Answered step by step
Verified Expert Solution
Question
1 Approved Answer
i just have one question to ask. Can you help me to change the code to make a borrow account using java applet. In the
i just have one question to ask. Can you help me to change the code to make a borrow account using java applet. In the borrow, account should have all the attributes like book title, author, name and for the borrower just borrow id and name.here I put also the file that can be reference. Thank you.
import java.util.*; import java.io.*; import java.awt.*; import javax.swing.*; import javax.swing.plaf.synth.ColorType; import javax.swing.table.DefaultTableCellRenderer; import javax.swing.table.TableColumn; import java.awt.event.*; public class Librarian implements ActionListener{ //Frames static JFrame LMainFrame; static JFrame addBookFrame; static JFrame delBookFrame; static JFrame disBookFrame; static JFrame temp; //Buttons private static JButton LaddButton; private static JButton LaddSubmit; private static JButton LaddBack; private static JButton LdelButton; private static JButton LdelSubmit; private static JButton LdelBack; private static JButton LdispButton; private static JButton LdispBack; private JPanel disBookPanel; //Text Field private static JTextField bnField,auField,didField; static int bid; static String bn,au; ArrayListbooks = new ArrayList (); File file = new File("Book.txt"); public static void main(String args[]){ init(); } public static void init(){ JPanel LMainPanel; JLabel LMainLabel; LMainPanel = new JPanel(); LMainPanel.setLayout(null); LMainFrame = new JFrame("Librarian Menu"); LMainFrame.setLocation(new Point(750,400)); LMainFrame.add(LMainPanel); LMainFrame.setSize(new Dimension(400,250)); LMainFrame.setResizable(false); LMainFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); LMainLabel = new JLabel("Welcome Librarian"); LMainLabel.setBounds(0, 0, 400, 50); LMainLabel.setHorizontalAlignment(SwingConstants.CENTER); LMainPanel.add(LMainLabel); //Create add button LaddButton = new JButton("Add Book"); LaddButton.setBounds(125,50,150,25); //Create delete button LdelButton = new JButton("Delete Book"); LdelButton.setBounds(125,90,150,25); //Create display button LdispButton = new JButton("Display Book"); LdispButton.setBounds(125,130,150,25); LMainPanel.add(LMainLabel); LMainPanel.add(LaddButton); LMainPanel.add(LdelButton); LMainPanel.add(LdispButton); Librarian lib = new Librarian(); LaddButton.addActionListener(lib); LdelButton.addActionListener(lib); LdispButton.addActionListener(lib); LMainFrame.setVisible(true); } public void actionPerformed(ActionEvent e){ //go to Add Book page if(e.getSource() == LaddButton){ loadBook(); LMainFrame.dispose(); int maxBookID = 0; for(Book book : books){ if(book.getBookID()>maxBookID){ maxBookID =book.getBookID(); } } maxBookID++; bid = maxBookID; addBook(); //Submit Book information to add book }else if(e.getSource() == LaddSubmit){ loadBook(); if(bnField.getText().isEmpty()|| auField.getText().isEmpty()){ JOptionPane.showMessageDialog(addBookFrame, "Please fill in all the information!", "Empty Input", JOptionPane.INFORMATION_MESSAGE); }else{ bn = bnField.getText(); au = auField.getText(); books.add(new Book(bid,bn,au,true)); JOptionPane.showMessageDialog(addBookFrame, "Book Added Successfully!", "Success", JOptionPane.INFORMATION_MESSAGE); addBookFrame.dispose(); saveBook(); init(); } }else if(e.getSource() == LaddBack){ addBookFrame.dispose(); init(); //go to Delete Book page }else if(e.getSource() == LdelButton){ loadBook(); LMainFrame.dispose(); delBook(); //Submit BookID to Delete }else if(e.getSource() == LdelSubmit){ if(didField.getText().isEmpty()){ JOptionPane.showMessageDialog(delBookFrame, "Please fill in Book ID that you want to delete!", "Empty Input", JOptionPane.INFORMATION_MESSAGE); }else{ loadBook(); int did = Integer.parseInt(didField.getText()); int f = -1; String DeletedB = null; for (int i = 0; i < books.size(); i++) { if (did == books.get(i).getBookID()) { // get(i) is to get the data from the i value of array & define getBookID() to get the bookID f=i; DeletedB = books.get(f).getBookName(); books.remove(i); break; } } if(f==-1){ JOptionPane.showMessageDialog(delBookFrame, "Book ID Not Found!", "No Record Found", JOptionPane.INFORMATION_MESSAGE); return; } else{ System.out.println( DeletedB + " Succusfully Deleted"); } JOptionPane.showMessageDialog(delBookFrame, "Book Deleted Successfully!", "Success", JOptionPane.INFORMATION_MESSAGE); delBookFrame.dispose(); saveBook(); delBook(); } //Back to Menu }else if(e.getSource() == LdelBack){ delBookFrame.dispose(); init(); //go to Display Book Page }else if(e.getSource() == LdispButton) { loadBook(); LMainFrame.dispose(); disBookFrame = new JFrame("Display Books"); disBookFrame.setSize(new Dimension(600,300)); disBookFrame.setLayout(null); disBookPanel = new JPanel(); disBookPanel.setBounds(25,25, 535, 550); disBookPanel.setLayout(new GridLayout(3, 4, 10, 10)); disBookFrame.setLocation(new Point(650,350)); disBookFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); displayBook(); LdispBack = new JButton("Back"); LdispBack.setBounds(225,220,120,25); disBookFrame.add(LdispBack); disBookFrame.add(disBookPanel); disBookFrame.setVisible(true); Librarian lib = new Librarian(); LdispBack.addActionListener(lib); }else if(e.getSource() == LdispBack){ disBookFrame.dispose(); init(); } } //Add Book Page public void addBook(){ Librarian lib = new Librarian(); addBookFrame = new JFrame("Add Book"); addBookFrame.setSize(new Dimension(400,250)); addBookFrame.setLayout(null); JPanel addBookPanel = new JPanel(); addBookPanel.setBounds(50,25, 300, 200); addBookPanel.setLayout(new GridLayout(5, 2, 10, 10)); addBookFrame.setLocation(new Point(750,400)); addBookFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Create labels and text fields JLabel bidLabel = new JLabel("Book ID:"); JLabel bidLabel1 = new JLabel(""+(bid)); bidLabel1.setHorizontalAlignment(SwingConstants.CENTER); JLabel bnLabel = new JLabel("Book Name:"); bnField = new JTextField(); JLabel auLabel = new JLabel("Author:"); auField = new JTextField(); // Create buttons LaddSubmit = new JButton("Submit"); LaddSubmit.setBounds(210,160,120,25); LaddBack = new JButton("Back"); LaddBack.setBounds(60,160,120,25); // Add components to panel addBookPanel.add(bidLabel); addBookPanel.add(bidLabel1); //addBookPanel.add(bidField); addBookPanel.add(bnLabel); addBookPanel.add(bnField); addBookPanel.add(auLabel); addBookPanel.add(auField); addBookPanel.add(new JLabel()); // Empty space addBookFrame.add(LaddBack); addBookFrame.add(LaddSubmit); // Add panel to frame and show frame addBookFrame.add(addBookPanel); addBookFrame.setVisible(true); LaddSubmit.addActionListener(lib); LaddBack.addActionListener(lib); } public void delBook(){ // Create frame for delete book interface delBookFrame = new JFrame("Delete Book"); delBookFrame.setSize(new Dimension(1000, 350)); delBookFrame.setLayout(null); JPanel delPanelBackground = new JPanel(); delPanelBackground.setBounds(80, 25, 200, 250); delPanelBackground.setBackground(Color.WHITE); // Create panel for delete book interface JPanel delBookPanel = new JPanel(); delBookPanel.setBounds(100, 25, 160, 250); delBookPanel.setBackground(Color.WHITE); delBookPanel.setLayout(new GridLayout(6, 1, 10, 10)); delBookFrame.setLocation(new Point(500, 350)); delBookFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Create labels for table header JLabel bidLabel = new JLabel("Book ID"); bidLabel.setHorizontalAlignment(SwingConstants.CENTER); didField = new JTextField(); didField.setHorizontalAlignment(SwingConstants.CENTER); //Create buttons LdelSubmit = new JButton("Delete"); LdelSubmit.setBounds(120,180,120,25); LdelBack = new JButton("Back"); LdelBack.setBounds(120,220,120,25); // Add labels to panel delBookPanel.add(new JLabel()); // Empty space delBookPanel.add(bidLabel); delBookPanel.add(didField); //Add buttons delBookFrame.add(LdelSubmit); delBookFrame.add(LdelBack); Librarian lib = new Librarian(); // Add panel to frame delBookFrame.add(delBookPanel); delBookFrame.add(delPanelBackground); // Make frame visible delBookFrame.setVisible(true); //Add button actionListener LdelSubmit.addActionListener(lib); LdelBack.addActionListener(lib); disBookPanel = new JPanel(); disBookPanel.setBounds(350,50, 535, 550); disBookPanel.setLayout(new GridLayout(3, 4, 10, 10)); displayBook(); delBookFrame.add(disBookPanel); /*Scanner sc = new Scanner(System.in); System.out.println("enter Book ID to del"); int did = Integer.parseInt(sc.nextLine()); int f = -1; String DeletedB = null; for (int i = 0; i < books.size(); i++) { if (did == books.get(i).getBookID()) { // get(i) is to get the data from the i value of array & define getBookID() to get the bookID f=i; DeletedB = books.get(f).getBookName(); books.remove(i); break; } } if(f==-1){ System.out.println("No record"); } else{ System.out.println( DeletedB + " Succusfully Deleted"); }*/ } public void displayBook(){ String[] columnNames = {"Book ID", "Book Name", "Author","Status"}; Object[][] data = new Object[books.size()][4]; for (int i = 0; i < books.size(); i++) { Book b = books.get(i); data[i][0] = b.getBookID(); data[i][1] = b.getBookName(); data[i][2] = b.getAuthor(); data[i][3] = b.getStatusString(); } JTable table = new JTable(data, columnNames); TableColumn Column1 = table.getColumnModel().getColumn(0); Column1.setPreferredWidth(50); TableColumn Column2 = table.getColumnModel().getColumn(1); Column2.setPreferredWidth(250); TableColumn Column3 = table.getColumnModel().getColumn(2); Column3.setPreferredWidth(150); TableColumn Column4 = table.getColumnModel().getColumn(3); Column4.setPreferredWidth(80); DefaultTableCellRenderer centerRenderer = new DefaultTableCellRenderer(); centerRenderer.setHorizontalAlignment(JLabel.CENTER); table.getColumnModel().getColumn(0).setCellRenderer(centerRenderer); table.getColumnModel().getColumn(3).setCellRenderer(centerRenderer); table.setFillsViewportHeight(true); JScrollPane scrollPane = new JScrollPane(table); disBookPanel.add(scrollPane); } public void saveBook(){ try{ PrintWriter add = new PrintWriter(file); for(Book a : books){ add.println(a.getBookID()); add.println(a.getBookName()); add.println(a.getAuthor()); add.println(a.getStatus()); } add.close(); } catch (IOException e){ System.out.println("Failed to save book: " + e.getMessage()); } } public void loadBook(){ try{ Scanner sc = new Scanner(file); while(sc.hasNextLine()){ int bbid = Integer.parseInt(sc.nextLine()); String n = sc.nextLine(); String a = sc.nextLine(); Boolean s = Boolean.parseBoolean(sc.nextLine()); books.add(new Book(bbid,n,a,s)); } sc.close(); } catch (IOException e){ System.out.println("Failed to load book: " + e.getMessage()); } } }
librarian change to borrow account.txt
Displaying librarian change to borrow account.txt.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
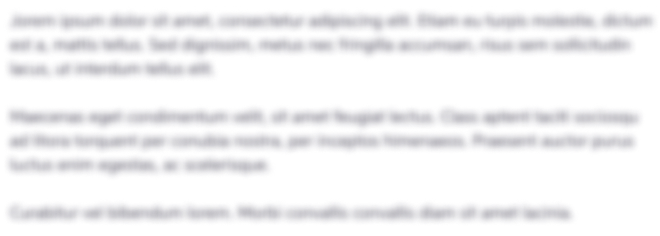
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started