Question
I just about have this finished but I'm struggling on finding my mistake at the end of the program. I'm trying to line up my
I just about have this finished but I'm struggling on finding my mistake at the end of the program. I'm trying to line up my columns Boss Name Ability Damage Health Currency, but when I choose a name with 8 characters then the columns will not align. Also, it's suppose to ask for 5 bosses and yet in my program it is asking for a 6th but doesn't print it out, but I'm lost to how to remove the 6th boss name asked.
import java.util.Scanner;
public class BossReport {
public static void main(String[] args) {
Boss boss1, boss2, boss3, boss4, boss5; // create 5 instances of bosses
Scanner input = new Scanner(System.in);
int index = 1, damage = 0, health = 0, currency = 0;
String bossName, abilityType;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
boss1 = new Boss(bossName, abilityType, damage, health, currency);
index = index + 1;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
boss2 = new Boss(bossName, abilityType, damage, health, currency);
index = index + 1;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
boss3 = new Boss(bossName, abilityType, damage, health, currency);
index = index + 1;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
boss4 = new Boss(bossName, abilityType, damage, health, currency);
index = index + 1;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
boss5 = new Boss(bossName, abilityType, damage, health, currency);
index = index + 1;
// Asks user to enter boss name here and reads the input
System.out.println("Enter " + index + " Boss Name: ");
bossName = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
bossName = bossName.length() > 8 ? bossName.substring(0, 8).toUpperCase() : bossName.toUpperCase();
// Ask user to enter ability name
System.out.println("Enter " + index + " Ability Type (fire breath, slash, magical, etc.) ");
abilityType = input.nextLine();
// the below line converts to upper case and truncates input to 8
// characters
abilityType = abilityType.length() > 8 ? abilityType.substring(0, 8).toUpperCase() : abilityType.toUpperCase();
// while loop to keep on asking user to enter damage between 25 and 50
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
while (damage < 25 || damage > 50) {
System.out.println("Enter " + index + " Damage ( 25-50) :");
damage = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 500 and 999
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
while (health < 500 || health > 999) {
System.out.println("Enter " + index + " Health ( 500-999) :");
health = Integer.parseInt(input.nextLine());
}
// while loop to keep on asking user to enter health between 750 and 999
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
while (currency < 750 || currency > 999) {
System.out.println("Enter " + index + " Currency ( 750-999) :");
currency = Integer.parseInt(input.nextLine());
}
// Print all Bosses results in columns
System.out.println("Boss\tAbility\tDamage\tHealth\tCurrency ");
System.out.println(boss1);
System.out.println(boss2);
System.out.println(boss3);
System.out.println(boss4);
System.out.println(boss5);
}
}
--------------------------------------------------------------------------------------------------------------------------------------
public class Boss {
private String bossName;
private String abilityType;
private int damage;
private int health;
private int currency;
public Boss(String bossName, String abilityType, int damage, int health, int currency) {
super();
this.bossName = bossName;
this.abilityType = abilityType;
this.damage = damage;
this.health = health;
this.currency = currency;
}
public String getBossName() {
return bossName;
}
public void setBossName(String bossName) {
this.bossName = bossName;
}
public String getAbilityType() {
return abilityType;
}
public void setAbilityType(String abilityType) {
this.abilityType = abilityType;
}
public int getDamage() {
return damage;
}
public void setDamage(int damage) {
this.damage = damage;
}
public int getHealth() {
return health;
}
public void setHealth(int health) {
this.health = health;
}
public int getCurrency() {
return currency;
}
public void setCurrency(int currency) {
this.currency = currency;
}
public String toString() {
return this.bossName + "\t" + this.abilityType + "\t" + this.damage + "\t" + this.health + "\t" + this.currency; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
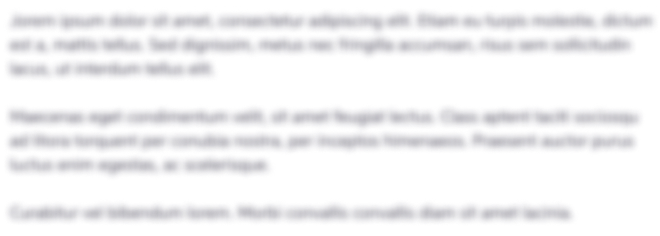
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started