Question
I have this error occuring trying to run this program that is fairly large. the error says no prop file for end of road but
I have this error occuring trying to run this program that is fairly large. the error says no prop file for end of road but in screenshot I have it shown with error. Can someone help me fix this.
Room Directory class:
import java.util.ArrayList;
import edu.waketech.ccave.location.Bathroom;
import edu.waketech.ccave.location.Bedroom;
import edu.waketech.ccave.location.CCaveRoom;
import edu.waketech.ccave.location.Dining;
import edu.waketech.ccave.location.EndOfRoad;
import edu.waketech.ccave.location.Garage;
import edu.waketech.ccave.location.Garden;
import edu.waketech.ccave.location.Kitchen;
import edu.waketech.ccave.location.Tunnels;
import edu.waketech.ccave.location.Washroom;
import edu.waketech.ccave.location.Wellhouse;
public class RoomDirectory {
private ArrayList
private static RoomDirectory instance = new RoomDirectory();
private final CCaveRoom[] ALL_ITEMS = {
new Bathroom(),
new Bedroom(),
new CCaveRoom(),
new Dining(),
new EndOfRoad(),
new Garage(),
new Garden(),
new Kitchen(),
new Tunnels(),
new Washroom(),
new Wellhouse(),
};
private RoomDirectory() {
for (CCaveRoom cci: ALL_ITEMS) {
caveRoom.add(cci);
}
}
public static RoomDirectory getInstance() {
return instance;
}
public boolean contains(java.lang.String id) {
for (CCaveRoom ele : caveRoom) {
if (((CCaveElement) ele).getId().equalsIgnoreCase(id))
return true;
}
return false;
}
public int indexOf(java.lang.String id) {
int i = 0;
for (i = 0; i
if (((CCaveElement) caveRoom.get(i)).getId().equalsIgnoreCase(id))
return i;
}
return -1;
}
public boolean remove(java.lang.String id) {
int i = -1;
boolean found = false;
while (!found) {
i++;
if (((CCaveElement) caveRoom.get(i)).getId().equalsIgnoreCase(id))
found = true;
}
if (i
caveRoom.remove(i);
return true;
}
return false;
}
public CCaveRoom get(java.lang.String id) {
int i = indexOf(id);
if (i == -1) return null;
return caveRoom.get(i);
}
}
Spelunker class:
import java.util.ArrayList;
import edu.waketech.ccave.common.CCaveElement;
import edu.waketech.ccave.common.Direction;
import edu.waketech.ccave.common.ItemDirectory;
import edu.waketech.ccave.common.RoomDirectory;
import edu.waketech.ccave.item.CCaveItem;
import edu.waketech.ccave.location.CCaveRoom;
/**
* Our fearless spelunker.
*
* We track the inventory of Items that we're carrying by assigning them the
* location SPELUNKER_ID. It's not really a "CCaveRoom," but it is a unique
* String and can let us identify the items we're carrying versus the items that
* we're not.
*
*
* If we were doing a Model-View-Controller design pattern for this game (and
* we're close, but not quite there), this would be our controller. The types
* associated with Rooms and Items would be our data model.
*
* @author parks
*
*/
public class Spelunker implements CCaveElement {
/**
*
*/
private static final long serialVersionUID = 1786845014557682582L;
public static final String SPELUNKER_ID = "spelunker";
public RoomDirectory roomDirec = RoomDirectory.getInstance();
private CCaveRoom currentLocation;
/**
* Create a spelunker with a given name and starting location.
*
* @param startRoom
* Were We Start
*/
public Spelunker(String startRoom) {
currentLocation = roomDirec.get(startRoom);
}
/**
* Assign the spelunker to the given location
*
* @param location will become the current location of the spelunker
*/
public void setLocation(CCaveRoom location) {
this.currentLocation = location;
}
/**
* Move the player from the current location in the given direction. Update our
* state as appropriate.
*
*
* @param direction
* Direction to move
*/
public void move(CCaveRoom direction) {
currentLocation = currentLocation.nextRoom(direction);
}
/**
* Take some action on an inventory item--pick it up, drop it, etc.
*
* Dropped items are assigned to be in our current location. Items that are
* picked up are assigned the location SPELUNKER_ID.
*
* @param whatToDo
* the command to perform, like GET or DROP
* @param objectOfAction
* the item to pick up or drop or do whatever with
*/
public void changeInventory(ItemCommand whatToDo, String objectOfAction) {
ItemDirectory itemDirec = ItemDirectory.getInstance();
if (whatToDo == ItemCommand.GET) {
CCaveItem itemObj = itemDirec.get(objectOfAction);
if (itemObj != null)
itemObj.setLocation(SPELUNKER_ID);
;
} else if (whatToDo == ItemCommand.DROP) {
CCaveItem itemObj = itemDirec.get(objectOfAction);
if (itemObj != null) {
itemObj.setLocation(currentLocation.getId());
}
}
}
// NOT I18N CAPABLE
@Override
public String getLongDescription() {
String cRoom = currentLocation.getLongDescription();
String roomInventory = currentLocation.getContentsLongDescription();
StringBuilder youHave = new StringBuilder();
youHave.append("you have ");
ItemDirectory direc = ItemDirectory.getInstance();
ArrayList
for (CCaveItem ele : contents) {
youHave.append(ele.getLongDescription());
youHave.append(" ");
}
return "You are in " + cRoom + " In here is" + roomInventory + " and you have " + youHave.toString();
}
// NOT I18N CAPABLE
@Override
public String getShortDescription() {
String cRoom = currentLocation.getShortDescription();
StringBuilder youHave = new StringBuilder();
youHave.append("you have ");
ItemDirectory direc = ItemDirectory.getInstance();
ArrayList
for (CCaveItem ele : contents) {
youHave.append(ele.getShortDescription());
}
return "You are in " + cRoom + " and has " + youHave;
}
@Override
public String getId() {
return SPELUNKER_ID;
}
@Override
public boolean isId(String identifier) {
return SPELUNKER_ID.equalsIgnoreCase(identifier);
}
/**
* If we get a command that's classified as "OTHER," we find the item being
* manipulated and rely on it to do whatever specific processing is necessary.
*
* @param cmd
* The command to be executed
*
* @param item
* the item being manipulated
*/
public void otherCommand(ItemCommand cmd, String item) {
// if we can identify the item, we'll operate on it and it alone
CCaveItem caveItem = ItemDirectory.getInstance().get(item);
if (caveItem != null) {
caveItem.executeCommand(cmd, item, currentLocation);
}
}
public Direction move(Direction dir) {
// TODO Auto-generated method stub
return dir;
}
}
Cave test Main Class:
package edu.waketech.ccave.provided;
import java.io.IOException; import edu.waketech.ccave.common.Direction;
public class CaveTestMain {
public static void main(String[] args) throws IOException {
// First some sanity checks.
// CCaveMap caveMap = CCaveMap.accessMap();
Spelunker me = new Spelunker("endofroad");
System.out.println(me.getLongDescription());
me.move(Direction.S);
System.out.println(me.getLongDescription());
me.move(Direction.N);
System.out.println(me.getLongDescription());
me.move(Direction.E);
System.out.println(me.getLongDescription());
}
}
Patkage Explurer Task List JUnit 5 resources 1 lonEdescription -End of road 2 shortdescription - cnod 3 nwnend of road Find AllActivato M bedroomproperties dirt propertics dcr.preg:erties down.properties Outline An outline is nat awailable R e properties ??fire.properties food.propertes garag.properties teminateds CaveTestMain Jave Application) CProaram FilesJavaire1.8.0.161 binjavaw.axe (Apr 28 2018,1235:31 AM) no prop file for end of road Exception in thread "main" iava.lang.ClassCastException: edu.waketech.ccave.location.Bathroon cannot be cast to edu.waketech.ccave.common.CCavetlenent lamp.propertes ne.properties M mw.properties at edu.waketech.ccave.comnonRoomVirectory.indexof RoonDirectory.java: B) at edu.waketech.ccave.comDirectory.get RoonDirectory.iava:182) at edu.waketech.ccave.provided.Spelunker.Step by Step Solution
There are 3 Steps involved in it
Step: 1
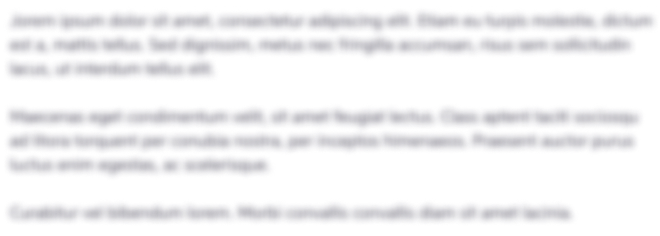
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started