Question
I have to implement a set of classes using inheritance and composition, the classes can be seen below. class person { public : person ();
I have to implement a set of classes using inheritance and composition, the classes can be seen below.
class person
{
public :
person ();
person ( string , string , string );
string getFirst () const ;
string getLast () const ;
string getId () const ;
private :
string first ;
string last ;
string id ;
};
class student
{
public :
static const int CREDITS_NEEDED = 15;
student ();
student ( string , string , string );
person getPersonInfo () const ;
unsigned int getCredits () const ;
double getGpa () const ;
void addCredits ( unsigned int , double );
bool graduated () const ;
private :
person info ;
unsigned int credits ;
double gpa ;
};
1
class undergrad : student
{
public :
undergrad ();
undergrad ( string , string , string );
string getFirst () const ;
string getLast () const ;
unsigned int getCredits () const ;
double getGpa () const ;
bool searchById ( string ) const ;
bool searchByName ( string ) const ;
void completedClass ( double );
bool graduated () const ;
};
class grad : student
{
public :
grad ();
grad ( string , string , string , string );
string getFirst () const ;
string getLast () const ;
unsigned int getCredits () const ;
double getGpa () const ;
bool searchById ( string ) const ;
bool searchByName ( string ) const ;
void completedClass ( double );
bool graduated () const ;
void completedThesis ();
bool hasDefended () const ;
private :
string thesis ;
bool defended ;
};
class phd : student
{
public :
phd ();
phd ( string , string , string , string );
string getFirst () const ;
string getLast () const ;
unsigned int getCredits () const ;
double getGpa () const ;
bool searchById ( string ) const ;
bool searchByName ( string ) const ;
void completedClass ( double );
bool graduated () const ;
void completedDissertation ();
bool hasDefended () const ;
private :
string dissertation ;
bool defended ;
};
As always, you cannot modify the class, you need to implement those functions as instructed and you cannot change the inheritance type, you can have extra helper (non member) functions in main or in the class implementation file, as always please comment your code (as well as the member functions) and use constants rather than literals as needed
Contents of main
In your main, you will need to do the following steps:
1. You will create three arrays (each of length 5, use a global named constant), you will create them
in the following way
undergrad undergradStudents [ SIZE ];
grad gradStudents [ SIZE ];
phd doctoralStudents [ SIZE ];
The array names can vary but you must declare them in that fashion that invokes the default constructor
for each object
2. Open an input filestream, you can hard code the name of the input file in the open function
3. Read the file, there will be 15 lines, and 5 of each kind of student, each line will contain
firstName LastName ID StudentType ThesisOrDissertationTitle
Each will be separated by a space, student type can be U for undergraduate, G for graduate, and P for
doctorate student, then there will be a thesis or dissertation title only if the student type is not U, if
the thesis or dissertation title read in is equal to None then you will set the dissertation or thesis title
to empty string, using the contents read on each line, insert a new object into the appropriate array
in a position of the corresponding array
4. Prompt the user to enter a name (first and last), and ID, or q to quit
5. If the user entered a lower case or upper case q, then quit the program
6. If the user entered an ID from 4, then traverse all three arrays, and call each objects searchById(string)
function until the student with that ID was found, if not found output the error and go back to step
4 otherwise go to step 8
7. If the user entered an name (first and last) from 4, then traverse all three arrays, and call each objects
searchByName(string) function until the student with that name was found, if not found output the
error and go back to step 4 otherwise go to step 8
8. Output the users info (refer to the sample output portion)
(a) If the student has graduated, if the graduated() function returns true then go back to step 4,
else go to the next step
(b) Prompt if the user has completed a class, if the user enters yes (case insensitive) then prompt for
the updated GPA and call the objects completedClass(double) function
(c) If that student is an undergraduate, then go back to step 4
(d) If that student is a graduate or doctoral student, if that student has not defended a dissertation or
thesis, hasDefended() function returns false, prompt the user if they defended their dissertation
or thesis, if the user entered yes (case insensitive), call the objects completedDissertation()
function
(e) Go back to step 4
Example Run
Star , Patrick
GPA : 0.0 Credits : 0
Graduated : No
Has the student completed a course ? ( Yes or No ): yes
Enter updated GPA : 3.4
Enter Student ID or full name or q to quit : 3451
Star , Patrick
GPA : 3.4 Credits : 3
Graduated : No
Has the student completed a course ? ( Yes or No ): yes
Enter updated GPA : 3.6
Enter Student ID or full name or q to quit : patrick star
Star , Patrick
GPA : 3.6 Credits : 6
Graduated : No
Has the student completed a course ? ( Yes or No ): yes
Enter updated GPA : 3.3
Enter Student ID or full name or q to quit : 3451
Star , Patrick
GPA : 3.3 Credits : 9
Graduated : No
Has the student completed a course ? ( Yes or No ): no
Enter Student ID or full name or q to quit : patrick star
Star , Patrick
GPA : 3.3 Credits : 9
Graduated : No
Has the student completed a course ? ( Yes or No ): yes
Enter updated GPA : 3.2
Here is the data used
John Smith 1234 U
Patrick Star 3451 U
Not Sure 1111 P Smartest_Man_In_The_World
Colonel Mustard 2316 P A_Game_Of_Clue
Mad Hatter 8762 P Tea_Time
Rick Sanchez 2468 P None
Timmy Turner 3416 U
Tony Tiger 3461 G None
Stewie Griffin 2989 P Victory_Is_Mine
Bam Margera 5634 G Viva_La_Bam
Glen Quagmire 1023 U
Sid Vicious 3876 G My_Way
Tommy Ramone 2718 G Sheena_Is_A_Punk_Rocker
John Lenon 2671 G Imagine
Kit Kat 9961 U
Step by Step Solution
There are 3 Steps involved in it
Step: 1
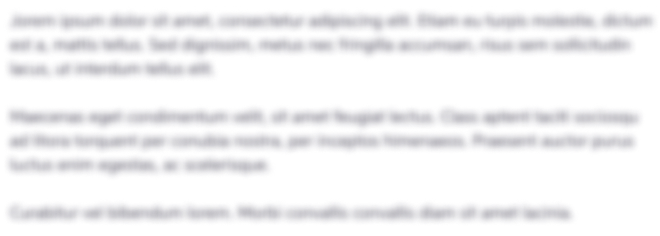
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started