Question
I have two classes one that represents the node in the linked list and the other that implements the linked list. My goal is to
I have two classes one that represents the node in the linked list and the other that implements the linked list. My goal is to read a line from a text file parse through it and assign it to multiple variables. Those variables are part of the node. In main, I call the function enqueue which sends those variables to the enqueue function to the class that implements the linked list. The enqueue function uses if statements that to turn the linked list into a priority queue using comparison statements. When I point head to priority value to compare it to the parameter that has just been passed into the enqueue function I receive a (THREAD 1: EXC_BAD_ACCESS (CODE = 1, ADDRESS 0X10) I have included a screenshot of the debug console as well as part of the code. I apologize ahead of time if I have not fully given enough information since I am a novice. If more information is needed please comment below.
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
using namespace std;
class Node {
public:
Node* next;
int jobID;
int arrival_time;
int priority_value;
int wait_time;
Node(int jd, int pv, int at, int wt);
};
class LinkedList{
public:
Node* head;
Node* firstNode(int jd, int pv, int at, int wt);
int peek(Node** head);
void enqueue(Node **head,int jd, int pv, int at, int wt);
void dequeue(Node **head);
int getLength(Node* head);
bool isEmpty(Node* head);
void display(Node* head);
LinkedList();
};
time_t t = time(0);
tm* now = localtime(&t);
Node::Node(int jd, int pv, int at, int wt){
jobID = jd;
priority_value = pv;
arrival_time = at;
wait_time = wt;
next = nullptr;
}
LinkedList::LinkedList(){
head = nullptr;
}
Node* LinkedList::firstNode(int jd, int pv, int at, int wt){
Node *newNode = new Node(jd, pv, at, wt);
newNode->jobID = jd;
newNode->priority_value = pv;
newNode->arrival_time = at;
newNode->wait_time = wt;
return newNode;
}
int LinkedList::peek(Node** head){
return (*head)->jobID;
}
void LinkedList::dequeue(Node** head){
Node* newNode = *head;
(*head) = (*head)->next;
free(newNode);
}
void LinkedList::enqueue(Node **head, int jd, int pv, int at, int wt){
Node* startNode = (*head);
Node* tempNode = firstNode(jd, pv, at, wt);
if(head == NULL){
Node *newNode = firstNode(jd, pv, at, wt);
}
else if((*head)->priority_value > pv){
tempNode->next = *head;
(*head) = tempNode;
}
else{
while (startNode->next != NULL && startNode->next->priority_value < pv){
startNode = startNode->next;
}
tempNode->next = startNode->next;
startNode->next = tempNode;
}
}
int LinkedList::getLength(Node* head){
Node *list = head;
int count = 0;
while(list){
list = list->next;
count += 1;
}
return count;
}
bool LinkedList::isEmpty(Node* head){
return head == nullptr;
}
void LinkedList::display(Node* head){
ofstream outputFile ("output.txt");
Node* list = head;
outputFile << "Job ID " << setw(10) << "Arrival Time " << setw(10) << "Priority ID " << endl;
while(list){
if (head == nullptr){
isEmpty(list);
}
else{
outputFile << list->jobID << " " << setw(10) << (now->tm_year + 1900) << "-" << (now->tm_mon +1) << "-" << now->tm_mday << setw(5) << list->priority_value << endl;
list = list->next;
}
}
}
int main(){
LinkedList l;
string line;
unsigned int sys_time = 0;
Node *head = NULL;
string date;
int job_ID;
int priority_value;
int arrival_time;
int processing_time;
fstream myfile ("myfile.txt");
ofstream outputFile ("output.txt");
while(getline(myfile, line)){
replace(line.begin(), line.end(), ',', ' ');
stringstream ss(line);
ss >> job_ID;
ss >> priority_value;
ss >> arrival_time;
ss >> processing_time;
l.enqueue(&head, job_ID, priority_value, arrival_time, processing_time);
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
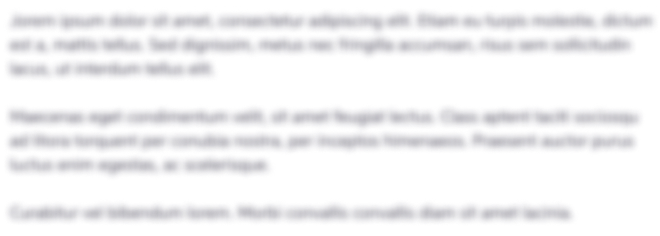
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started