Question
I have to convert this ArrayIntStack class to an ArrayCellStack Class. Here is the cell class: #include Cell.h Cell::Cell() { _symbol = ' '; _isStart
I have to convert this ArrayIntStack class to an ArrayCellStack Class.
Here is the cell class:
#include "Cell.h"
Cell::Cell() { _symbol = ' '; _isStart = false; _isEnd = false; _x = 0; _y = 0; }
Cell::Cell(char c, int x, int y) { _symbol = c; _x = x; _y = y; _isStart = (_symbol == 'S'); _isEnd = (_symbol == 'E'); }
Cell::Cell(const Cell& obj) { _symbol = obj._symbol; _isStart = obj._isStart; _isEnd = obj._isEnd; _x = obj._x; _y = obj._y; }
Cell::~Cell() { // Nothing special }
char Cell::getSymbol() { return _symbol; }
void Cell::setSymbol(char c) { _symbol = c; }
bool Cell::isStart() { return _isStart; }
bool Cell::isEnd() { return _isEnd; }
int Cell::getX() { return _x; }
int Cell::getY() { return _y; }
Here is the ArrayIntStack class:
#include "ArrayIntStack.h"
ArrayIntStack::ArrayIntStack() { _max = 10; _top = 0; _stack = new int*[_max]; }
ArrayIntStack::ArrayIntStack(int size) { _max = size; _top = 0; _stack = new int*[_max]; }
ArrayIntStack::ArrayIntStack(const ArrayIntStack & obj) { this->_max = obj._max; this->_top = obj._top;
this->_stack = new int*[_max]; // Copy over the contents of the stack // being sure make COPIES of the contents for (int i = 0; i < obj._top; i++) { // Make the new stack's new thing's pointer point // to the thing that he other stack's thing's pointer // pointed to this->_stack[i] = obj._stack[i]; } }
ArrayIntStack::~ArrayIntStack() {
// Delete the container itself delete[] _stack; }
void ArrayIntStack::expandCapacity() { // Double the size _max = _max * 2;
// Make a new array int** newArray = new int*[_max];
// Copy over the pointers from the old stack for (int i = 0; i < _top; i++) { newArray[i] = _stack[i]; }
// Delete the old array delete[] _stack;
// Make the class attribute point // to the new array we just made _stack = newArray; }
void ArrayIntStack::push(int * toPush) { // If we are out of space in the array // we will automatically expand the capacity if (_top == _max) { expandCapacity(); }
// Put the pointer on the top of the stack _stack[_top] = toPush;
// Increase the _top _top++; }
int* ArrayIntStack::pop() { if (is_empty()) { // Works on Windows (and is better) //throw std::exception("Stack is Empty"); // Works everywhere throw std::exception(); }
// No need to change what _stack[_top] points to // since _top knows that that spot is free to use _top--; return _stack[_top]; }
int* ArrayIntStack::peek() { if (is_empty()) { // Works on Windows (and is better) //throw std::exception("Stack is Empty"); // Works everywhere throw std::exception(); }
// Return the thing, but leave _top alone return _stack[_top-1]; }
int ArrayIntStack::size() { return _top; }
bool ArrayIntStack::is_empty() { // If top is 0, it's empty return _top == 0; }
std::string ArrayIntStack::to_string() { std::string s = "";
for (int i = 0; i < _top; i++) { s = s + std::to_string(*_stack[i]) + ", "; } s = s + " <-- Top"; return s; }
Here is what I have done to that class:
#include "ArrayCellStack.h" #include "Cell.h"
ArrayCellStack::ArrayCellStack() { _max = 10; _top = 0; _stack = new Cell* [_max]; }
ArrayCellStack::ArrayCellStack(int size) { _max = size; _top = 0; _stack = new Cell* [_max]; }
ArrayCellStack::ArrayCellStack(const ArrayCellStack& obj) { this->_max = obj._max; this->_top = obj._top;
this->_stack = new Cell* [_max];
// Copy over the contents of the stack // being sure make COPIES of the contents for (int i = 0; i < obj._top; i++) { // Make the new stack's new thing's pointer point // to the thing that he other stack's thing's pointer // pointed to this->_stack[i] = obj._stack[i]; } }
ArrayCellStack::~ArrayCellStack() {
// Delete the container itself delete[] _stack; }
void ArrayCellStack::expandCapacity() { // Double the size _max = _max * 2;
// Make a new array Cell** newArray = new Cell* [_max];
// Copy over the pointers from the old stack for (int i = 0; i < _top; i++) { newArray[i] = _stack[i]; }
// Delete the old array delete[] _stack;
// Make the class attribute point // to the new array we just made _stack = newArray; }
void ArrayCellStack::push(Cell* toPush) { // If we are out of space in the array // we will automatically expand the capacity if (_top == _max) { expandCapacity(); }
// Put the pointer on the top of the stack _stack[_top] = toPush;
// Increase the _top _top++; }
Cell* ArrayCellStack::pop() { if (is_empty()) { // Works on Windows (and is better) //throw std::exception("Stack is Empty"); // Works everywhere throw std::exception(); }
// No need to change what _stack[_top] points to // since _top knows that that spot is free to use _top--; return _stack[_top]; }
Cell* ArrayCellStack::peek() { if (is_empty()) { // Works on Windows (and is better) //throw std::exception("Stack is Empty"); // Works everywhere throw std::exception(); }
// Return the thing, but leave _top alone return _stack[_top - 1]; }
int ArrayCellStack::size() { return _top; }
bool ArrayCellStack::is_empty() { // If top is 0, it's empty return _top == 0; }
std::string ArrayCellStack::to_string() { std::string s = "";
for (int i = 0; i < _top; i++) { s = s + *_stack[i] + ", "; **** My issue is with this line, converting Cell to string ********* } s = s + " <-- Top"; return s; }
Any help would be great. Thanks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
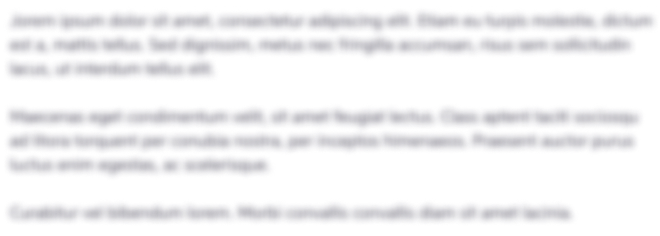
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started