Question
I having a problem coding Part-B of this assignment , I will post my working code for part-A below. I would appreciate any help. Thank
I having a problem coding Part-B of this assignment, I will post my working code for part-A below. I would appreciate any help. Thank you in advance for taking the time to help.
Programming Fundamentals C++
Part - A
Write a program that reads GPA scores from a file called studentData.txt and displays a menu asking the user to choose a, b, or c. In addition, the user also has to option to quit by choosing d. As long as the user does not choose to quit, the program loops over displaying the menu. Your program must also do input validation and print an error message if the user types anything other than a, b, c, or d, then display the menu again and ask the user to reenter the users choice.
a) The number of scores in the file
b) The sum of all the scores in the file
c) The average of all scores in the file
d) Quit
If the user chooses a), b), or c), your program should print the result on the screen (cout).
Use studentData.txt file to test your code, but your code must work for any file which contains GPA scores, where the number of GPA scores is arbitrary.
1. Additional requirements Make sure you meet all the requirements to avoid losing points
1. To complete the assignment, you are required to use only what has been taught in class. If you have prior programming experience, refrain from using more advanced C++ constructs, so all the homework programs can be graded on a consistent basis.
2. You are allowed to hard code the file name in your program.
3. You are required to implement the following functions
getUserChoice: displays the menu, prompts the user to enter the choice, performs input validation, displays the menu again if the input is invalid. Returns the users choice if it is valid
calcNumber: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the number of values in the file, then close the file, and returns the result.
calcSum: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the sum of values in the file, then close the file, and returns the result.
calcAvg: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the average of values in the file, then close the file, and returns the result
Your main() function implements the following pseudocode
call getUserChoice
depending on the users choice, call the appropriate calculation function, or quit print the result on the screen
loop while users choice is not Quit
2. Implementation Notes
If you open the file only at the start of your program and close it only at the end, you will get incorrect results. Make sure you close the file and reopen it at every new calculation, as indicated above in the functions. Doing so will allow your program to read from the beginning of the file.
Part - B
In PART-B, your program reads from a more complicated file, which contains student records. Each record is a students name, followed by that students GPA.
An example of file having 5 student records is shown below studentDataXtraCredit.txt. Use that file to test your code, but the file is only an example, and your code must work for an arbitrary number of records in the file. You can assume the students name has no whitespace in it.
Kai 3.8
Alice 3.9
Seppo 3.2
Ken 3.0
Anna 3.8
The program displays a menu asking the user to choose a, b, c, or d. In addition, the user also has to option to quit by choosing e. As long as the user does not choose to quit, the program loops over displaying the menu. Your program must also do input validation and print an error message if the user types anything other than a, b, c, d, or e, then display the menu again and ask the user to reenter the users choice.
a) The number of scores in the file
b) The sum of all the scores in the file
c) The average of all scores in the file
d) Highest score in the file, along with the name of the student who achieved that score
e) Quit
If the user chooses a), b), c), or d), your program should print the result on the screen (cout).
3. Additional requirements Make sure you meet all the requirements to avoid losing points
1. To complete the assignment, you are required to use only what has been taught in class. If you have prior programming experience, refrain from using more advanced C++ constructs, so all the homework programs can be graded on a consistent basis.
2. You are allowed to hard code the file name in your program.
3. You are required to implement the following functions
> getUserChoice: displays the menu, prompts the user to enter the choice, performs input validation, displays the menu again if the input is invalid. Returns the users choice if it is valid
> calcNumber: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the number of values in the file, then close the file, and returns the result.
> calcSum: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the sum of values in the file, then close the file, and returns the result.
> calcAvg: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the average of values in the file, then close the file, and returns the result
> findHighest: open the file (checks for file open failure and exit the program if there is failure), read the data, finds the highest GPA and the corresponding students name in the file, then close the file, and returns the result
Your main() function implements the following pseudocode
call getUserChoice
depending on the users choice, call the appropriate calculation function, or quit print the result on the screen
loop while users choice is not Quit
4. Implementation Hints
If you open the file only at the start of your program and close it only at the end, you will get incorrect results. Make sure you close the file and reopen it at every new calculation, as indicated above in the functions. Doing so will allow your program to read from the beginning of the file.
> findHighest: To find the highest score, you can use essentially the same algorithm as provided on the lecture slides to find the highest value in an array. However, the algorithm needs to be adapted, because the score values are not readily available in an array, but will be read on the fly from the file. Instead of initializing highest to numbers[0], initialize it to -1. highest will be compared with the score read from the file, instead of numbers[count].
To enable findHighest to return two data items (highest score and students name), you can use a
return statement and a reference variable.
/* This function reads student records from a file
and finds the highest score, along with the name of the student.
The name is returned as a string, and the highest score is passed back as a reference variable
*/
string findHighest(double & score)
{
// Function body
}
Example Output
1. Part -A
2. Part- B
#include
using namespace std;
char getUserChoice(); int calcNumber(); double calcSum(); double calcAvg(); ifstream inputFile;
int main() {
char choice; int num; double sum; double average;
do { choice = getUserChoice(); if (choice == 'a') { num = calcNumber(); cout
}
if (choice == 'c') { average = calcAvg(); cout
} while(choice == 'a' || choice == 'b' || choice == 'c');
/*depending on the users choice, call the appropriate calculation function, or quit print the result on the screen loop while users choice is not Quit*/
return 0; }
char getUserChoice() { char choice; do { cout > choice; if (!(choice == 'a' || choice == 'b' || choice =='c' || choice =='d')) { cout
} while(!(choice == 'a' || choice == 'b' || choice =='c' || choice =='d')); /*displays the menu, prompts the user to enter the choice, performs input validation, displays the menu again if the input is invalid. Returns the users choice if it is valid*/ return choice; }
int calcNumber()/*calcNumber: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the number of values in the file, then close the file, and returns the result.*/ {
double number;
int total = 0; inputFile.open("studentData.txt"); if(! inputFile) { exit(0); } else { while (inputFile >> number) { ++total; } inputFile.close(); } return total; }
double calcSum() /* calcSum: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the sum of values in the file, then close the file, and returns the result.*/ {
double number;
double sumTotal = 0; inputFile.open("studentData.txt"); if(! inputFile) { exit(0); } else { while (inputFile >> number) { sumTotal+=number; } inputFile.close(); } return sumTotal; }
double calcAvg()/*calcAvg: open the file (checks for file open failure and exit the program if there is failure), read the data, calculate the average of values in the file, then close the file, and returns the result*/ {
double number;
int counter = 0; double avgTotal = 0; double total = 0; inputFile.open("studentData.txt"); if(! inputFile) { exit(0); } else { while (inputFile >> number) { ++counter; total+=number; } avgTotal = (total/counter); inputFile.close(); } return avgTotal; }
Make your choice a. Calculate the number of scores in the file Calculate the sum of all scores in the file C. Calculate the average of all scores in the file Quit Number of scores is 5 Make your choice a. Calculate the number of scores in the file Calculate the sum of all scores in the file C. Calculate the average of all scores in the file Quit Sum of scores is 17.7 Make your choice a. Calculate the number of scores in the file Calculate the sum of all scores in the file C. Calculate the average of all scores in the file d. Quit Average of scores is 3.54 Make your choice a. Calculate the number of scores in the file C. Calculate the average of all scores in the file Quit Invalid input Make your choice a Calculate the number of scores in the file b. Calculate the sum of all scores in the file C. Calculate the average of all scores in the file d. Quit Invalid input Make your choice a. Calculate the number of scores in the file b Calculate the sum of all scores in the file C. Calculate the average of all scores in the file d. Quit Process returned 0 (0x0) execution time 15.051 s Press any key to continueStep by Step Solution
There are 3 Steps involved in it
Step: 1
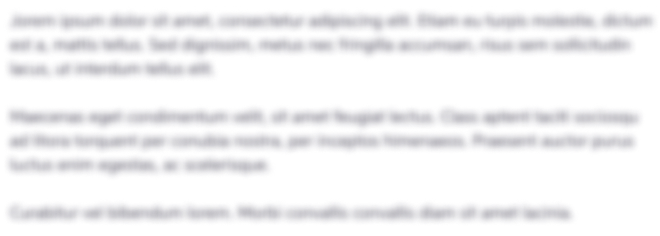
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started