I have provided all the information that was provided to me.
In C, instead of implementing the buffer as a circular queue, implement the buffer as a Dequeue (Doubly Ended Queue, i.e. insertion and deletion can be done at both end of the queue)
Will upvote if correct!
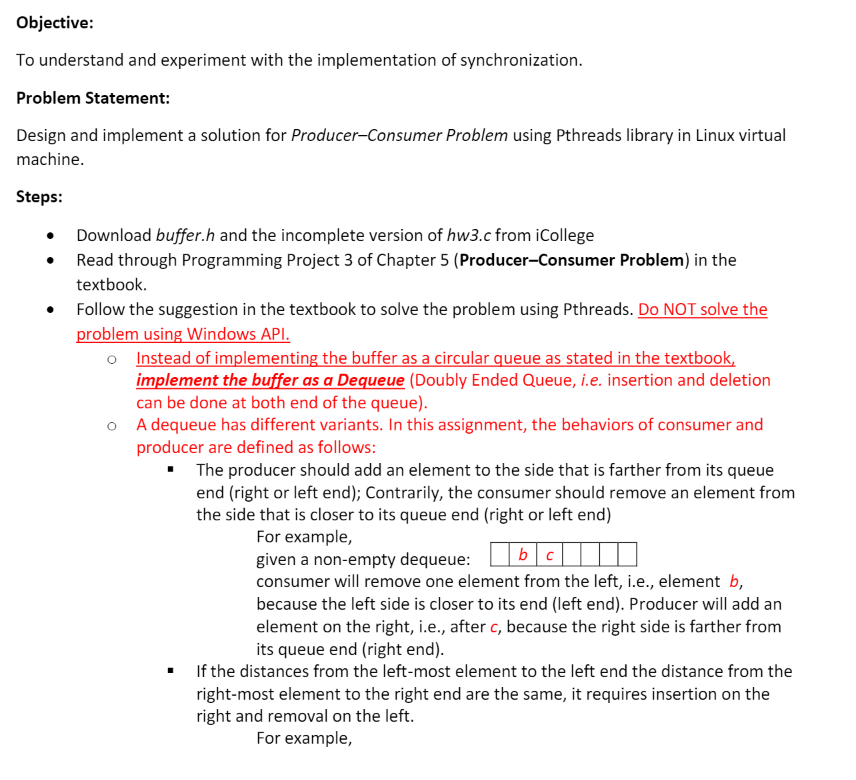
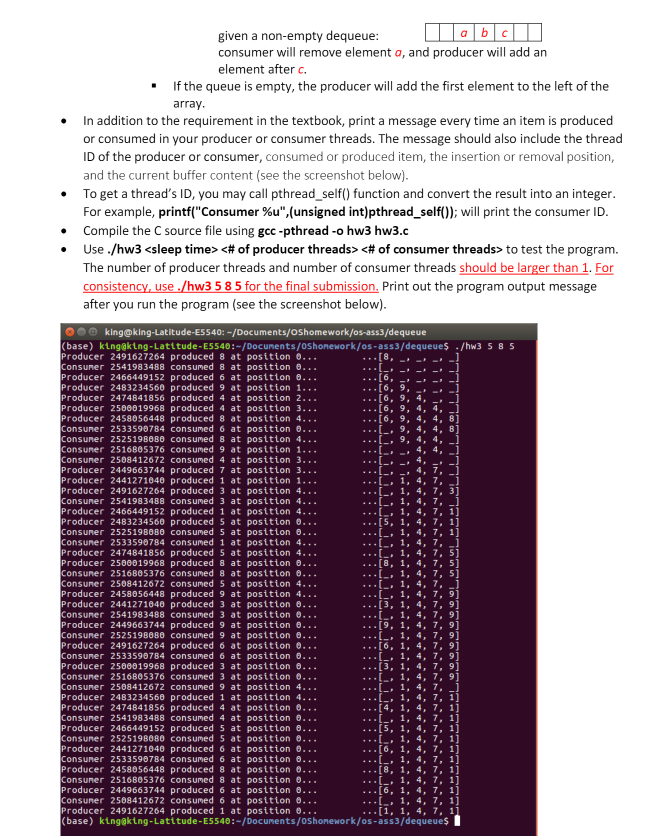
HW3.C FILE------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
#include "buffer.h" #include #include #include #include
buffer_item buffer[BUFFER_SIZE];
void *producer(void *param); void *consumer(void *param);
int insert_item(buffer_item item) { /* Acquire Empty Semaphore */ /* Acquire mutex lock to protect buffer */ /* Insert item into buffer */
/* Release mutex lock and full semaphore */
return 0; }
int remove_item(buffer_item *item) { /* Acquire Full Semaphore */
/* Acquire mutex lock to protect buffer */ /* remove an object from buffer placing it in item */
/* Release mutex lock and empty semaphore */ return 0; }
int main(int argc, char *argv[]) { /* Get command line arguments argv[1],argv[2],argv[3] */ /* Initialize buffer related synchronization tools */ /* Create producer threads based on the command line input */ /* Create consumer threads based on the command line input */ /* Sleep for user specified time based on the command line input */ return 0; }
void *producer(void *param) { /* producer thread that calls insert_item() */
}
void *consumer(void *param) { /* consumer thread that calls remove_item() */
}
BUFFER.H FILE-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
/* buffer.h*/
typedef int buffer_item; #define BUFFER_SIZE 5
int insert_item(buffer_item item); int remove_item(buffer_item *item);
Objective: To understand and experiment with the implementation of synchronization. Problem Statement: Design and implement a solution for Producer-Consumer Problem using Pthreads library in Linux virtual machine. Steps: . Download buffer.h and the incomplete version of hw3.c from iCollege Read through Programming Project 3 of Chapter 5 (Producer-Consumer Problem) in the textbook. Follow the suggestion in the textbook to solve the problem using Pthreads. Do NOT solve the problem using Windows API. Instead of implementing the buffer as a circular queue as stated in the textbook, implement the buffer as a Dequeue (Doubly Ended Queue, i.e.insertion and deletion can be done at both end of the queue). o Adequeue has different variants. In this assignment, the behaviors of consumer and producer are defined as follows: The producer should add an element to the side that is farther from its queue end (right or left end); Contrarily, the consumer should remove an element from the side that is closer to its queue end (right or left end) For example, given a non-empty dequeue: bc consumer will remove one element from the left, i.e., element b, because the left side is closer to its end (left end). Producer will add an element on the right, i.e., after c, because the right side is farther from its queue end (right end). If the distances from the left-most element to the left end the distance from the right-most element to the right end are the same, it requires insertion on the right and removal on the left. For example, given a non-empty dequeue: abc consumer will remove element a, and producer will add an element after c. If the queue is empty, the producer will add the first element to the left of the array. In addition to the requirement in the textbook, print a message every time an item is produced or consumed in your producer or consumer threads. The message should also include the thread ID of the producer or consumer, consumed or produced item, the insertion or removal position, and the current buffer content (see the screenshot below). To get a thread's ID, you may call pthread_self() function and convert the result into an integer. For example, printf("Consumer %u",(unsigned int)pthread_self()); will print the consumer ID. Compile the source file using gcc-pthread -o hw3 hw3.c Use ./hw3
to test the program. The number of producer threads and number of consumer threads should be larger than 1. For consistency, use ./hw3 5 8 5 for the final submission. Print out the program output message after you run the program (see the screenshot below). O king@king-Latitude E5540:-/Documents/oshomework/os-ass3/dequeue (base) king@king-Latitude-E5540:-/Documents/oshonework/os-ass3/dequeue$ /hw3 5 8 5 Producer 2491627264 produced 8 at position 0... ...[8, Consumer 2541983488 consumed 8 at position 0... Producer 2466449152 produced 6 at position 0... [6, Producer 2483234560 produced 9 at position 1... 6, 9 Producer 2474841856 produced 4 at position 2... [6, 9, 4, _ Producer 2560019968 produced 4 at position 3... Producer 2458056448 produced 8 at position 4. [6, 9, 4, 4, Consumer 2533590784 consumed 6 at position o... 9, 4, 4. Consumer 2525198688 consumed 8 at position 4... Consumer 2516805376 consumed 9 at position 1... Consumer 2508412672 consumed 4 at position 3... Producer 2449663744 produced 7 at position 3... Producer 2441271040 produced i at position 1. 1, 4, 7, Producer 2491627264 produced 3 at position 4.. 1, 4, 7, 3) Consumer 2541983488 consumed 3 at position 4. L. 1, 4, 7, _) Producer 2466449152 produced i at position 4. -- 1, 4, 7, 1] Producer 2483234566 produced 5 at position 0.. (5, 1, 4, 7, 1] Consumer 2525198688 consumed 5 at position o.. 1, 4, 7, 1] Consumer 2533590784 consumed i at position 4.. 1, 4, 7, Producer 2474841856 produced 5 at position 4. . 1, 4, 7, 5] Producer 2506019968 produced 8 at position e. 8, 1, 4, 7, 5] Consumer 2516805376 consumed 8 at position 0. 1, 4, 7, 5] Consumer 2508412672 consumed 5 at position 4. - 1, 4, 7, _ Producer 2458856448 produced 9 at position 4.. 1, 4, 7, 9] Producer 2441271848 produced 3 at position 0.. (3, 1, 4, 7, 9j Consumer 2541983488 consumed 3 at position o... 1, 4, 7, 9] Producer 2449663744 produced 9 at position o... [9, 1, 4, 7, 9) Consumer 2525198680 consumed 9 at position 0... -. 1, 4, 7, 9] Producer 2491627264 produced 6 at position 0. [6, 1, 4, 7, 9) Consumer 2533590784 consumed 6 at position o.. 1, 4, 7, 9] Producer 2500019968 produced 3 at position 0.. (3, 1, 4, 7, 9] Consumer 2516805376 consumed 3 at position o... 1, 4, 7, 9] Consumer 2568412672 consumed 9 at position 4.. -- 1, 4, 7, _) Producer 2483234560 produced i at position 4... - 1, 4, 7, 1] Producer 2474841856 produced 4 at position o.. [4, 1, 4, 7, 1] Consumer 2541983488 consumed 4 at position 0. -- 1, 4, 7, 1] Producer 2466449152 produced 5 at position o.. [5, 1, 4, 7, 1] Consumer 2525198080 consumed 5 at position 0.. -, 1, 4, 7, 1] Producer 2441271840 produced 6 at position e... [6, 1, 4, 7, 1] Consumer 2533598784 consumed 6 at position ... 1, 4, 7, 1] Producer 2458056448 produced 8 at position 0... [8, 1, 4, 7, 1] Consumer 2516805376 consumed 8 at position o.. (_. 1, 4, 7, 1] Producer 2449663744 produced 6 at position e. (6, 1, 4, 7, 1] Consumer 2508412672 consumed 6 at position 0.. 1, 4, 7, 1] Producer 2491627264 produced i at position 0... .[1, 1, 4, 7, 11 (base) king@king-Latitude-E5540:-/Documents/oshonework/os-ass3/dequeues Objective: To understand and experiment with the implementation of synchronization. Problem Statement: Design and implement a solution for Producer-Consumer Problem using Pthreads library in Linux virtual machine. Steps: . Download buffer.h and the incomplete version of hw3.c from iCollege Read through Programming Project 3 of Chapter 5 (Producer-Consumer Problem) in the textbook. Follow the suggestion in the textbook to solve the problem using Pthreads. Do NOT solve the problem using Windows API. Instead of implementing the buffer as a circular queue as stated in the textbook, implement the buffer as a Dequeue (Doubly Ended Queue, i.e.insertion and deletion can be done at both end of the queue). o Adequeue has different variants. In this assignment, the behaviors of consumer and producer are defined as follows: The producer should add an element to the side that is farther from its queue end (right or left end); Contrarily, the consumer should remove an element from the side that is closer to its queue end (right or left end) For example, given a non-empty dequeue: bc consumer will remove one element from the left, i.e., element b, because the left side is closer to its end (left end). Producer will add an element on the right, i.e., after c, because the right side is farther from its queue end (right end). If the distances from the left-most element to the left end the distance from the right-most element to the right end are the same, it requires insertion on the right and removal on the left. For example, given a non-empty dequeue: abc consumer will remove element a, and producer will add an element after c. If the queue is empty, the producer will add the first element to the left of the array. In addition to the requirement in the textbook, print a message every time an item is produced or consumed in your producer or consumer threads. The message should also include the thread ID of the producer or consumer, consumed or produced item, the insertion or removal position, and the current buffer content (see the screenshot below). To get a thread's ID, you may call pthread_self() function and convert the result into an integer. For example, printf("Consumer %u",(unsigned int)pthread_self()); will print the consumer ID. Compile the source file using gcc-pthread -o hw3 hw3.c Use ./hw3 to test the program. The number of producer threads and number of consumer threads should be larger than 1. For consistency, use ./hw3 5 8 5 for the final submission. Print out the program output message after you run the program (see the screenshot below). O king@king-Latitude E5540:-/Documents/oshomework/os-ass3/dequeue (base) king@king-Latitude-E5540:-/Documents/oshonework/os-ass3/dequeue$ /hw3 5 8 5 Producer 2491627264 produced 8 at position 0... ...[8, Consumer 2541983488 consumed 8 at position 0... Producer 2466449152 produced 6 at position 0... [6, Producer 2483234560 produced 9 at position 1... 6, 9 Producer 2474841856 produced 4 at position 2... [6, 9, 4, _ Producer 2560019968 produced 4 at position 3... Producer 2458056448 produced 8 at position 4. [6, 9, 4, 4, Consumer 2533590784 consumed 6 at position o... 9, 4, 4. Consumer 2525198688 consumed 8 at position 4... Consumer 2516805376 consumed 9 at position 1... Consumer 2508412672 consumed 4 at position 3... Producer 2449663744 produced 7 at position 3... Producer 2441271040 produced i at position 1. 1, 4, 7, Producer 2491627264 produced 3 at position 4.. 1, 4, 7, 3) Consumer 2541983488 consumed 3 at position 4. L. 1, 4, 7, _) Producer 2466449152 produced i at position 4. -- 1, 4, 7, 1] Producer 2483234566 produced 5 at position 0.. (5, 1, 4, 7, 1] Consumer 2525198688 consumed 5 at position o.. 1, 4, 7, 1] Consumer 2533590784 consumed i at position 4.. 1, 4, 7, Producer 2474841856 produced 5 at position 4. . 1, 4, 7, 5] Producer 2506019968 produced 8 at position e. 8, 1, 4, 7, 5] Consumer 2516805376 consumed 8 at position 0. 1, 4, 7, 5] Consumer 2508412672 consumed 5 at position 4. - 1, 4, 7, _ Producer 2458856448 produced 9 at position 4.. 1, 4, 7, 9] Producer 2441271848 produced 3 at position 0.. (3, 1, 4, 7, 9j Consumer 2541983488 consumed 3 at position o... 1, 4, 7, 9] Producer 2449663744 produced 9 at position o... [9, 1, 4, 7, 9) Consumer 2525198680 consumed 9 at position 0... -. 1, 4, 7, 9] Producer 2491627264 produced 6 at position 0. [6, 1, 4, 7, 9) Consumer 2533590784 consumed 6 at position o.. 1, 4, 7, 9] Producer 2500019968 produced 3 at position 0.. (3, 1, 4, 7, 9] Consumer 2516805376 consumed 3 at position o... 1, 4, 7, 9] Consumer 2568412672 consumed 9 at position 4.. -- 1, 4, 7, _) Producer 2483234560 produced i at position 4... - 1, 4, 7, 1] Producer 2474841856 produced 4 at position o.. [4, 1, 4, 7, 1] Consumer 2541983488 consumed 4 at position 0. -- 1, 4, 7, 1] Producer 2466449152 produced 5 at position o.. [5, 1, 4, 7, 1] Consumer 2525198080 consumed 5 at position 0.. -, 1, 4, 7, 1] Producer 2441271840 produced 6 at position e... [6, 1, 4, 7, 1] Consumer 2533598784 consumed 6 at position ... 1, 4, 7, 1] Producer 2458056448 produced 8 at position 0... [8, 1, 4, 7, 1] Consumer 2516805376 consumed 8 at position o.. (_. 1, 4, 7, 1] Producer 2449663744 produced 6 at position e. (6, 1, 4, 7, 1] Consumer 2508412672 consumed 6 at position 0.. 1, 4, 7, 1] Producer 2491627264 produced i at position 0... .[1, 1, 4, 7, 11 (base) king@king-Latitude-E5540:-/Documents/oshonework/os-ass3/dequeues