Question
I have written a program (see code below) that let the user enter any number of non-negative integers in a sequence and save them in
I have written a program (see code below) that let the user enter any number of non-negative integers in a sequence and save them in an array. The numbers should be between 0 1000. The sequence ends when the user enters a negative number, or when 100 numbers have been entered. Numbers larger than 1000 are ignored (and not stored in the array).
The program calculates which of the entered integers that occurs the most frequently in the array, and also how many times that number occurs in the array
Example run 1 (user input in bold, comments in italic):
Please enter numbers between 0 and 1000 (max 100 numbers). End with a negative number.
Number: 1 Number: 2 Number: 3 Number: 568 Number: 2 Number: 2 Number: 3 Number: -1
The number 2 occurs the most times, a total of 3 times.
Example run 2:
Please enter numbers between 0 and 1000 (max 100 numbers). End with a negative number.
Number: 1200 remember that numbers above 1000 are ignored Number: 4 Number: 1200 Number: 1200 Number: 4 Number: -5
The number 4 occurs the most times, a total of 2 times.
4 occurs most times because the 1200 were never saved in the array since they are above 1000
My question is: How can I modify the program to calculate which number occurs least frequently and also prints how many times that number was entered (could this be done using an array in case the user enters several numbers that are the least frequent number)?: example run below:
Please enter numbers between 0 and 1000 (max 100 numbers). End with a negative number.
Number: 800 remember that numbers above 1000 are ignored Number: 4 Number: 3 Number: 2 Number: 800 Number: -5
The number 800 occurs the most times, a total of 2 times.The numbers 4,3,2 occurs least times, a total of 1 time.
My code:
#include
int main(void) { int numbers[size]; int mostFrequent; int numberOfOccurences; int ind, i = 0; printf("Please enter numbers between 0 and 1000 (max %d numbers). End with a negative number.", size); for (ind = 0; ind < size; ind++) { int num; printf(" Number: "); scanf_s("%d", &num); if (num < 0) { break; } if (num > 1000) { continue; }
numbers[i++] = num; } mostFrequentNumber(numbers, &mostFrequent, &numberOfOccurences); printf("The number %d occurs the most times, a total of %d times. ", mostFrequent, numberOfOccurences); return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
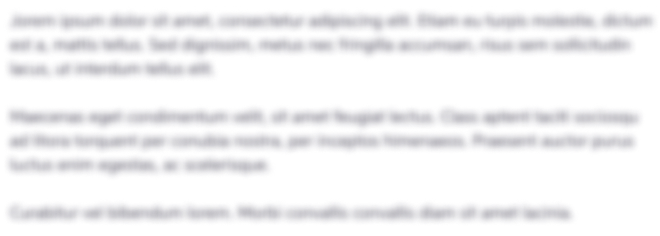
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started