Question
I have this assignment described below. Most of the code is written out, but having trouble getting it to compile, any suggestions? I had to
I have this assignment described below. Most of the code is written out, but having trouble getting it to compile, any suggestions? I had to combine all class and header files into one cpp for submisison, so I don't know if something went wrong with that
Goals:
Learn how to implement a Run-time Polymorphic system by using Shape family.
- Learn how to use Virtual Function and Pure Virtual Function.
- Learn how to use Manager Class to automatically release dynamically allocated memory.
General requirement:
In order to save our time, let us put all classes and main() in one single .cpp file and submit the file. The order of the classes in the file is important. The Shape family classes should be on the top, the Manager Class in the middle, and main at the bottom.
Project requirements:
We purposely minimize the set of the requirements so that you can have more flexibility to design and implement the system.
shaperecords.txt // Please save these contents as shaperecords.txt txt file on your computer..
C 1001 30 -1 R 1002 14 10 S 1003 30 20 Q 1004 28 -1 S 1005 30 75
- As shown in the attached document, the input file, named shaperecords.txt, provides the information of five Shape records. The four columns are listed in the following table.
Column# | Name | Description |
#1 | Shape type | C Circle; R Rectangle, excluding Square; S Spray; Q - Square. |
#2 | Serial number | |
#3 | Radius, width, or side | C or S Radius; R Width; Q Side. |
#4 | Dummy, density or height | C Dummy; S Density; R Height; Q Dummy. |
- The Manager Class must own the array of Shape pointers, which is initialized to nullptr. The Constructor function of the Manager class calls a function named populateShapeData() to load the Shape record data into the array. (You can assume that we may soon have up to 99 Shape records and you can initialize the size of the array to 99.)
- The Manager Class must own a function named displayAll(), which draws the shapes all the Shape objects in the array.
- The Manager Class is also responsible for releasing dynamically allocated memory.
- The following is what the main() might look like.
int main() { mgrShape ms; ms.displayAll(); return 0; } |
#include
using namespace std;
#ifndef RECTANGLE_H #define RECTANGLE_H
class Rectangle : public Shape { protected: int width; int height; public: virtual void setDataOne(int); virtual int getDataOne(); virtual void setDataTwo(int); virtual int getDataTwo(); virtual void draw(); };
#endif // Rectangle_H
///circle class
#ifndef CIRCLE_H #define CIRCLE_H
class Circle : public Shape { protected: int radius; int dummy; public: virtual void setDataOne(int); virtual int getDataOne(); virtual void setDataTwo(int); virtual int getDataTwo(); virtual void draw(); };
#endif // Circle_H
///square class
#ifndef SQUARE_H #define SQUARE_H
class Square : public Rectangle { private: int side; int dummy; public: void setDataOne(int); int getDataOne(); void setDataTwo(int); int getDataTwo(); void draw(); };
#endif // Square_H
/// spray class
#ifndef SPRAY_H #define SPRAY_H
class Spray : public Circle { private: int density; public: void setDataTwo(int); int getDataTwo(); void draw(); };
#endif // Square_H
#ifndef SHAPE_H #define SHAPE_H
#include
using namespace std;
class Shape { protected: int serialNumber = 0; public: void setSerialNumber(int); int getSerialNumber() const;
virtual void setDataOne(int) = 0; virtual int getDataOne() = 0; virtual void setDataTwo(int) = 0; virtual int getDataTwo() = 0; virtual void draw() = 0; };
#endif // Shape_H
//shape mng class
#ifndef SHAPEMGR_H #define SHAPEMGR_H
const int SIZE = 99;
#ifndef SHAPE_H #define SHAPE_H
class ShapeMgr { protected: Shape* arrShapes[SIZE] = { nullptr }; int recordCount = 0; public: ShapeMgr(); ~ShapeMgr(); void populateShapeData(); void displayAll(); };
#endif // ShapeMgr_H
/* Implementation of Square */
void Square::setDataOne(int x) { side = x; }
int Square::getDataOne() { return side; }
void Square::setDataTwo(int x) { dummy = x; }
int Square::getDataTwo() { return dummy; }
void Square::draw() { cout << "-----Square object-----" << endl << endl; cout << " Serial number: " << serialNumber << endl; cout << " Side: " << side << endl << endl; }
/* Implementation of Spray */
void Spray::setDataTwo(int x) { density = x; }
int Spray::getDataTwo() { return density; }
void Spray::draw() { cout << "-----Spray object-----" << endl << endl; cout << " Serial number: " << serialNumber << endl; cout << " Radius: " << radius << endl; cout << " Density: " << density << endl << endl; } /* Implementation of Shape */
void Shape::setSerialNumber(int x) { serialNumber = x; }
int Shape::getSerialNumber() const { return serialNumber; }
/* Implementation of Rectangle */
void Rectangle::setDataOne(int x) { width = x; }
int Rectangle::getDataOne() { return width; }
void Rectangle::setDataTwo(int x) { height = x; }
int Rectangle::getDataTwo() { return height; }
void Rectangle::draw() { cout << "-----Rectangle object-----" << endl << endl; cout << " Serial number: " << serialNumber << endl; cout << " Width: " << width << endl; cout << " Height: " << height << endl << endl;
/* Implementation of Circle */
void Circle::setDataOne(int x) { radius = x; }
int Circle::getDataOne() { return radius; }
void Circle::setDataTwo(int x) { dummy = x; }
int Circle::getDataTwo() { return dummy; }
void Circle::draw() { cout << "-----Circle object-----" << endl << endl; cout << " Serial number: " << serialNumber << endl; cout << " Radius: " << radius << endl << endl; }
//constructor ShapeMgr::ShapeMgr() { populateShapeData(); }
//destructor ShapeMgr::~ShapeMgr() { for (int i = 0; i < SIZE; i++) { if (arrShapes[i] != nullptr) { arrShapes[i]; } } }
//create shapes void ShapeMgr::populateShapeData() { //input file data fstream inputFile; string fileName = "shaperecords.txt"; string line, temp; char shapType; inputFile.open(fileName);
//if shape bool validShape;
while (getline(inputFile, line)) { //turn line into stringstream to separate each piece of data stringstream stream(line);
//take first piece (type of shape) as temp stream >> temp; shapeType = temp[0];
//create shape bool validShape = true; if (shapeType == 'R') arrShapes[recordCount] = new Rectangle; else if (shapeType == 'Q') arrShapes[recordCount] = new Square; else if (shapeType == 'C') arrShapes[recordCount] = new Circle; else if (shapeType == 'S') arrShapes[recordCount] = new Spray; else { cout << "Shape does not exist" << endl << endl; validShape = false; }
//If shhape is valid, fill in setSerialNumber, dateOne, and dataTwo if (validShape) { stream >> temp; arrShapes[recordCount]->setSerialNumber(stoi(temp));
stream >> temp; arrShapes[recordCount]->setDataOne(stoi(temp));
stream >> temp; arrShapes[recordCount]->setDataTwo(stoi(temp)); }
//increment count of shapes recordCount++; }
//close file inputFile.close(); }
//display shapes void ShapeMgr::displayAll() { cout << endl; for (int i = 0; i < recordCount; i++) { //because of polymorphism, each shape has a different draw() function arrShapes[i]->draw(); } }
int main() { ShapeMgr ms; ms.displayAll(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
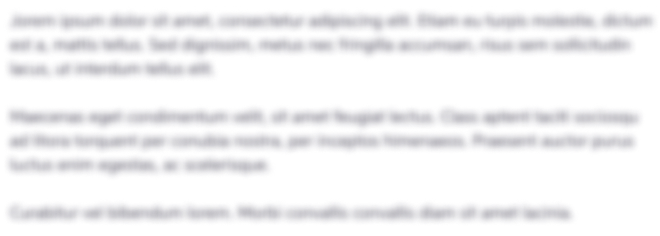
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started