Question
I have to complete a java program I already have part of the program (down below) I just have to fill it in Your Task
I have to complete a java program
I already have part of the program (down below) I just have to fill it in
Your Task Complete the Java code in Assignment2.java to get the following output
--------------------------Rectangle--------------------------
I am the RegularGeometricShape constructor with no parameters I am the Quadrilateral constructor with five parameters Rectangle side number: 4 Rectangle length = 2.0 Rectangle width = 1.0 Rectangle center = (1.00,0.50) Rectangle area = 2.0 The perimeter of the Rectangle = 6.0 The points p1(0.00,1.00), p2(2.00,1.00), p3(2.00,0.00), p4(0.00,0.00) form a rectangle
-------------------------------------------------------------
--------------------------Square------------------------------
I am the constructor of RegularGeometricShape without parameter I am the constructor of Quadrilateral with five parameters Number of sides of the Square: 4 Length of the Square = 1.0 The center of the Square = (0.50,0.50) The points p1, p2, p3, p4 form a edge
-------------------------------------------------------------
--------------------------Circle-----------------------------
I am the constructor of RegularGeometricShape without parameter Number of sides of the Circle: 0 The center of the Circle = (1.00,2.00) The surface of the Circle = 3.14 The perimeter of the Circle = 6.28
-------------------------------------------------------------
--------------------------Triangle---------------------------
I am the constructor of RegularGeometricShape without parameter Number side of the Triangle: 3 The center of the Triangle = (0.33,0.33) The perimeter of the Triangle = 3.414213562373095 The area of the Triangle = 0.5 Isosceles Triangle The triangle is not Equilateral Triangle Rectangle
import java.lang.Math;
class Point {
Point() {
this.x = 0;
this.y = 0;
}
Point(double x, double y) {
}
public double distance(Point p){
}
public double getX() {
}
public double getY() {
}
public void setX (double x) {
}
public void setY (double y) {
}
public String toString() {
return "(" + String.format("%.2f",this.x) + "," + String.format("%.2f",this.y) + ")";
}
}
interface Forme {
public Point getCentre();
public int getNombreCotes();
public Point [] getSommets();
public double perimetre();
public double surface();
public String nomDeLaForme();
public void dessiner(); //optionnel
}
abstract class FormeGeometriqueReguliere implements Forme{
FormeGeometriqueReguliere() {
System.out.println("Je suis le constructeur de FormeGeometriqueReguliere sans parametre");
}
public Point getCentre(){
}
public int getNombreCotes() {
}
public Point [] getSommets() {
}
public static void afficherNomDeLaForme() {
}
public String nomDeLaForme(){
}
}
abstract class Quadrilatere extends FormeGeometriqueReguliere{
Quadrilatere() {
System.out.println("Je suis le constructeur de Quadrilatere sans parametre");
centre = new Point(0,0);
}
Quadrilatere(Point p1, Point p2, Point p3, Point p4, Point centre) {
System.out.println("Je suis le constructeur de Quadrilatere avec cinq parametres");
}
public String nomDeLaForme(){ //Overriding method
}
public int getNombreCotes() {
}
public boolean isRectangle() {
//Il suffit de verifier que si P1P2 == P3P4 et P1P4 == P2P3
}
public boolean isCarre() {
//Si rectangle et P1P2 == P2P3
}
}
class Rectangle extends Quadrilatere{
public static void afficherNomDeLaForme() { //Overriding method
}
public String nomDeLaForme(){
}
private static Point calculerCentre(Point p1, Point p2, Point p3, Point p4) {
}
Rectangle (Point p1, Point p2, Point p3, Point p4) {
}
public double perimetre() {
}
public double surface() {
}
public double getLongueur() {
}
public double getLargeur() {
}
public void dessiner(){
//optionnel
}
}
class Carre extends Rectangle{
public String nomDeLaForme(){ //Overriding method
}
Carre (Point p1, Point p2, Point p3, Point p4) {
}
Carre (Point p1, Point p3) {
//optionnel
}
}
class Cercle extends FormeGeometriqueReguliere{
public String nomDeLaForme(){ //Overriding method
}
Cercle (Point centre, double rayon) {
}
public int getNombreCotes() {
}
public double perimetre() {
}
public double surface() {
}
public void dessiner() {
//Optionnel
}
}
class Triangle extends FormeGeometriqueReguliere{
public static void afficherNomDeLaForme() { //Overriding method
}
public String nomDeLaForme(){
}
private static Point calculerCentre(Point p1, Point p2, Point p3) {
}
Triangle (Point p1, Point p2, Point p3) {
}
public int getNombreCotes() {
}
public double perimetre() {
}
public double surface() {
}
public void dessiner(){
//Optionnel
}
public boolean isRectanle() {
}
public boolean isIsocele() {
}
public boolean isEquilaterale() {
}
}
public class Devoir2
{
public static void main(String[] args) {
//Rectangle
System.out.println("--------------------------Rectangle--------------------------");
Rectangle rect1 = new Rectangle(new Point(0,1),new Point(2,1), new Point(2,0),new Point(0,0));
System.out.println("Nombre de cote du " + rect1.nomDeLaForme() + " : " + rect1.getNombreCotes());
System.out.println("Longueur du " + rect1.nomDeLaForme() + " = " + rect1.getLongueur());
System.out.println("Largeur du " + rect1.nomDeLaForme() + " = " + rect1.getLargeur());
System.out.println("Le centre du " + rect1.nomDeLaForme() + " = " + rect1.getCentre());
System.out.println("La surface du " + rect1.nomDeLaForme() + " = " + rect1.surface());
System.out.println("Le perimetre du " + rect1.nomDeLaForme() + " = " + rect1.perimetre());
Point[] sommets = rect1.getSommets();
if (rect1.isRectangle()) {
System.out.println("Les points p1" + sommets[0] + ", p2" + sommets[1] + ", p3" + sommets[2] + ", p4" + sommets[3] + " forment un rectangle");
} else {
System.out.println("Les points p1" + sommets[0] + ", p2" + sommets[1] + ", p3" + sommets[2] + ", p4" + sommets[3] + " ne forment pas un rectangle");
}
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Carre------------------------------");
//Carre
Carre c1 = new Carre(new Point(0,1), new Point(1,1), new Point(1,0), new Point(0,0));
System.out.println("Nombre de cote du " + c1.nomDeLaForme() + " : " + c1.nbre_cotes);
System.out.println("Longueur du " + c1.nomDeLaForme() + " = " + c1.getLongueur());
System.out.println("Le centre du " + c1.nomDeLaForme() + " = " + c1.getCentre());
if (c1.isCarre()) {
System.out.println("Les points p1, p2, p3, p4 forment un carre");
} else {
System.out.println("Les points p1, p2, p3, p4 ne forment pas un carre");
}
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Cercle-----------------------------");
//Cercle
Cercle cercle1 = new Cercle(new Point(1,2) , 1);
System.out.println("Nombre de cote du " + cercle1.nomDeLaForme() + " : " + cercle1.getNombreCotes());
System.out.println("Le centre du " + cercle1.nomDeLaForme() + " = " + cercle1.getCentre());
System.out.println("La surface du " + cercle1.nomDeLaForme() + " = " + String.format("%.2f",cercle1.surface()));
System.out.println("Le perimetre du " + cercle1.nomDeLaForme() + " = " + String.format("%.2f",cercle1.perimetre()));
System.out.println("-------------------------------------------------------------");
System.out.println("--------------------------Triangle---------------------------");
//Triangle
Triangle triangle1 = new Triangle(new Point(0,0),new Point(1,0), new Point(0,1));
System.out.println("Nombre cote du " + triangle1.nomDeLaForme() + " : " + triangle1.getNombreCotes());
System.out.println("Le centre du " + triangle1.nomDeLaForme() + " = " + triangle1.getCentre());
System.out.println("Le perimetre du " + triangle1.nomDeLaForme() + " = " + triangle1.perimetre());
System.out.println("La surface du " + triangle1.nomDeLaForme() + " = " + triangle1.surface());
if (triangle1.isIsocele()) {
System.out.println("Triangle Isocele");
} else {
System.out.println("Le triangle n'est pas Isocele");
}
if (triangle1.isEquilaterale()) {
System.out.println("Triangle Equilaterale");
} else {
System.out.println("Le triangle n'est pas Equilaterale");
}
if (triangle1.isRectangle()) {
System.out.println("Triangle Rectangle");
} else {
System.out.println("Le triangle n'est pas Rectangle");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
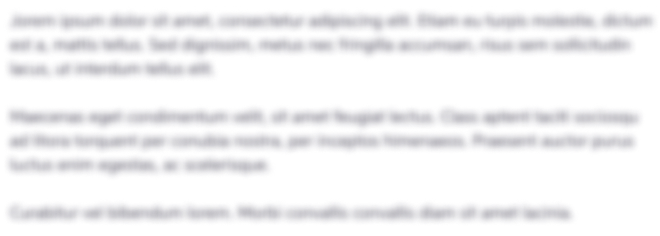
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started