Question
I have this code for a maze but I want to modify it by showing some statistics at the end of the game import random
I have this code for a maze but I want to modify it by showing some statistics at the end of the game
import random
from typing import List, Tuple, Optional
def make_grid(w: int, h: int, player_coord: Tuple[int, int],
gold_coord: Tuple[int, int]) -> List[List[str]]:
grid = [['(_)' for i in range(w)] for j in range(h)]
x, y = player_coord
grid[y][x] = '(x)'
x, y = gold_coord
grid[y][x] = '(o)'
return grid
def print_grid(grid: List[List[str]]) -> None:
s = ''
for row in range(len(grid)):
s += ''.join(element for element in grid[row]) + " "
print(s)
def update_grid(grid: List[List[str]], w: int, h: int, px: int, py: int,
dx: int, dy: int) -> Optional[Tuple[int, int]]:
if (px + dx < 0) or (px + dx >= w) or (py + dy < 0) or (py + dy >= h):
return None
grid[py][px] = '(_)'
grid[py+dy][px+dx] = '(x)'
return (px+dx, py+dy)
def get_moves(d: str) -> Tuple[int, int]:
if d == "N":
return 0, -1
elif d == "S":
return 0, 1
elif d == "E":
return 1, 0
else:
return -1, 0
def get_direction() -> str:
d = input("Which way would you like to move? Choose N, S, E, W. ")
while (len(d) != 1) or (d.upper() not in 'NSEW'):
d = input("Invalid input. Choose N, S, E, W. ")
return d.upper()
# ==== Finish the functions above according to their docstrings ==== #
# ==== The program starts here. ==== #
# ==== Do NOT change anything below this line. ==== #
if __name__ == "__main__":
w = int(input("Width: "))
h = int(input("Height: "))
p_x = p_y = 0
gold_x = random.randint(1, w - 1)
gold_y = random.randint(1, h - 1)
game_won = False
grid = make_grid(w, h, (p_x, p_y), (gold_x, gold_y))
while not game_won:
print_grid(grid)
d = get_direction()
# the following line unpacks the tuple returned by get_moves
# this means the first element in the returned tuple gets assigned
# to dx, the second element in the tuple assigned to dy
dx, dy = get_moves(d)
new_xy = update_grid(grid, w, h, p_x, p_y, dx, dy)
if new_xy:
p_x, p_y = new_xy
game_won = (p_x == gold_x) and (p_y == gold_y)
print("Congratulations! You won.")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
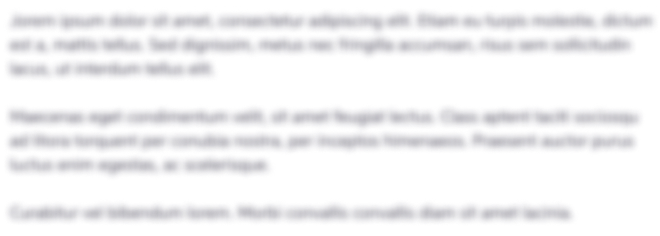
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started