Question
I have the shoppingcartprinter.java file already and the itemtopurchase files i will add them at the end of the post. i need to implement the
I have the shoppingcartprinter.java file already and the itemtopurchase files i will add them at the end of the post. i need to implement the following within those 2 files.
This program extends the earlier "Online shopping cart" program. (Consider first saving your earlier program).1. Extend the ItemToPurchase class per the following specifications Private fields string itemDescription - Initialized in default constructor to "none" Parameterized constructor to assign item name, item description, item price, and itemquantity (default values of 0). Public instance member methods setDescription() mutator & getDescription() accessor (2 pts) printItemCost() - Outputs the item name followed by the quantity, price, and subtotal printItemDescription() - Outputs the item name and descriptionEx. of printItemCost() output:
Bottled Water 10 @ $1 = $10Ex. of printItemDescription() output:Bottled Water: Deer Park, 12 oz.2. Create two new files ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() methodBuild the ShoppingCart class with the following specifications. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to"January 1, 2020" ArrayList cartItems where each item is of type ItemToPurchase Coupon code - Enum class with constants 10OFF, 20OFF, 30OFFPublic member methods Default constructor Parameterized constructor which takes the customer name and date as parameterso getCustomerName() accessoro getDate() accessor addItem() Adds an item to cartItems array. Has parameter ItemToPurchase. Does not return anythingremoveItem()o Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anythingo If item name cannot be found, output this message: Item not found in cart. Nothing removed. modifyItem()o Modifies an item's description, price, and/or quantity. Has parameter Bottled Water 10 @ $1 = $10Ex. of printItemDescription() output:Bottled Water: Deer Park, 12 oz.2. Create two new files ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() methodBuild the ShoppingCart class with the following specifications. Note: Some can be method stubs (empty methods) initially, to be completed in later steps. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to"January 1, 2020" ArrayList cartItems where each item is of type ItemToPurchase Coupon code - Enum class with constants 10OFF, 20OFF, 30OFFPublic member methods Default constructor Parameterized constructor which takes the customer name and date as parameterso getCustomerName() accessoro getDate() accessor addItem() Adds an item to cartItems array. Has parameter ItemToPurchase. Does not return anythingremoveItem()o Removes item from cartItems array. Has a string (an item's name) parameter. Does not return anythingo If item name cannot be found, output this message: Item not found in cart. Nothing removed. modifyItem()o Modifies an item's description, price, and/or quantity. Has parameter
ItemToPurchase. Does not return anything.o If item can be found (by name) in cart, check if parameter has default values for description, price, and quantity. If not, modify item in cart.o If item cannot be found (by name) in cart, output this message: Item not found in cart. Nothing modified. getNumItemsInCart() o Returns quantity of all items in cart. Has no parameters. getCostOfCart() o Determines and returns the total cost of items in cart. Has no parameters.applyCouponCode() A coupon code can be applied based on the following conditions: 10OFF - $10 off the total price if current total exceeds $50 20OFF - $20 off the total price if current total exceeds $100 30OFF - $20 off the total price if current total exceeds $150 printTotal()o outputs total of objects in cart. Apply any applicable coupon codes.o If invalid coupon code is applied, output the message: INVALID CODEo If cart is empty, output this message: SHOPPING CART IS EMPTYExample of PrintTotal()output:John Doe's Shopping Cart - February 1, 2020 Number of Items: 8Nike Romaleos 2 @$189 = $378 Chocolate Chips 5 @ $3 = $15Powerbeats 2 Headphones 1 @ $128 = $128Discount (30OFF): -$30 Total: $491 printDescription()o Outputs each items description. Example of printDescription()output:John Doe's Shopping Cart - February 1, 2020Item Descriptions
Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweetPowerbeats 2 Headphones: Bluetooth headphones
3. In the file ShoppingCartManager.java, have the main method prompt the user for a customer's name and today's date. o Output the name and date.o Create an object of type ShoppingCart. Output Example:Enter Customer's Name: John DoeEnter Today's Date:February 1, 2020Customer Name: John Doe Today's Date: February 1, 20204. Implement the printMenu() method in ShoppingCartManager.java. printMenu() has a ShoppingCart parameter, and outputs a menu of options to manipulate the shopping cart. Each option is represented by a single character. Build and output the menu within the methodo If there is an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. o Call printMenu() in the main() method. o Continue to execute the menu until the user enters q to Quit.Output Example:MENUa - Add item to cartd - Remove item from cart c - Change item quantityi - Output items' descriptions o - Output shopping cartp - Apply coupon codeq - QuitChoose an option:5. Implement Output shopping cart menu optionOutput Example:OUTPUT SHOPPING CARTJohn Doe's Shopping Cart - February 1, 2020 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128Discount (30OFF): -$30 Total: $491
6. Implement Output item's description menu option.Output Example:OUTPUT ITEMS' DESCRIPTIONSJohn Doe's Shopping Cart - February 1, 2020Item DescriptionsNike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweetPowerbeats 2 Headphones: Bluetooth headphones7. Implement Add item to cart menu option.Output Example:ADD ITEM TO CARTEnter the item name:Nike RomaleosEnter the item description: Volt color, Weightlifting shoes Enter the item price:189Enter the item quantity: 28. Implement Remove item menu optionOutput Example:REMOVE ITEM FROM CARTEnter name of item to remove: Chocolate Chips9. Implement Change item quantity menu option. Output Example:CHANGE ITEM QUANTITYEnter the item name: Nike RomaleosEnter the new quantity: 310. Implement the Apply coupon code menu option. When an invalid coupon code or a coupon code which doesnt meet the condition is applied, it should be rejected with the message INVALID CODE. Else, display the message COUPON APPLIED. Output Example:APPLY COUPON CODEEnter coupon code: 20OFFINVALID CODEEnter coupon code: 10OFFCOUPON APPLIED
ItemToPurchase.java
enum ItemTaxCategory { N,// no tax for item X,// 8% T;//10% } public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; private ItemTaxCategory category; public ItemToPurchase(){ this.itemName = "none"; this.itemPrice = 0; this.itemQuantity = 0; this.category = ItemTaxCategory.N; } public String getName() { return itemName; }
public void setName(String itemName) { this.itemName = itemName; }
public int getPrice() { return itemPrice; }
public void setPrice(int itemPrice) { this.itemPrice = itemPrice; }
public int getQuantity() { return itemQuantity; }
public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; }
public ItemTaxCategory getTaxCategory() { return category; }
public void setTaxCategory(ItemTaxCategory category) { this.category = category; } public double itemTotalPrice() { return getQuantity() * getPrice(); } public double totalTax() { double tax; if(category == ItemTaxCategory.X) { tax = 0.08 * getPrice(); }else if(category == ItemTaxCategory.T) { tax = 0.10 * getPrice(); }else { tax = 0; } return tax; } }
ShoppingCartPrinter.java
import java.util.Scanner;
public class ShoppingCartPrinter { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("Item 1"); ItemToPurchase item1 = getItem(sc); System.out.println("Item 2"); ItemToPurchase item2 = getItem(sc); double cost = item1.getPrice() + item1.totalTax() + item2.getPrice()+item2.totalTax(); System.out.println("TOTAL COST"); System.out.println(item1.getName() +" " + item1.getQuantity() +" @ "+item1.getPrice()+" = $" +item1.itemTotalPrice()); System.out.println(item2.getName() +" " + item2.getQuantity() +" @ "+item2.getPrice()+" = $" +item2.itemTotalPrice()); System.out.println("Tax = $"+(item1.totalTax() + item2.totalTax())); System.out.println("Total: $"+cost); } public static ItemToPurchase getItem(Scanner sc) { System.out.print("Enter the item name: "); String name = sc.nextLine(); System.out.print("Enter the item price: "); int price = sc.nextInt(); sc.nextLine(); System.out.print("Enter the item quantity: "); int quantity = sc.nextInt(); sc.nextLine(); System.out.print("Enter the tax category: "); String category = sc.nextLine(); ItemTaxCategory it; if(category.equalsIgnoreCase("X")) { it = ItemTaxCategory.X; }else if(category.equalsIgnoreCase("T")) { it = ItemTaxCategory.T; }else { it = ItemTaxCategory.N; } ItemToPurchase itemToPurchase = new ItemToPurchase(); itemToPurchase.setName(name); itemToPurchase.setPrice(price); itemToPurchase.setQuantity(quantity); itemToPurchase.setTaxCategory(it); return itemToPurchase; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
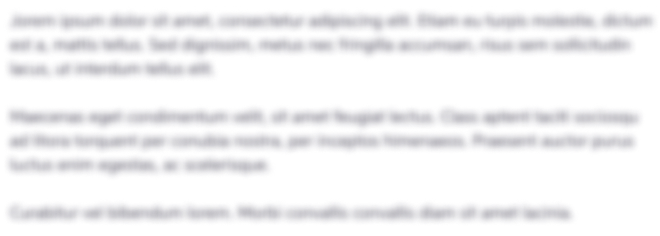
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started