Question
I have to create a playable game of Tic Tac Toe in Notepad++ using Java. I have my code below but for some reason its
I have to create a playable game of Tic Tac Toe in Notepad++ using Java. I have my code below but for some reason its showing 30 errors and I don't see the problem. Can someone please help me troubleshoot!
import java.util.Scanner;
public class TicTacToeTest1 {
public static void main(String[] args) { public static final int Empty = 0; public static final int Cross = 1; public static final int Nought = 2; public static final int Playing = 0; public static final int Draw = 1; public static final int Cross_Win = 2; public static final int Nought_Win = 3; public static final int Rows = 3, Cols = 3; public static int[][] board = new int[Rows][Cols]; public static int currentState; public static int currentPlayer; public static int currentRow, currentCol; public static Scanner in = new Scanner(System.in); public static void main(String[] args) { initGame(); do{ playerMove(currentPlayer); updateGame(currentPlayer, currentRow, currentCol); printBoard(); if (currentState == Cross_Win){ System.out.println("'X' Wins!!! Congrats!"); } else if (currentState == Nought_Win){ System.out.println("'O' Wins!!! Congrats!"); } else if (currentState == Draw){ System.out.println("It's a Draw :("); } currentPlayer = (currentPlayer == Cross) ? Nought : Cross; } while (currentState == Playing); } public static void initGame(){ for (int row = 0; row < Rows; ++row){ for (int row = 0; col < Cols; ++col) { board[row][col] = Empty; } } currentState = Playing; currentPlayer = Cross; } public static void playerMove(int theSeed){ boolean validInput = false; do{ if(theSeed == Cross){ System.out.print("Player 'X', take your turn (row[1-3] column[1-3]): "); } else{ System.out.print("Player 'O', take your turn (row[1-3] column[1-3]): "); } int row = in.nextInt() - 1; int col = in.nextInt() - 1; if (row >= 0 && row < Rows && col >= 0 && col < Cols && board[row][col] == Empty) { currentRow = row; currentCol = col; board[currentRow][currentCol] = theSeed; validInput = true; } else { System.out.println("This move at (" + (row + 1) + "," + (col + 1) + ") is not valid. Try a different move."); } } while (!validInput); } public static void updateGame(int theSeed, int currentRow, int currentCol) { if (hasWon(theSeed, currentRow, currentCol)) ? Cross_Win : Nought_Win; } else if (isDraw()) { currentState = Draw; } }
public static boolean isDraw() { for (int row = 0; row < Rows; ++row) { for (int col = 0; col < Cols; ++col) { if (board[row][col] == Empty) { return false; } } } return true; }
public static boolean hasWon(int theSeed, int currentRow, int currentCol) { return (board[currentRow][0] == theSeed && board[currentRow][1] == theSeed && board[currentRow][2] == theSeed || board[0][currentCol] == theSeed && board[1][currentCol] == theSeed && board[2][currentCol] == theSeed || currentRow == currentCol && board[0][0] == theSeed && board[1][1] == theSeed && board[2][2] == theSeed ||currentRow + currentCol == 2 && board[0][0] == theSeed && board[1][1] == theSeed && board[2][0] == theSeed); }
public static void printBoard() { for (int row = 0; row < Rows; ++row) { for (int col = 0; col < Cols; ++col) { printCell(board[row][col]); if (col != Cols - 1) { System.out.print("|"); } } System.out.println(); if (row != Rows - 1) { System.out.println("-----------"); } } System.out.println(); }
public static void printCell(int content) { switch (content) { case Empty: System.out.print(" "); break; case Nought: System.out.print(" O ") break; case Cross: System.out.print(" X "); break; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
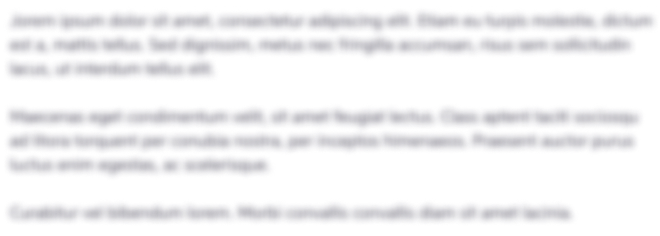
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started