Question
I have the code but its not working showing similar error like invalid syntax in line 5 the code is bellow the question at the
I have the code but its not working showing similar error like invalid syntax in line 5 the code is bellow the question at the very end
IT 212 -- Project 2
- Goal: write an application that computes bowling scores.
- Deliverables:
Filename Description readraggedarray.py Definition of read_ragged_array method framescore.py Definition of frame_score method testframescore.py Unit test file for frame_score method bowling.py Main source code file that calls other methods and implements the pseudocode game1.txt, game2.txt, game3.txt Input files that contain game scores - Bowling terminology:
- Bowling is a game in which the player attempts to knock down ten ten bowling pins with a bowling ball. A bowling game consists of ten frames. In a frame, a player rolls one or two balls to knock down as many pins as possible. A player bowls a strike in a frame if he or she knocks down all ten pins with one ball. A strike is denoted by X. A player bowls a spare in a frame if he or she knocks down all ten pins with two balls. A spare is denoted by /. An open frame results if a player fails to knock down all ten pins with two balls in that frame.
- Bowling scoring: The score for an open frame is the number of balls knocked down in that frame. Here is a bowling game consisting of only open frames (no strikes or spares): Game 1
Frame Number 1 2 3 4 5 6 7 8 9 10 Bonus +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Ball Scores | 8 | 0 | 7 | 2 | 6 | 0 | 6 | 2 | 0 | 8 | 6 | 1 | 9 | 0 | 8 | 0 | 8 | 1 | 5 | 2 | | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Frame Scores | 8 | 9 | 6 | 8 | 8 | 7 | 9 | 8 | 9 | 7 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Running Total | 8 | 17 | 23 | 31 | 39 | 46 | 55 | 63 | 72 | 79 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
The total score for Game 1 is 79. The score for a spare is 10 plus the bonus ball in the next frame. Game 2Frame Number 1 2 3 4 5 6 7 8 9 10 Bonus +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Ball Scores | 8 | 1 | 7 | / | 5 | 3 | 0 | / | 7 | / | 9 | / | 9 | 0 | 8 | / | 8 | 1 | 5 | / | 1 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Frame Scores | 9 | 15 | 8 | 17 | 19 | 19 | 9 | 18 | 9 | 11 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Running Total | 9 | 24 | 32 | 39 | 68 | 87 | 96 | 114 | 123 | 134 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
In Game 2, Frame 2, the score is 10 for the spare plus the bonus ball 5, which is the first ball in Frame 3: 10 + 5 = 15. If a player scores a spare in Frame 10, he or she is allowed one more bonus ball. In Game 2, Frame 10, the score is 10 + 1 = 11. The score for a strike is 10 for the strike plus the sum of the next two balls. Game 3Frame Number 1 2 3 4 5 6 7 8 9 10 Bonus +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Ball Scores | 8 | 1 | X | | 6 | / | 8 | 1 | 9 | / | X | | X | | X | | 9 | / | X | | X | 8 | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Frame Scores | 9 | 20 | 18 | 9 | 20 | 30 | 29 | 20 | 20 | 28 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+ Running Total | 9 | 29 | 47 | 56 | 76 | 106 | 135 | 155 | 175 | 203 | | +-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+-------+
In Game 3, Frame 2, the score is 10 for the strike plus the next two balls, which are 6 and 4: 10 + 6 + 4 = 20. In Frame 6, the score is 10 for the strike plus the next two balls , which are 10 and 10: 10 + 10 + 10 = 30. In Frame 7, the score is 10 + 10 + 9 = 29. In Frame 10, if the player rolls a strike, he or she gets two more bonus balls. The score for Frame 10 is 10 + 10 + 8 = 28. - Writing the script to compute bowling scores:
- A bowling frame is represented by a Fixnum array of length 2 if the frame is a spare or an open frame, for example [6, 4] or [5, 3]. A bowling frame is represented by an array of length 1 if the frame is a strike: [10].
- A bowling game is represented by a ragged array containing its frames, including the bonus balls if the last frame is a strike or a spare. For example, here are the representations of Games 1, 2, and 3:
Game Number File Name Ragged Array Game 1 game1.txt [["Frame0"], [8,0],[7,2],[6,0],[6,2], [0,8],[6,1],[9,0],[8,0],[8,1],[5,2]] Game 2 game2.txt [["Frame0"], [8,1],[7,3],[5,3],[0,10], [7,3],[9,1],[9,0],[8,2],[8,1], [5,5],[8]] Game 3 game3.txt [["Frame0"], [8,1],[10],[6,4],[8,1], [9,1], [10],[10],[10], [9,1], [10],[10],[8]] - Write a method named frame_score in the file framescore.py with this header:
def frame_score(the_frame, bonus1, bonus2):
Use an if..else statement with three cases. Here is the pseudocode for the body of the frame_score method:# Case where frame is a strike If the_frame[0] is equal to 10 Return 10 plus bonus1 plus bonus2. # Case where frame is a spare Else if the_frame[0] plus the_frame[1] is equal to 10 Return 10 plus bonus1. # Case where frame is an open frame Else Return the_frame[0] plus the_frame[1]. End if
- Use this unit test script to test your frame_score method:
# Filename: testframescore.py import unittest from framescore import frame_score class TestFrameScore(unittest.TestCase): def test_1(self): self.assertEqual(frame_score([10], 10, 7), 27) def test_2(self): self.assertEqual(frame_score([7, 3], 9, 0), 19) def test_3(self): self.assertEqual(frame_score([7, 1], 0, 0), 8) if __name__ == '__main__': unittest.main()
- Write a main script in the file bowling.py that computes the bowling score from the scores for a game in an input file:
- Prompt the user for the name of the input file, for example, game1.txt, game2.txt, or game3.txt.
- Use the read_ragged_array method from the RaggedArray Example to read the game scores from the input file into a ragged array (see the table above). Make these changes to the read_ragged_array method before using it:
- Change the delimiter in the split method to be " " instead of ",".
- Store the values in the ragged array as int values instead of float.
- Store the frames in an array named frames. Also, instead of initializing the frames array to [ ], initialize it to [["Frame0"]] so that the first actual bowling frame in frames is at index 1. ["Frame0"] is a placeholder at index 0.
- Loop over each frame in the frames array to compute the sum of all the return values of the frame_score method calls.
Import the frame_score method from the file framescore.py Import the read_ragged_array method from the framescore.py file. (See the RaggedArray Example) read inputfile from keyboard, promping user. Call the read_ragged_array method to create a ragged array of the data in the input_file like game1.txt, game2.txt, or game3.txt. Assign the ragged array to the variable frames. Initialize score to 0. For i in the range from 1 to 10 (use range(1, 11)) if frames[i][0] equals 10 and frames[i+1][0] equals 10 assign 10 to bonus1. assign frames[i+2][0] to bonus2. elsif frames[i][0] equals 10 and frames[i+1][0] < 10 assign frames[i+1][0] to bonus1. assign frames[i+1][1] to bonus2. elsif frames[i][0] + frames[i][1] equals 10 assign frames[i+1][0] to bonus1. assign zero to bonus2. else assign zero to bonus1. assign zero to bonus2. end if # Next line is actual Python statement. score += framescore(frames[i], bonus1, bonus2) End loop. Print score. this is my code
-
# Number of normal Frame in a game.
LIMIT = 10
# Total Number of pin lined up COUNT = 10
class BowlingExp
class Frame_Range attr_reader :remaining_pin, shots
def initialize(Frame_Range_num) @Frame_Range_num = Frame_Range_num @remaining_pin = COUNT @shots = [] end
#Call BowlingGame#play first.Do not use this method directly;
def register_shot(pin) if !played? @remaining_pin -= pin end
if !score_finalized? @shots << pin end
def score @shots.inject(0, :+) end
def strike? shots.first == COUNT end
def spare? !strike? && remaining_pin == 0 end
# If a strike or two shots have been played return true def played? remaining_pin == 0 || shots.length == 2 end
# If the score has been finalized return true: no more bonus shots
def score_finalized? if @Frame_Range_num > LIMIT played? # No bonuses else shots.length == ((strike? || spare?) ? 3 : 2 : 1) end end
def to_s strike? ? 'X' : spare? ? "#{shots[0]}/" : shots[0...2].join() end end
attr_reader :Frame_Ranges
def initialize @Frame_Ranges = [] new_Frame_Range() end
def play(&block) throw BowlingExp.new("Game over") if completed? pin = yield Frame_Ranges.last.remaining_pin, Frame_Ranges.last.shots.length throw BowlingExp.new("Shot Impossible") if pin > Frame_Ranges.last.remaining_pin Frame_Ranges.each { |f| f.register_shot(pin) } new_Frame_Range() if Frame_Ranges.last.played? && !completed? end
def completed? Frame_Ranges.length >= LIMIT && Frame_Ranges[LIMIT - 1].score_finalized? end
def score Frame_Ranges[0...LIMIT].collect { |f| f.score }.inject(0, :+) end
def to_s Frame_Ranges.collect { |f| f.to_s }.join('-') end
private def new_Frame_Range @Frame_Ranges << Frame_Range.new(@Frame_Ranges.length + 1) end end
Step by Step Solution
There are 3 Steps involved in it
Step: 1
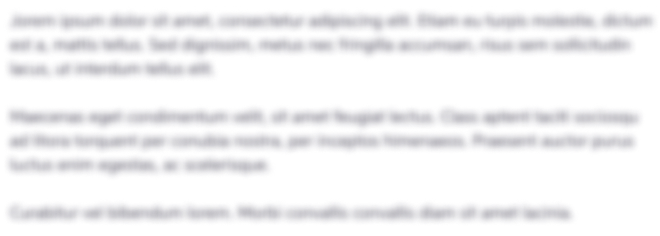
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started