Question
I have created this shape program in Java, I want it to be modified to these requirements: Part I: Create a program that stores objects
I have created this shape program in Java, I want it to be modified to these requirements: Part I: Create a program that stores objects of the RightTriangle, Rectangle, and Circle class in a Shape array. Use polymorphism or method overriding to print a description of each object. Part II: Same as above, but use an Object array. Go ahead and put both the above in the same main method. Thank you.
package main;
public class main {
public static void main(String[] args) { Shape s[] = new Shape[3]; s[0] = new Circle(10); s[1] = new RightTriangle(10, 10); s[2] = new Rectangle(10, 10); for(int i= 0; i < s.length; i++) System.out.println(s[i].toString()); }
}
public class Circle extends Shape {
private double radius = 10; private double PI = 3.14;
Circle(double r) { super("Circle"); radius = r; }
public double getArea() { return PI * radius * radius; } }
public class Rectangle extends Shape{ private double length = 10; private double width = 10; Rectangle(double l, double w) { super("Rectangle"); length = l; width = w; } public double getArea() { return length * width; } }
public class RightTriangle extends Shape {
private double base = 10; private double heigth = 10;
RightTriangle(double b, double h) { super("RightTriangle"); base = b; heigth = h; }
public double getArea() { return (base * heigth) / 2; } }
public class Shape {
private String type; private double area;
public Shape(String t) { type = t; area = getArea(); }
public void setType(String t) { type = t; }
public void setArea(double a) { area = a; }
public String getDescription() { return type; }
public double getArea() { return area; }
@Override public String toString() { return " Shape: " + type + " Area: " + getArea(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
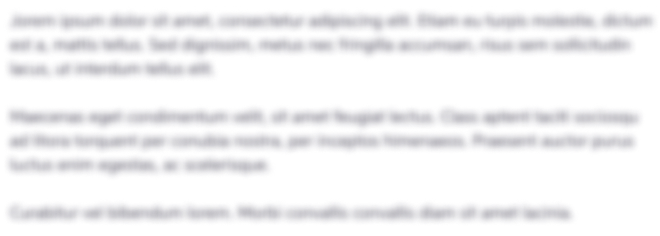
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started