Question
I have to count the number of duplicate fractions from a text file. For example, if the text file contatins the fractions 6/3, 4/2, 2/1
I have to count the number of duplicate fractions from a text file. For example, if the text file contatins the fractions 6/3, 4/2, 2/1 and 5/7, the output should return 2/1 = 3 times, 5/7 = 1 time. I did write a program but it prints all the numbers the number of times it repeats. How do I fix it and make it only return once?
CODE:
public class Fraction{
public static void main(String [] args){
//Reads file containing fraction Scanner input = null; try { input = new Scanner(new FileInputStream("fractions.txt")); } catch (FileNotFoundException e) { System.out.println("File not found."); System.exit(0); }
//variables String[] fraction = new String[100]; //will take in the fraction String[] split = new String[2]; //used to split the fraction int[] num = new int[100]; //store nums int[] den = new int[100]; //store dens int numOfLines = 0; //number of lines in file int count = 0; //number of fraction that are the duplicate
//the number of lines in the file, put each line into the string[] fraction for (int i = 0; input.hasNextLine(); i++){ fraction[i]=input.nextLine(); numOfLines++; }
//split the fraction[] into two arrays: num and den for(int i = 0; i < numOfLines; i++){ split = fraction[i].split("/"); num[i] = Integer.parseInt(split[0]); den[i] = Integer.parseInt(split[1]); } //used to compare specific num and den to the rest of the numbers double num1; double den1; double[] result = new double[100];
//start off by comparing den, and then compares the num //of like dens for(int i = 0; i < numOfLines; i++){ try{ den1 = den[i]; num1 = num[i]; result[i]= num1/den1;
} catch (ArithmeticException e){ System.out.println("Sorry cant divide by 0."); System.exit(0); } }
int min = 0; int max = 0; for(int i = 0; i < numOfLines; i++){
for ( int j = 0; j< numOfLines; j++){
if (result[i] == result [j]){ count++;
min = num[i]; max = den[i]; if ( num[j] < min){ min = num[j]; max = den[j]; } }
}
System.out.println(min + "/" + max + " " + count + " " + result[i] ); count = 0;
}
} }
OUTPUT:
2/1 count is 3 2/1 count is 3 5/9 count is 1 80/90 count is 2 800/900 count is 2 15/25 count is 1 2/2 count is 4 1/1 count is 4 1/10 count is 1 1/100 count is 1 1/1000 count is 1 1/3 count is 4 2/6 count is 4 1/2 count is 2 1/3 count is 4 1/1 count is 4 1/4 count is 2 1/5 count is 1 1/6 count is 1 1/7 count is 1 1/8 count is 1 1/9 count is 1 2/1 count is 3 2/2 count is 4 2/3 count is 1 2/4 count is 2 2/5 count is 1 2/6 count is 4 2/7 count is 1 2/8 count is 2 2/9 count is 1
TEXTFILE:
6/3 4/2 5/9 80/90 800/900 15/25 5/5 1/1 1/10 1/100 1/1000 1/3 2/6 1/2 1/3 1/1 1/4 1/5 1/6 1/7 1/8 1/9 2/1 2/2 2/3 2/4 2/5 2/6 2/7 2/8 2/9
Step by Step Solution
There are 3 Steps involved in it
Step: 1
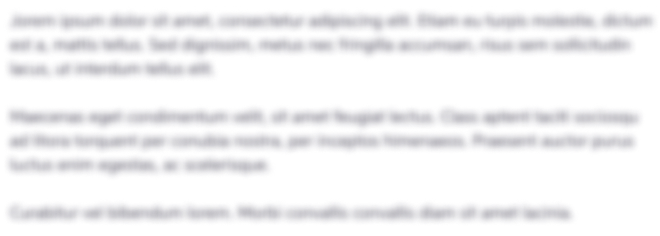
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started