Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
I have Implemented the algorithms for insertion sort, Merge Sort and Quicksort. I want to use find a timing system in JAVA like timing in
I have Implemented the algorithms for insertion sort, Merge Sort and Quicksort. I want to use find a timing system in JAVA like timing in C to compare the run time of the algorithms on inputs consisting of only positive integers. Please be able to input any size such as input sizes of The input can be generated randomly using the rand function in C but I dont know how in java. Please use a vector structure like in C STL to store the integers. Also I would like to be able to test the algorithms by using a small input size and print the list to make sure the algorithm sorts the list properly. Here is the code for the algorithms: import java.util.Arrays; public class SortingAlgorithms public static void mainString args int numbers ; System.out.println UNSORTED: Arrays.toStringnumbers; insertionSortnumbers; System.out.printlnInsertion sort SORTED: Arrays.toStringnumbers; mergeSortnumbers numbers.length ; System.out.println Merge sort SORTED: Arrays.toStringnumbers; quicksortnumbers numbers.length ; System.out.println Quick sort SORTED: Arrays.toStringnumbers; private static void insertionSortint numbers for int i ; i numbers.length; i int j i; while j && numbersj numbersj int temp numbersj; numbersj numbersj ; numbersj temp; j; private static void mergeint numbers, int i int j int k int mergedSize k i ; int mergedNumbers new intmergedSize; int mergePos ; int leftPos i; int rightPos j ; while leftPos j && rightPos k if numbersleftPos numbersrightPos mergedNumbersmergePos numbersleftPos; leftPos; else mergedNumbersmergePos numbersrightPos; rightPos; mergePos; while leftPos j mergedNumbersmergePos numbersleftPos; leftPos; mergePos; while rightPos k mergedNumbersmergePos numbersrightPos; rightPos; mergePos; for mergePos ; mergePos mergedSize; mergePos numbersi mergePos mergedNumbersmergePos; private static void mergeSortint numbers, int i int k int j ; if i k j i k; mergeSortnumbers i j; mergeSortnumbers j k; mergenumbers i j k; private static int partitionint numbers, int startIndex, int endIndex int midpoint startIndex endIndex startIndex; int pivot numbersmidpoint; int low startIndex; int high endIndex; boolean done false; while done while numberslow pivot low low ; while pivot numbershigh high high ; if low high done true; else int temp numberslow; numberslow numbershigh; numbershigh temp; low; high; return high; private static void quicksortint numbers, int startIndex, int endIndex Only attempt to sort the array segment if there are at least elements if endIndex startIndex return; Partition the array segment int high partitionnumbers startIndex, endIndex; Recursively sort the left segment quicksortnumbers startIndex, high; Recursively sort the right segment quicksortnumbers high endIndex;
I have Implemented the algorithms for insertion sort, Merge Sort and Quicksort. I want to use find a timing system in JAVA like timing in C to compare the run time of the algorithms on inputs consisting of only positive integers.
Please be able to input any size such as input sizes of
The input can be generated randomly using the rand function in C but I dont know how in java. Please use a vector structure like in C STL to store the integers.
Also I would like to be able to test the algorithms by using a small input size and print the list to make sure the algorithm sorts the list properly.
Here is the code for the algorithms:
import java.util.Arrays;
public class SortingAlgorithms
public static void mainString args
int numbers ;
System.out.println UNSORTED: Arrays.toStringnumbers;
insertionSortnumbers;
System.out.printlnInsertion sort SORTED: Arrays.toStringnumbers;
mergeSortnumbers numbers.length ;
System.out.println Merge sort SORTED: Arrays.toStringnumbers;
quicksortnumbers numbers.length ;
System.out.println Quick sort SORTED: Arrays.toStringnumbers;
private static void insertionSortint numbers
for int i ; i numbers.length; i
int j i;
while j && numbersj numbersj
int temp numbersj;
numbersj numbersj ;
numbersj temp;
j;
private static void mergeint numbers, int i int j int k
int mergedSize k i ;
int mergedNumbers new intmergedSize;
int mergePos ;
int leftPos i;
int rightPos j ;
while leftPos j && rightPos k
if numbersleftPos numbersrightPos
mergedNumbersmergePos numbersleftPos;
leftPos;
else
mergedNumbersmergePos numbersrightPos;
rightPos;
mergePos;
while leftPos j
mergedNumbersmergePos numbersleftPos;
leftPos;
mergePos;
while rightPos k
mergedNumbersmergePos numbersrightPos;
rightPos;
mergePos;
for mergePos ; mergePos mergedSize; mergePos
numbersi mergePos mergedNumbersmergePos;
private static void mergeSortint numbers, int i int k
int j ;
if i k
j i k;
mergeSortnumbers i j;
mergeSortnumbers j k;
mergenumbers i j k;
private static int partitionint numbers, int startIndex, int endIndex
int midpoint startIndex endIndex startIndex;
int pivot numbersmidpoint;
int low startIndex;
int high endIndex;
boolean done false;
while done
while numberslow pivot
low low ;
while pivot numbershigh
high high ;
if low high
done true;
else
int temp numberslow;
numberslow numbershigh;
numbershigh temp;
low;
high;
return high;
private static void quicksortint numbers, int startIndex, int endIndex
Only attempt to sort the array segment if there are
at least elements
if endIndex startIndex
return;
Partition the array segment
int high partitionnumbers startIndex, endIndex;
Recursively sort the left segment
quicksortnumbers startIndex, high;
Recursively sort the right segment
quicksortnumbers high endIndex;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
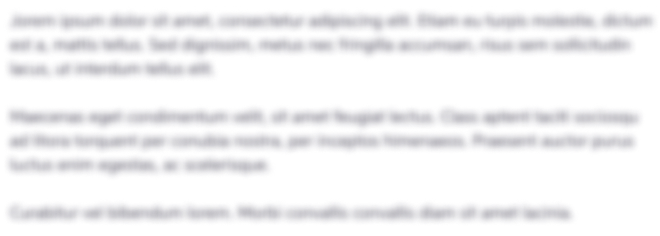
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started