Question
I have the following c++ code, it is a simple vector header file with a main to run it. I need to implement a bubble
I have the following c++ code, it is a simple vector header file with a main to run it. I need to implement a bubble sort function that re-orders the displayed simple vector values in descending order. I included a BubbleSort.cpp sample from the textbook that reorders a given int array in random order into ascending order. I need something similar but implemented into the intArray and doubleArray that is already working in the main, ordered in descending order since it is already ordered from smallest to biggest.
/////////////////// Main.cpp ////////////////////////////
// This program demonstrates the SimpleVector template.
#include
#include "SimpleVector.h"
using namespace std;
int main()
{
const int SIZE = 10; // Number of elements
int count; // Loop counter
// Create a SimpleVector of ints.
SimpleVector
// Create a SimpleVector of doubles.
SimpleVector
// Store values in the two SimpleVectors.
for (count = 0; count < SIZE; count++)
{
intTable[count] = (count * 2);
doubleTable[count] = (count * 2.14);
}
// Display the values in the SimpleVectors.
cout << "These values are in intTable: ";
for (count = 0; count < SIZE; count++)
cout << intTable[count] << " ";
cout << endl;
cout << "These values are in doubleTable: ";
for (count = 0; count < SIZE; count++)
cout << doubleTable[count] << " ";
cout << endl;
// Use the standard + operator on the elements.
cout << " Adding 5 to each element of intTable"
<< " and doubleTable. ";
for (count = 0; count < SIZE; count++)
{
intTable[count] = intTable[count] + 5;
doubleTable[count] = doubleTable[count] + 5.0;
}
// Display the values in the SimpleVectors.
cout << "These values are in intTable: ";
for (count = 0; count < SIZE; count++)
cout << intTable[count] << " ";
cout << endl;
cout << "These values are in doubleTable: ";
for (count = 0; count < SIZE; count++)
cout << doubleTable[count] << " ";
cout << endl;
// Use the standard ++ operator on the elements.
cout << " Incrementing each element of intTable and"
<< " doubleTable. ";
for (count = 0; count < SIZE; count++)
{
intTable[count]++;
doubleTable[count]++;
}
// Display the values in the SimpleVectors.
cout << "These values are in intTable: ";
for (count = 0; count < SIZE; count++)
cout << intTable[count] << " ";
cout << endl;
cout << "These values are in doubleTable: ";
for (count = 0; count < SIZE; count++)
cout << doubleTable[count] << " ";
cout << endl;
//Use BubbleSort to create a set of values and re-order them in ascending order
//bubblesort implementation
system("pause");
return 0;
}
//////////////////////// SimpleVector.h //////////////////////////
// SimpleVector class template
#ifndef SIMPLEVECTOR_H
#define SIMPLEVECTOR_H
#include
#include
#include
using namespace std;
template
class SimpleVector
{
private:
T *aptr; // To point to the allocated array
int arraySize; // Number of elements in the array
void memError(); // Handles memory allocation errors
void subError(); // Handles subscripts out of range
public:
// Default constructor
SimpleVector()
{
aptr = 0; arraySize = 0;
}
// Constructor declaration
SimpleVector(int);
// Copy constructor declaration
SimpleVector(const SimpleVector &);
// Destructor declaration
~SimpleVector();
// Accessor to return the array size
int size() const
{
return arraySize;
}
// Accessor to return a specific element
T getElementAt(int position);
// Overloaded [] operator declaration
T &operator[](const int &);
};
//************************************************************
// Constructor for SimpleVector class. Sets the size of the *
// array and allocates memory for it. *
//************************************************************
template
SimpleVector
{
arraySize = s;
// Allocate memory for the array.
try
{
aptr = new T[s];
}
catch (bad_alloc)
{
memError();
}
// Initialize the array.
for (int count = 0; count < arraySize; count++)
*(aptr + count) = 0;
}
//*******************************************
// Copy Constructor for SimpleVector class. *
//*******************************************
template
SimpleVector
{
// Copy the array size.
arraySize = obj.arraySize;
// Allocate memory for the array.
aptr = new T[arraySize];
if (aptr == 0)
memError();
// Copy the elements of obj's array.
for (int count = 0; count < arraySize; count++)
*(aptr + count) = *(obj.aptr + count);
}
//**************************************
// Destructor for SimpleVector class. *
//**************************************
template
SimpleVector
{
if (arraySize > 0)
delete[] aptr;
}
//********************************************************
// memError function. Displays an error message and *
// terminates the program when memory allocation fails. *
//********************************************************
template
void SimpleVector
{
cout << "ERROR:Cannot allocate memory. ";
exit(EXIT_FAILURE);
}
//************************************************************
// subError function. Displays an error message and *
// terminates the program when a subscript is out of range. *
//************************************************************
template
void SimpleVector
{
cout << "ERROR: Subscript out of range. ";
exit(EXIT_FAILURE);
}
//*******************************************************
// getElementAt function. The argument is a subscript. *
// This function returns the value stored at the *
// subcript in the array. *
//*******************************************************
template
T SimpleVector
{
if (sub < 0 || sub >= arraySize)
subError();
return aptr[sub];
}
//********************************************************
// Overloaded [] operator. The argument is a subscript. *
// This function returns a reference to the element *
// in the array indexed by the subscript. *
//********************************************************
template
T &SimpleVector
{
if (sub < 0 || sub >= arraySize)
subError();
return aptr[sub];
}
#endif
////////////////////////// BubbleSort.cpp /////////////////////////////////////////
// This program uses the bubble sort algorithm to sort an
// array in ascending order.
#include
using namespace std;
// Function prototypes
void sortArray(int[], int);
void showArray(const int[], int);
int main()
{
// Array of unsorted values
int values[6] = { 7, 2, 3, 8, 9, 1 };
// Display the values.
cout << "The unsorted values are: ";
showArray(values, 6);
// Sort the values.
sortArray(values, 6);
// Display them again.
cout << "The sorted values are: ";
showArray(values, 6);
return 0;
}
//*********************
// Definition of function sortArray *
// This function performs an ascending order bubble sort on *
// array. size is the number of elements in the array. *
//*********************
void sortArray(int array[], int size)
{
bool swap;
int temp;
do
{
swap = false;
for (int count = 0; count < (size - 1); count++)
{
if (array[count] > array[count + 1])
{
temp = array[count];
array[count] = array[count + 1];
array[count + 1] = temp;
swap = true;
}
}
} while (swap);
}
//*********************
// Definition of function showArray. *
// This function displays the contents of array. size is the *
// number of elements. *
//*********************
void showArray(const int array[], int size)
{
for (int count = 0; count < size; count++)
cout << array[count] << " ";
cout << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
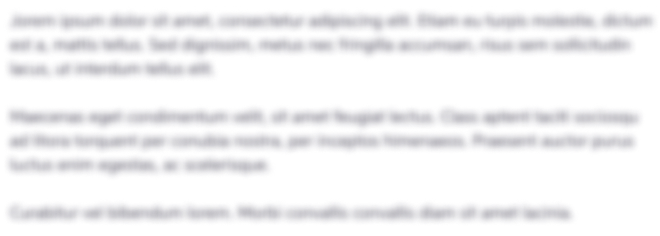
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started