasajeros(string codigo, string nombre, double precio_ticket, double imp);
double getPago_total();
void setPago_total(double pay);
void print();
private:
string codigo;
string nombre;
double precio_ticket;
double imp;
double pago_total;
};
#endif // PASAJEROS_H
//Pasajeros.cpp
#include "Pasajeros.h"
#pragma once
#include
#include
//Codigo constructor de la clase Pasajeros
using namespace std;
Pasajeros::Pasajeros()
{
}
Pasajeros::Pasajeros(string codigo, string nombre, double precio_ticket, double imp)
{
this->codigo = codigo;
this->nombre = nombre;
this->precio_ticket = precio_ticket;
this->imp = imp;
pago_total = precio_ticket + imp * precio_ticket;
}
double Pasajeros::getPago_total()
{
return pago_total;
}
void Pasajeros::setPago_total(double pay)
{
this->pago_total = pay;
}
void Pasajeros::print()
{
cout
cout
cout
cout
cout
}
//Vuelo.h
#ifndef VUELO_H
#define VUELO_H
#pragma once
#include "Tiempo.h"
using namespace std;
//Clase Vuelo: esta contiene los atributos del nmero de vuelo, hora de salida y llegada de cada vuelo
// Esta tambin trabaja en conjunto con la clase tiempo para establecer los tiempos
class Vuelo
{
public:
Vuelo(string num_vuelo);
Vuelo(string num_vuelo, Tiempo hora_llegada, Tiempo hora_salida);
string getnum_vuelo();
Tiempo gethora_salida();
Tiempo gethora_llegada();
void print();
private:
string num_vuelo;
Tiempo hora_salida;
Tiempo hora_llegada;
};
#endif // VUELO_H
Vuelo.cpp
#include "Vuelo.h"
#include
#include
using namespace std;
//Cdigo constructor de vuelo, este utiliza las funciones get para asi devolver correctamente lo solicitado.
Vuelo::Vuelo(string num_vuelo)
{
this->num_vuelo = num_vuelo;
}
Vuelo::Vuelo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada)
{
this->num_vuelo = num_vuelo;
this->hora_salida = hora_salida;
this->hora_llegada = hora_llegada;
}
string Vuelo::getnum_vuelo()
{
return num_vuelo;
}
Tiempo Vuelo::gethora_salida()
{
return hora_salida;
}
Tiempo Vuelo::gethora_llegada()
{
return hora_llegada;
}
void Vuelo::print()
{
cout
cout
cout
}
//VueloLocal.h
#ifndef VUELOLOCAL_H
#define VUELOLOCAL_H
#pragma once
#include "Vuelo.h"
#include "PasajerosFrecuentes.h"
/* Clase Vuelo Local, trabaja en conjunto con la clase Vuelo con todos sus atributos
adems de los nuevos; categoria, minimo de pasajeros
*/
class VueloLocal :public Vuelo
{
public:
VueloLocal(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, int min_pasajeros, string categoria);
VueloLocal(string num_vuelo, int min_pasajeros, string categoria);
int getMin_Pasajeros();
string getCategoria();
void addPasajero(PasajerosFrecuentes pasajeros_frecuentes);
void printPasajeros();
void print();
private:
int min_pasajeros;
string categoria;//dosmetica, comercial
PasajerosFrecuentes arr[200];
int totalPasajeros;
};
#endif // VUELOLOCAL_H
//VueloLocal.cpp
#include "VueloLocal.h"
#pragma once
#include
#include "VueloLocal.h"
//Cdigo constructor de Vuelo Local
using namespace std;
VueloLocal::VueloLocal(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, int min_pasajeros, string categoria)
:Vuelo(num_vuelo, hora_salida, hora_llegada)
{
this->min_pasajeros = min_pasajeros;
this->categoria = categoria;
totalPasajeros = 0;
}
VueloLocal::VueloLocal(string num_vuelo, int min_pasajeros, string categoria)
:Vuelo(num_vuelo)
{
this->min_pasajeros = min_pasajeros;
this->categoria = categoria;
totalPasajeros = 0;
}
int VueloLocal::getMin_Pasajeros()
{
return min_pasajeros;
}
string VueloLocal::getCategoria()
{
return categoria;
}
void VueloLocal::addPasajero(PasajerosFrecuentes frecuentespasajeros)
{
arr[totalPasajeros++] = frecuentespasajeros;
}
void VueloLocal::printPasajeros()
{
cout
for(int i = 0; i
{
arr[i].print();
cout
}
}
void VueloLocal::print()
{
Vuelo::print();
cout
cout
cout
}
//VueloInternacional.h
#ifndef VUELOINTERNACIONAL_H
#define VUELOINTERNACIONAL_H
#pragma once
#include
#include "Vuelo.h"
#include "Pasajeros.h"
using namespace std;
/*
Clase Vuelo Internacional que trabaja en conjunto con la clase vuelo
contiene sus atributos ademas del atributo de pais de destino.
*/
class VueloInternacional :protected Vuelo
{
public:
VueloInternacional(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, string pais_destino);
VueloInternacional(string num_vuelo, string pais_destino);
string getPaisDestino();
void printPasajeros();
void addPasajeros(Pasajeros frecuentesPasajeros);
void print();
private:
string pais_destino;
Pasajeros arr[200];
int totalPasajeros;
};
#endif // VUELOINTERNACIONAL_H
//VueloInternacional.cpp
#include "VueloInternacional.h"
#pragma once
#include
#include
#include "VueloInternacional.h"
//Codigo constructor de la Clase Vuelo Internacional.
using namespace std;
VueloInternacional::VueloInternacional(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, string pais_destino)
:Vuelo(num_vuelo, hora_salida, hora_llegada)
{
this->pais_destino = pais_destino;
totalPasajeros = 0;
}
VueloInternacional::VueloInternacional(string num_vuelo, string pais_destino)
:Vuelo(num_vuelo)
{
this->pais_destino = pais_destino;
totalPasajeros = 0;
}
string VueloInternacional::getPaisDestino()
{
return pais_destino;
}
void VueloInternacional::printPasajeros()
{
cout
for (int i = 0; i
{
arr[i].print();
cout
}
}
void VueloInternacional::addPasajeros(Pasajeros frecuentesPasajeros)
{
arr[totalPasajeros++] = frecuentesPasajeros;
}
void VueloInternacional::print()
{
Vuelo::print();
cout
cout
}
//VueloCargo.h
#ifndef VUELOCARGO_H
#define VUELOCARGO_H
#pragma once
#include "Vuelo.h"
/*
Clase Vuelo de Cargo, trabaja en conjunto con la clase Vuelo, contiene todos sus atributos
ademas del atributo peso maximo y el de destino de cliente.
*/
class VueloCargo :protected Vuelo
{
public:
VueloCargo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, double peso_max, string destino_cliente);
VueloCargo(string num_vuelo, double peso_max, string destino_cliente);
double getPesoMax();
string getDestinoCliente();
private:
double peso_max;
string destino_cliente;
};
#endif // VUELOCARGO_H
VueloCargo.cpp
#include "VueloCargo.h"
#pragma once
//Codigo constructor de la clase de Vuelo Cargo
VueloCargo::VueloCargo(string num_vuelo, Tiempo hora_salida, Tiempo hora_llegada, double peso_max, string destino_cliente)
:Vuelo(num_vuelo, hora_salida, hora_llegada)
{
this->peso_max = peso_max;
this->destino_cliente = destino_cliente;
}
VueloCargo::VueloCargo(string num_vuelo, double peso_max, string destino_cliente)
:Vuelo(num_vuelo)
{
this->destino_cliente = destino_cliente;
}
double VueloCargo::getPesoMax()
{
return peso_max;
}
string VueloCargo::getDestinoCliente()
{
return destino_cliente;
}
//PasajerosFrecuentes.h
#ifndef PASAJEROSFRECUENTES_H
#define PASAJEROSFRECUENTES_H
#pragma once
#include "Pasajeros.h"
/*
Clase de Pasajeros Frecuentes que trabaja en conjunto con la clase Pasajeros, esta contiene sus atributos
ademas de un atributo descuento.
*/
class PasajerosFrecuentes :public Pasajeros
{
public:
PasajerosFrecuentes();
PasajerosFrecuentes(string codigo, string nombre, double precio_ticket, double imp);
private:
double discount;
};
#endif // PASAJEROSFRECUENTES_H
//PasajerosFrecuentes.cpp
#pragma once
#include "PasajerosFrecuentes.h"
//Codigo constructor de la clase Pasajeros Frecuentes, con la funcin set calcula el descuento que aplica.
PasajerosFrecuentes::PasajerosFrecuentes()
{
}
PasajerosFrecuentes::PasajerosFrecuentes(string codigo, string nombre, double precio_ticket, double imp):
Pasajeros(codigo, nombre, precio_ticket, imp)
{
discount = 0.20;
setPago_total(getPago_total()- getPago_total() * discount);
}
//main.cpp
#include
#include "VueloLocal.h"
#include "VueloInternacional.h"
/*
Codigo principal
*/
using namespace std;
int main()
{
Tiempo t11(12, 30);
Tiempo t12(13, 30);
Tiempo t21(9, 00);
Tiempo t22(11, 30);
VueloLocal vuelolocal("A109", t11, t12, 70, "domestico");
VueloInternacional vuelointernacional("B637", t21, t22, "UK");
Pasajeros p1("CD897", "Andrey Porras", 450, 3);
Pasajeros p2("FG736", "Kendra Solis", 450, 3);
PasajerosFrecuentes fp1("DE721", "Marco Ramirez", 670, 4.3);
PasajerosFrecuentes fp2("JU981", "Oscar Fallas", 670, 4.3);
vuelolocal.addPasajero(fp1);
vuelolocal.addPasajero(fp2);
vuelointernacional.addPasajeros(p1);
vuelointernacional.addPasajeros(p2);
cout
vuelolocal.print();
cout
vuelolocal.printPasajeros();
cout
vuelointernacional.print();
cout
vuelointernacional.printPasajeros();
return 0;
}
And this is an output
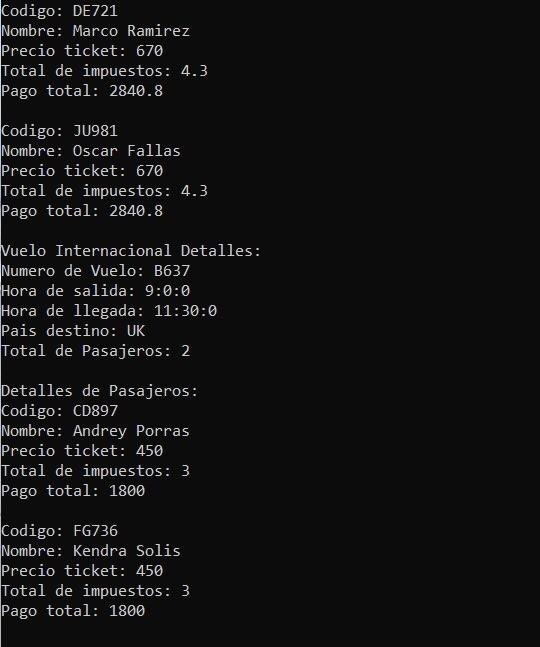
Now I need to modify this code with the following instructions:
Using "friend" functions add two new classes; "UpdateVuelos" that is through class inheritance and that allows adding istream ostream for the entry and visualization of new flights using the cout command, in the same way add the class "UpdatePasajeros" that will work through inheritance with the Passengers class to add new passengers to the flights and that can be viewed as in the original code. Modify main () so that the data entry and update of the new passengers is from the keyboard and towards the console, the cin command can be used to enter the data.
Please someone help me with that, it is important!!!!!!
Copiable Code in english language:
//==Time.h=================
#pragma once
#include
using namespace std;
class Time{
private:
int hour;
int minute;
int second;
public:
Time();
Time(int hour, int minute, int second);
Time(int hour, int minute);
Time(int hour);
string toString();
};
//==end of Time.h
//==Time.cpp=================
#pragma once
#include "Time.h"
#include
using namespace std;
Time::Time() {
hour = minute = second = 0;
}
Time::Time(int hour, int minute, int second) {
this->hour = hour;
this->minute = minute;
this->second = second;
}
Time::Time(int hour, int minute) {
this->hour = hour;
this->minute = minute;
this->second = 0;
}
Time::Time(int hour) {
this->hour = hour;
this->minute = 0;
this->second = 0;
}
string Time::toString() {
return to_string(hour) + ":" + to_string(minute) + ":" + to_string(second);
}
//==end of Time.cpp
//==Passenger.h==================
#pragma once
#include
using namespace std;
class Passenger{
private:
string code;
string name;
double ticket_price;
double tax_percentage;
double total_payable;
public:
Passenger();
Passenger(string code, string name, double ticket_price, double tax_percentage);
double getTotalPayable();
void setTotalPayable(double payable);
void print();
};
//==end of Passenger.h=============
//==Passenger.cpp==================
#pragma once
#include
#include
#include "Passenger.h"
using namespace std;
Passenger::Passenger() {
}
Passenger::Passenger(string code, string name, double ticket_price, double tax_percentage) {
this->code = code;
this->name = name;
this->ticket_price = ticket_price;
this->tax_percentage = tax_percentage;
total_payable = ticket_price + tax_percentage * ticket_price;
}
double Passenger::getTotalPayable() {
return total_payable;
}
void Passenger::setTotalPayable(double payable) {
this->total_payable = payable;
}
void Passenger::print() {
cout
cout
cout
cout
cout
}
//==end of Passenger.cpp=============
//==Flight.h======================
#pragma once
#include
#include "Time.h"
using namespace std;
class Flight{
private:
string flight_number;
Time departure_time;
Time arrival_time;
public:
Flight(string flight_number);
Flight(string flight_number, Time departure_time, Time arrival_time);
string getFlightNumber();
Time getDepartureTime();
Time getArrivalTime();
void print();
};
//===end of Flight.h==============
//==Flight.cpp========================
#include
#include
#include "Flight.h"
using namespace std;
Flight::Flight(string flight_number) {
this->flight_number = flight_number;
}
Flight::Flight(string flight_number, Time departure_time, Time arrival_time) {
this->flight_number = flight_number;
this->departure_time = departure_time;
this->arrival_time = arrival_time;
}
string Flight::getFlightNumber() {
return flight_number;
}
Time Flight::getDepartureTime() {
return departure_time;
}
Time Flight::getArrivalTime() {
return arrival_time;
}
void Flight::print() {
cout
cout
cout
}
//==end of Flight.cpp===============
//CargoFlight.h=====================
#pragma once
#include "Flight.h"
class CargoFlight :protected Flight{
private:
double maximum_weight;
string destination_client;
public:
CargoFlight(string flight_number, Time departure_time, Time arrival_time, double maximum_weight, string destination_client);
CargoFlight(string flight_number, double maximum_weight, string destination_client);
double getMaximumWeight();
string getDestinationClient();
};
//==end of CargoFlight.h============
//CargoFlight.cpp=====================
#pragma once
#include "CargoFlight.h"
CargoFlight::CargoFlight(string flight_number, Time departure_time, Time arrival_time, double maximum_weight, string destination_client)
:Flight(flight_number, departure_time, arrival_time)
{
this->maximum_weight = maximum_weight;
this->destination_client = destination_client;
}
CargoFlight::CargoFlight(string flight_number, double maximum_weight, string destination_client)
:Flight(flight_number)
{
this->destination_client = destination_client;
}
double CargoFlight::getMaximumWeight() {
return maximum_weight;
}
string CargoFlight::getDestinationClient() {
return destination_client;
}
//==end of CargoFlight.cpp============
//===LocalFlight.h======================
#pragma once
#include "Flight.h"
#include "FrequentPassengers.h"
class LocalFlight :public Flight {
private:
int minimum_no_of_passengers;
string category;//domestic, commercial
FrequentPassengers arr[200];
int totalPassenger;
public:
LocalFlight(string flight_number, Time departure_time, Time arrival_time, int minimum_no_of_passenger, string category);
LocalFlight(string flight_number, int minimum_no_of_passenger, string category);
int getMinimumNoOfPassengers();
string getCategory();
void addPassenger(FrequentPassengers frequentPassenger);
void printPassenger();
void print();
};
//==end of LocalFlight.h==============
//===LocalFlight.cpp======================
#pragma once
#include
#include "LocalFlight.h"
using namespace std;
LocalFlight::LocalFlight(string flight_number, Time departure_time, Time arrival_time, int minimum_no_of_passenger, string category)
:Flight(flight_number, departure_time, arrival_time)
{
this->minimum_no_of_passengers = minimum_no_of_passenger;
this->category = category;
totalPassenger = 0;
}
LocalFlight::LocalFlight(string flight_number, int minimum_no_of_passenger, string category)
:Flight(flight_number)
{
this->minimum_no_of_passengers = minimum_no_of_passenger;
this->category = category;
totalPassenger = 0;
}
int LocalFlight::getMinimumNoOfPassengers() {
return minimum_no_of_passengers;
}
string LocalFlight::getCategory() {
return category;
}
void LocalFlight::addPassenger(FrequentPassengers frequentPassenger) {
arr[totalPassenger++] = frequentPassenger;
}
void LocalFlight::printPassenger() {
cout
for (int i = 0; i
arr[i].print();
cout
}
}
void LocalFlight::print() {
Flight::print();
cout
cout
cout
}
//==end of LocalFlight.cpp==============
//==InternationalFlight.h====================
#pragma once
#include
#include "Flight.h"
#include "Passenger.h"
using namespace std;
class InternationalFlight :public Flight{
private:
string destination_country;
Passenger arr[200];
int totalPassenger;
public:
InternationalFlight(string flight_number, Time departure_time, Time arrival_time, string destination_country);
InternationalFlight(string flight_number, string destination_country);
string getDestinationCountry();
void printPassenger();
void addPassenger(Passenger frequentPassenger);
void print();
};
//==end of InternationalFlight.h=============
//==InternationalFlight.cpp====================
#pragma once
#include
#include
#include "InternationalFlight.h"
using namespace std;
InternationalFlight::InternationalFlight(string flight_number, Time departure_time, Time arrival_time, string destination_country)
:Flight(flight_number, departure_time, arrival_time)
{
this->destination_country = destination_country;
totalPassenger = 0;
}
InternationalFlight::InternationalFlight(string flight_number, string destination_country)
:Flight(flight_number)
{
this->destination_country = destination_country;
totalPassenger = 0;
}
string InternationalFlight::getDestinationCountry() {
return destination_country;
}
void InternationalFlight::printPassenger() {
cout
for (int i = 0; i
arr[i].print();
cout
}
}
void InternationalFlight::addPassenger(Passenger frequentPassenger) {
arr[totalPassenger++] = frequentPassenger;
}
void InternationalFlight::print() {
Flight::print();
cout
cout
}
//==end of InternationalFlight.cpp=============
//==FrequentPassengers.h=================
#pragma once
#include "Passenger.h"
class FrequentPassengers :public Passenger{
private:
double discount;
public:
FrequentPassengers();
FrequentPassengers(string code, string name, double ticket_price, double tax_percentage);
};
//==end of FrequentPassengers.h=============
//==FrequentPassengers.cpp=================
#pragma once
#include "FrequentPassengers.h"
FrequentPassengers::FrequentPassengers() {
}
FrequentPassengers::FrequentPassengers(string code, string name, double ticket_price, double tax_percentage) :
Passenger(code, name, ticket_price, tax_percentage)
{
discount = 0.20;
setTotalPayable(getTotalPayable() - getTotalPayable() * discount);
}
//==end of FrequentPassengers.cpp=============
// Main.cpp :
//This file contains the 'main' function. Program execution begins and ends there.
#include
#include "LocalFlight.h"
#include "InternationalFlight.h"
using namespace std;
int main(){
Time t11(12, 30);
Time t12(13, 30);
Time t21(9, 00);
Time t22(11, 30);
LocalFlight localflight("A109", t11, t12, 70, "domestic");
InternationalFlight internationalflight("B637", t21, t22, "UK");
Passenger p1("CD897", "Raman Sha", 450, 3);
Passenger p2("FG736", "Jack Dawson", 450, 3);
FrequentPassengers fp1("DE721", "Jenifer Ken", 670, 4.3);
FrequentPassengers fp2("JU981", "David Dey", 670, 4.3);
localflight.addPassenger(fp1);
localflight.addPassenger(fp2);
internationalflight.addPassenger(p1);
internationalflight.addPassenger(p2);
cout
localflight.print();
cout
localflight.printPassenger();
cout
internationalflight.print();
cout
Codigo: DE721 Nombre: Marco Ramirez Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Codigo: JU981 Nombre: Oscar Fallas Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Vuelo Internacional Detalles: Numero de Vuelo: B637 Hora de salida: 9:0:0 Hora de llegada: 11:30:0 Pais destino: UK Total de Pasajeros: 2 Detalles de pasajeros: Codigo: CD897 Nombre: Andrey Porras Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: FG736 Nombre: Kendra Solis Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: DE721 Nombre: Marco Ramirez Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Codigo: JU981 Nombre: Oscar Fallas Precio ticket: 670 Total de impuestos: 4.3 Pago total: 2840.8 Vuelo Internacional Detalles: Numero de Vuelo: B637 Hora de salida: 9:0:0 Hora de llegada: 11:30:0 Pais destino: UK Total de Pasajeros: 2 Detalles de pasajeros: Codigo: CD897 Nombre: Andrey Porras Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 Codigo: FG736 Nombre: Kendra Solis Precio ticket: 450 Total de impuestos: 3 Pago total: 1800 internationalflight.printPassenger();
return 0;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
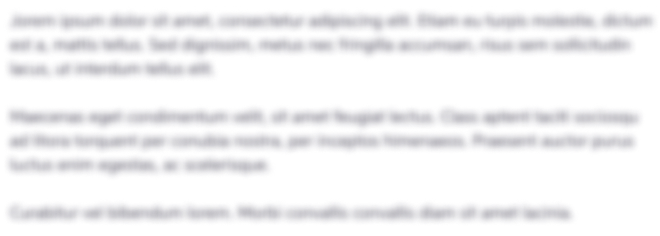
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started