Question
I have written this C++ program below that manages a small music library. I need to implement a class data structure instead of struct. Here
I have written this C++ program below that manages a small music library.
I need to implement a class data structure instead of struct. Here are the following guidelines:
1.) Do not use vector or string class (cstring instead)
2.) Use class named SongList to model the collection of songs.
3.) Use array of Song to implement class SongList
4.) Use int data type to model the duration (minutes and seconds) for each song. You must
do data validation for this to prevent letters or characters from crashing your code. (This is something I didn't do in my program)
5.) When using class, please make sure you encapsulate the data which means make all the
instance data member private and provide accessor methods and mutator methods to
access and manipulate the data.
-Thanks in advance.
-----------------------------------------------------------
#include
using namespace std;
//Declare variables const int MAX_CHAR = 100; const int Title_W = 40; const int Artist_W = 40; const int Duration_W = 40; const int Album_W = 40;
//Create a type song struct song { char title[MAX_CHAR]; char artist[MAX_CHAR]; char duration[MAX_CHAR]; char album[MAX_CHAR]; };
//Declare functions void displyOpt(); char option(); void readinSearch(char search[]); void readInput (const char prompt[], char inputStr[], int maxChar); void executeOption(char opt, song list[], int& listSize); void allSongs(const song list[], int listSize); void readInEntry(song& anEntry); void addEntry(const song& anEntry, song list[], int& listSize); bool artistSearch(const char search[], const song list[], int listSize); bool albumSearch(const char search[], const song list[], int listSize); void loadFile(const char fileName[], song list[], int& listSize); void saveFile(const char fileName[], const song list[], int listSize); void rmSong(song list[], int& listSize);
//Main function int main() { int listSize; char opt; song list[MAX_CHAR]; char fileName[] = "songs.txt"; loadFile(fileName, list, listSize); displyOpt(); opt = option(); //while user doesn't exit, display menu while (opt != 'f') { executeOption(opt, list, listSize); displyOpt(); opt = option(); } saveFile(fileName, list, listSize); return 0; }
//The menu void displyOpt() { cout << " Welcome to Jonathon's Music Manager. " << "a) View all songs. " << "b) Add a new song. " << "c) Search for songs by artist. " << "d) Search for songs by album. " << "e) Delete a song by index number. " << "f) Exit " << "Please make a selection: "; }
//Read in user input char option() { char opt; cin >> opt; cin.ignore(100, ' '); return tolower(opt); }
//Menu options void executeOption(char opt, song list[], int& listSize) { song entry; char search[MAX_CHAR]; switch (opt) { case 'a': allSongs(list, listSize); break; case 'b': readInEntry(entry); addEntry(entry, list, listSize); allSongs(list, listSize); break; case 'c': readinSearch(search); artistSearch(search, list, listSize); break; case 'd': readinSearch(search); albumSearch(search, list, listSize); break; case 'e': rmSong(list, listSize); break; case 'f': //saveFile(fileName, list, listSize); cout << "Saving Changes." << endl; break; default: cout << endl << "Invalid option." << endl; break; } }
//Display all the songs' information in different columns. void allSongs(const song list[], int listSize) { int index; cout << setw(Title_W) << "Title" << setw(Artist_W) << "Artist" << setw(Duration_W) << "Duration" << setw(Album_W) << "Album" << endl; for(index=0; index void readInput (const char prompt[], char inputStr[], int maxChar) { cout << endl << prompt; //read until reach the limit or a new line cin.get(inputStr, maxChar, ' '); while(!cin) { cin.clear (); cin.ignore (100, ' '); cout << endl << prompt; cin.get(inputStr, maxChar, ' '); } cin.ignore (100, ' '); } //searches for word user enters void readinSearch(char search[]) { readInput("Please enter the name of the artist or album: ", search, MAX_CHAR); } //Search the artist column bool artistSearch(const char search[], const song list[], int listSize) { int index; bool found = false; // lists number of matched songs cout << "Here are the matched songs: " << endl; for(index=0; index if(strcmp(search, list[index].artist) == 0) { cout << index << setw(Title_W) << list[index].title << setw(Artist_W) << list[index].artist << setw(Duration_W) << list[index].duration << setw(Album_W) << list[index].album << endl; found = true; } } //When nothing is found display error if ( found == false) { cout << "No matches found." << endl; } return found; } //Search for album bool albumSearch(const char search[], const song list[], int listSize) { int index; bool found = false; cout << "Number of record: " << listSize << endl; cout << "Here are the matched songs: " << endl; for(index=0; index //Add the information user entered into each respective column. void addEntry(const song& anEntry, song list[], int& listSize) { strcpy(list[listSize].title, anEntry.title); strcpy(list[listSize].artist, anEntry.artist); strcpy(list[listSize].duration, anEntry.duration); strcpy(list[listSize].album, anEntry.album); listSize++; } //Load the text file that contains the music information. void loadFile(const char fileName[], song list[], int& listSize) { ifstream in; char title[MAX_CHAR]; char artist[MAX_CHAR]; char duration[MAX_CHAR]; char album[MAX_CHAR]; song anEntry; in.open (fileName); if(!in) { in.clear(); cerr << endl << "Fail to open " << fileName << " for input!" << endl << endl; exit(1); } in.get(title, MAX_CHAR, ';'); while (!in.eof()) { //removes ';' and reads next data. in.get(); in.get(artist, MAX_CHAR, ';'); in.get(); in.get(duration, MAX_CHAR, ';'); in.get(); in.get(album, MAX_CHAR, ';'); in.get(); in.ignore(100, ' '); strcpy(anEntry.title, title); strcpy(anEntry.artist, artist); strcpy(anEntry.duration, duration); strcpy(anEntry.album, album); addEntry(anEntry, list, listSize); in.get(title, MAX_CHAR, ';'); //next record } in.close(); } //Save changes to the file void saveFile(const char fileName[], const song list[], int listSize) { ofstream out; int index; out.open (fileName); if(!out) { out.clear(); cerr << endl << "Fail to open " << fileName << " for output!" << endl << endl; exit(1); } for(index=0; index
Step by Step Solution
There are 3 Steps involved in it
Step: 1
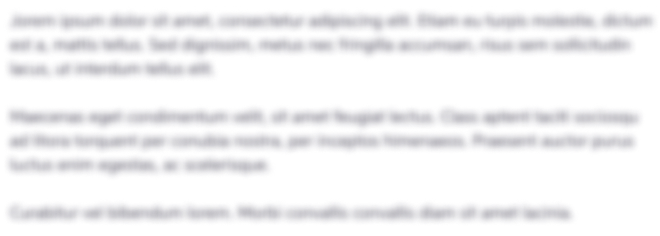
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started