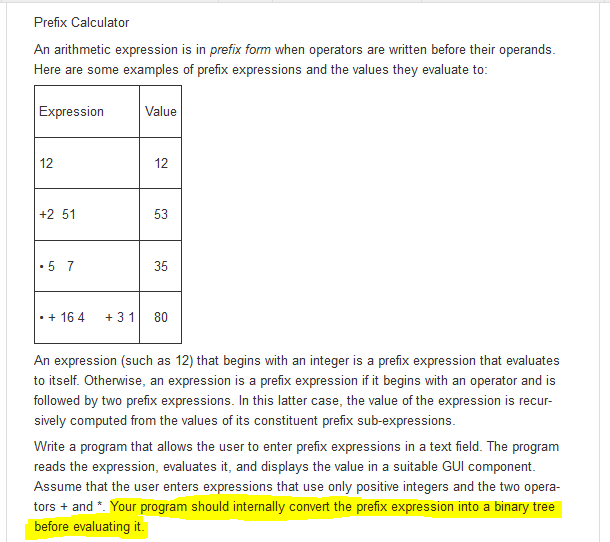
I have the code, however it wants me to internally convert the prefix expression into a binary tree before evaluating it. How do I make the program convert the expression to a binary tree?
T_Applet class
import java.applet.*; import java.awt.*; import java.awt.event.*; /* * This program demonstrates how to calculate expression * in infix form. */ public class T_Applet extends Applet implements ActionListener { /** * */ private static final long serialVersionUID = 1L; TextField input; TextField output; public void init() { // Construct the textFields input = new TextField(40); output = new TextField(40); // add the text fields to the layout add(input); add(output); // add the ActionListenerInterface input.addActionListener((ActionListener) this); output.addActionListener((ActionListener) this); } public void actionPerformed(ActionEvent ae) { // Call the paint method repaint(); } public void paint(Graphics g) { // Creating an object Prefix Prefix objt = new Prefix(); // Reading input from text field 1 String str = input.getText(); // Invoke the evaluate method of Prefix object objt.evaluate(str); // Store the result in result int result = objt.getResult(); // Change the integer value to string and write it // to the output text field output.setText(Integer.toString(result)); }
}
Prefix class
import java.util.Stack; // Stack class is used for // storing the values of the expression
class Prefix { // Create a stack reference Stack stk; public Prefix() { // Create a stack object stk = new Stack(); } // get the results with the getResults method // returns the last element of the stack public int getResult() { return (Integer)(stk.pop()); } // evaluate method separates the operators and the // operands. public void evaluate(String input) { // Split the given input by basing on // delimiter space (" ") String [] str1 = input.split(" "); // Calculate the length of Array // The number of elements in the given expression int length = str1.length; // Run the loop from bottom of the array to the top for (int i = (length-1); i >= 0; i--) { // Check whether the string is number or not if (isNumber(str1[i])) { // push the string after converting it // to an integer stk.push(new Integer(Integer.parseInt(str1[i]))); } else if(str1[i].equals("+") || str1[i].equals("*")) { // push the results after performing the calculation stk.push(new Integer(doCalculation(str1[i]))); } else { // Wrong format is entered System.out.println(" Sorry wrong format! Please enter the right format"); } } } // Check whether the given string is integer or not public boolean isNumber(String str2) { try { int p = Integer.parseInt(str2); } catch (NumberFormatException e) { return false; } return true; } // perform calculation if the operator is found public int doCalculation (String str) { // Try for possibility of occurrence // of EmptyStackException try { // Read top of the stack int p = (Integer)(stk.pop()); // Read top of the stack int q = (Integer)(stk.pop()); // Check operations if(str.equals("+")) return (p+q); else return (p*q); } catch(java.util.EmptyStackException e) { // Exception Caught return 0; } } }
Prefix Calculator An arithmetic expression is in prefix form when operators are written before their operands Here are some examples of prefix expressions and the values they evaluate to Expression Value 12 12 +2 51 53 35 1643180 An expression (such as 12) that begins with an integer is a prefix expression that evaluates to itself. Otherwise, an expression is a prefix expression if it begins with an operator and is followed by two prefix expressions. In this latter case, the value of the expression is recur sively computed from the values of its constituent prefix sub-expressions Write a program that allows the user to enter prefix expressions in a text field. The reads the expression, evaluates it, and displays the value in a suitable GUI component Assume that the user enters expressions that use only positive integers and the two opera- tors + and Your program should internally convert the prefix expression into a binary tree before evaluating it program Prefix Calculator An arithmetic expression is in prefix form when operators are written before their operands Here are some examples of prefix expressions and the values they evaluate to Expression Value 12 12 +2 51 53 35 1643180 An expression (such as 12) that begins with an integer is a prefix expression that evaluates to itself. Otherwise, an expression is a prefix expression if it begins with an operator and is followed by two prefix expressions. In this latter case, the value of the expression is recur sively computed from the values of its constituent prefix sub-expressions Write a program that allows the user to enter prefix expressions in a text field. The reads the expression, evaluates it, and displays the value in a suitable GUI component Assume that the user enters expressions that use only positive integers and the two opera- tors + and Your program should internally convert the prefix expression into a binary tree before evaluating it program