Question
I have the following classes, Project1, DateGUI, and Date212. Project1 is meant to take an unsorted textfile, and output to a one row, two column
I have the following classes, Project1, DateGUI, and Date212. Project1 is meant to take an unsorted textfile, and output to a one row, two column GUI the unsorted information read from the text file to the left column, and the sorted version to the right column. The format for the right(sorted) column is called from Date212, which takes the information from the textfile and outputs in the form month dd , yyyy (example 20130531 will output as May 31 , 2013). I already have some code for these but the output is not what I need and I am not sure how to fix it. I will also provide a class textfileinput which I used to all Project1 to read the textfile. I think the problems are only with Project1. Thank you for any assistance.
input: Date.txt
20141001 20080912,20131120,19980927 20020202 20120104
The output to the GUI should be in the form:
Unsorted | Sorted
20141001 | September 27, 1998
20080912 | February 2, 2002
20131120 | September 12, 2008
19980927 | January 4, 2012
20020202 | November 20, 2013
20120104 | October 1, 2014
_______________________________________________________________
import java.awt.Container; import java.awt.TextArea; import java.util.StringTokenizer;
public class Project1 { static final int LIST_SIZE = 100; static TextFileInput inFile; static DateGUI myDateGUI; public static void main(String[] args) { myDateGUI = new DateGUI("Date List", 500, 300); String[] dataSource = new String[LIST_SIZE]; int numDate = readDatesFromFile("Date.txt", dataSource); System.out.println("The number of Date is: "+ numDate); printDatetoJFrame(myDateGUI, dataSource, numDate); } public static int readDatesFromFile(String myFile, String[] dateList){ TextFileInput in = new TextFileInput(myFile); int lengthFilled = 0; String line = in.readLine(); while (lengthFilled < dateList.length && line != null) { dateList[lengthFilled++] = line; line = in.readLine(); } // while if (line != null) { System.out.println("File contains too many dates."); System.out.println("This program can process only " + dateList.length + " dates."); System.exit(1); } // if in.close(); return lengthFilled; }
private static void swap(Date212[] a, int x, int y) { //helper method for the 'selectionSort' method below: Date212 temp; temp = a[x]; a[x] = a[y]; a[y] = temp; }
private static void selectionSort(Date212[] array, int length) { for (int i = 0; i < length - 1; i++) { int indexLowest = i; for (int j = i + 1; j < length; j++) { if (array[j].compareTo(array[indexLowest]) < 0) { indexLowest = j; if (array[indexLowest].compareTo(array[i]) < 0) swap(array, indexLowest, i); } } } }
public static void printDatetoJFrame(DateGUI myDateGUI, String[] dl, int numDate) {
Container myContentPane = myDateGUI.getContentPane(); // get content pane from the myDateGUI TextArea myTextArea = new TextArea(); TextArea mySubscripts = new TextArea(); myContentPane.add(myTextArea); myContentPane.add(mySubscripts); for (int i=0;i mySubscripts.append(Integer.toString(i)+" "); myTextArea.append(dl[i]+" "); } //create two text areas // put the dates read from the file into the first text area by .setText method // put the formatted dates into the second text area use the same method myDateGUI.setVisible(true); }
}
_____________________________________________________________________________________
class Date212{ private String monthsInYear[]={"","January","February","March","April","May","June","July","August","September","October","November","December"}; private int month; private String monthString; private int day; private int year; public Date212 (int y, int m, int d) { //Validating month if(m < 1 || m > 12) throw new IllegalArgumentException("Month cannot be less than 1 or more than 12.");
//Validating day if(d < 1 || d > 31) throw new IllegalArgumentException("Day cannot be less than 1 or more than 32."); { month=m; day=d; year=y; monthString = monthsInYear[m]; } } public int getDay() { return day; } public int getMonth() { return month; } public int getYear() { return year; } public void setDay(int d) { if(d<1 || d>31) throw new IllegalArgumentException("Day must be more than 0 and less than or equal to 31."); day=d; } public void setMonth(int m) { if(m<1 || m>12) throw new IllegalArgumentException("Month must be more than 0 and less than 13."); month=m; } public void setYear(int y) { year=y; } public Date212 (String d) { //Calling three argument constructor this(Integer.parseInt(d.substring(0, 4)), Integer.parseInt(d.substring(4, 6)), Integer.parseInt(d.substring(6, 8))); }
//Method that returns date in the required format public String toString() { return monthString + " " + String.format("%02d", day) + "," + String.format("%04d", year); }
public static void main(String[] args) { Date212 date1 = new Date212("20130531"); Date212 date2 = new Date212("20121231"); System.out.println(date1.year); System.out.println(date1.month); System.out.println(date1.day); System.out.println(date1.toString()); System.out.println("compare: "+date1.compareTo(date2)); }
public String compareTo(Date212 another) {
if (this.getDay() == another.getDay() && this.getMonth() == another.getMonth()
&& this.getYear() == another.getYear()) {
return "Both are same";
}
if (this.getYear() > another.getYear()) {
return this.toString() + " is greater than " + another.toString();
} else if (this.getYear() < another.getYear()) {
return this.toString() + " is lesser than " + another.toString();
} else {
if (this.getMonth() > another.getMonth()) {
return this.toString() + " greater than " + another.toString();
} else if (this.getMonth() < another.getMonth()) {
return this.toString() + " lesser than " + another.toString();
} else {
if (this.getDay() > another.getDay()) {
return this.toString() + " greater than " + another.toString();
} else if (this.getDay() < another.getDay()) {
return this.toString() + " lesser than " + another.toString();
}
}
}
return "";
}
// compare this date212 with other date212 // compare the year first, then the month, then the day // return -1 if this < other // return 0 if this == other // return 1 if this > other }
__________________________________________________________________________________________________________________
import java.awt.GridLayout;
import javax.swing.*;
public class DateGUI extends JFrame{ public DateGUI(String title, int height, int width) { setSize(400, 400); // set the size of the JFrame setLocation(100, 100); // set the location of the JFrame setTitle("Project 1"); // set the title of the JFrame setLayout(new GridLayout(1, 2)); // set the layout of the JFrame, setLayout(new GridLayout(1,2)); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); }
}
______________________________________________________________________________________________________________
// TextFileInput.java // Copyright (c) 2000, 2005 Dorothy L. Nixon. All rights reserved.
import java.io.BufferedReader; import java.io.FileInputStream; import java.io.InputStreamReader; import java.io.IOException;
/** * Simplified buffered character input * stream from an input text file. * Manages an input text file, * handling all IOExceptions by generating * RuntimeExcpetions (run-time error * messages). * * If the text file cannot be created, * a RuntimeException is thrown, * which by default results an an * error message being printed to * the standard error stream. * * @author D. Nixon */ public class TextFileInput {
/** Name of text file */ private String filename;
/** Buffered character stream from file */ private BufferedReader br;
/** Count of lines read so far. */ private int lineCount = 0;
/** * Creates a buffered character input * strea, for the specified text file. * * @param filename the input text file. * @exception RuntimeException if an * IOException is thrown when * attempting to open the file. */ public TextFileInput(String filename) { this.filename = filename; try { br = new BufferedReader( new InputStreamReader( new FileInputStream(filename))); } catch ( IOException ioe ) { throw new RuntimeException(ioe); } // catch } // constructor
/** * Closes this character input stream. * No more characters can be read from * this TextFileInput once it is closed. * @exception NullPointerException if * the file is already closed. * @exception RuntimeException if an * IOException is thrown when * closing the file. */ public void close() { try { br.close(); br = null; } catch ( NullPointerException npe ) { throw new NullPointerException( filename + "already closed."); } catch ( IOException ioe ) { throw new RuntimeException(ioe); } // catch } // method close
/** * Reads a line of text from the file and * positions cursor at 0 for the next line. * Reads from the current cursor position * to end of line. * Implementation does not invoke read. * * @return the line of text, with * end-of-line marker deleted. * @exception RuntimeException if an * IOException is thrown when * attempting to read from the file. */ public String readLine() { return readLineOriginal(); } // method readLine()
/** * Returns a count of lines * read from the file so far. */ public int getLineCount() { return lineCount; }
/** * Tests whether the specified character is equal, * ignoring case, to one of the specified options. * * @param toBeChecked the character to be tested. * @param options a set of characters * @return true if toBeChecked is * equal, ignoring case, to one of the * options, false otherwise. */ public static boolean isOneOf(char toBeChecked, char[] options) { boolean oneOf = false; for ( int i = 0; i < options.length && !oneOf; i++ ) if ( Character.toUpperCase(toBeChecked) == Character.toUpperCase(options[i]) ) oneOf = true; return oneOf; } // method isOneOf(char, char[])
/** * Tests whether the specified string is one of the * specified options. Checks whether the string * contains the same sequence of characters (ignoring * case) as one of the specified options. * * @param toBeChecked the String to be tested * @param options a set of Strings * @return true if toBeChecked * contains the same sequence of * characters, ignoring case, as one of the * options, false otherwise. */ public static boolean isOneOf(String toBeChecked, String[] options) { boolean oneOf = false; for ( int i = 0; i < options.length && !oneOf; i++ ) if ( toBeChecked.equalsIgnoreCase(options[i]) ) oneOf = true; return oneOf; } // method isOneOf(String, String[]) /** * Reads a line from the text file and ensures that * it matches one of a specified set of options. * * @param options array of permitted replies * * @return the line of text, if it contains the same * sequence of characters (ignoring case for * letters) as one of the specified options, * null otherwise. * @exception RuntimeException if the line of text * does not match any of the specified options, * or if an IOException is thrown when reading * from the file. * @exception NullPointerException if no options are * provided, or if the end of the file has been * reached. */ public String readSelection(String[] options) { if ( options == null || options.length == 0 ) throw new NullPointerException( "No options provided for " + " selection to be read in file " + filename + ", line " + (lineCount + 1) + ".");
String answer = readLine();
if ( answer == null ) throw new NullPointerException( "End of file " + filename + "has been reached.");
if ( !TextFileInput.isOneOf(answer, options) ) { String optionString = options[0]; for ( int i = 1; i < options.length; i++ ) optionString += ", " + options[i]; throw new RuntimeException("File " + filename + ", line " + lineCount + ": \"" + answer + "\" not one of " + optionString + "."); } // if return answer; } // method readSelection
/** * Reads a line from the text file and ensures that * it matches, ignoring case, one of "Y", "N", "yes", * "no", "1", "0", "T", "F", "true", or "false". * There must be no additional characters on the line. * * @return true if the line matches * "Y", "yes", "1" "T", or "true". * false if the line matches * "N", "no", "0", "F", or "false". * @exception RuntimeException if the line of text * does not match one of "Y", "N", "yes", * "no", "1", "0", "T", "F", "true", or "false", * or if an IOException is thrown when reading * from the file. * @exception NullPointerException if the end of the * file has been reached. */ public boolean readBooleanSelection() { String[] options = {"Y", "N", "yes", "no", "1", "0", "T", "F", "true", "false"}; String answer = readSelection(options); return isOneOf(answer, new String[] {"Y", "yes", "1", "T", "true"} ); } // method askUserYesNo
/** * Reads a line of text from the file and * increments line count. (This method * is called by public readLine and is * final to facilitate avoidance of side * effects when public readLine is overridden.) * * @return the line of text, with * end-of-line marker deleted. * @exception RuntimeException if an * IOException is thrown when * attempting to read from the file. */ protected final String readLineOriginal() { try { if ( br == null ) throw new RuntimeException( "Cannot read from closed file " + filename + "."); String line = br.readLine(); if ( line != null ) lineCount++; return line; } catch (IOException ioe) { throw new RuntimeException(ioe); } // catch } // method readLineOriginal } // class TextFileInput
Step by Step Solution
There are 3 Steps involved in it
Step: 1
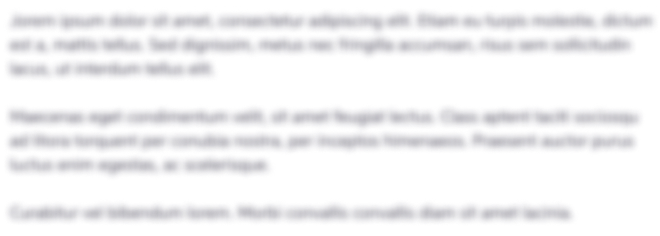
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started