Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I have the below code that runs without errors but the problem I am having is that if you enter the wrong number for radius
I have the below code that runs without errors but the problem I am having is that if you enter the wrong number for radius it tells you correctly that it must be or greater but instead of asking again it moves right to Fill color of the circle instead. If you enter nothing or blank it just goes to another line and doesn't complete. What is wrong with my error checking code?
Circle.h
#ifndef CIRCLEH
#define CIRCLEH
#include
class Circle
private:
int radius; Radius of the circle must be or greater
std::string colorFill; Fill color of the circle cannot be blank
public:
Constructors
Circle; Zeroargument constructor
Functions
double calcDiameter const; Calculate and return the diameter of the circle
double calcArea const; Calculate and return the area of the circle
double calcCircumference const; Calculate and return the circumference of the circle
void setRadiusint r; Set the radius of the circle
int getRadius const; Get the radius of the circle
void setColorFillconst std::string& color; Set the fill color of the circle
std::string getColorFill const; Get the fill color of the circle
Nonclass function
void displayCircleInfo const; Display the formatted output of the circle information
;
#endif
Circle.cpp
#include "Circle.h
#include
#include
const double PI ;
Constructors
Circle::Circle : radius colorFill
Functions
double Circle::calcDiameter const
return staticcastradius;
double Circle::calcArea const
return PI powstaticcastradius;
double Circle::calcCircumference const
return PI staticcastradius;
void Circle::setRadiusint r
if r
radius r;
else
std::cerr "Error: Radius must be or greater." std::endl;
int Circle::getRadius const
return radius;
void Circle::setColorFillconst std::string& color
if color.empty
colorFill color;
else
std::cerr "Error: Fill color cannot be blank." std::endl;
std::string Circle::getColorFill const
return colorFill;
Nonclass function
void Circle::displayCircleInfo const
std::cout "Circle Information:" std::endl;
std::cout "Radius: getRadius std::endl;
std::cout "Fill Color: getColorFill std::endl;
std::cout "Diameter: calcDiameter std::endl;
std::cout "Area: calcArea std::endl;
std::cout "Circumference: calcCircumference std::endl;
CircleDriver.cpp
#include "Circle.h
#include
#include
void displayCircleInfoconst Circle& circle
std::cout std::setw std::left "Radius:" std::setw std::left circle.getRadius std::endl;
std::cout std::setw std::left "Diameter:" std::setw std::left circle.calcDiameter std::endl;
std::cout std::setw std::left "Area:" std::setw std::left circle.calcArea std::endl;
std::cout std::setw std::left "Circumference:" std::setw std::left circle.calcCircumference std::endl;
std::cout std::setw std::left "Color Fill:" std::setw std::left circle.getColorFill std::endl;
int main
std::cout "Brandon Gauntt Lab Class Creation std::endl;
int radius;
Circle circle;
do
std::cout "Radius of Circle as a positive number to exit: ;
std::cin radius;
if radius
break;
circle.setRadiusradius;
std::string color;
std::cout "Fill Color of Circle blank not allowed: ;
std::cin color;
circle.setColorFillcolor;
displayCircleInfocircle;
Pause for the user to read the displayed information
systempause;
while true;
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
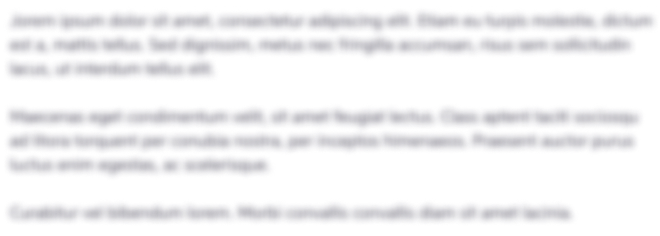
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started