Question
i just need appointmentDiary.java there are more files related, time, timeslot, patient, but couldn't attach them for space issues. Doctor.java: package option1.stage1; import java.util.ArrayList; import
i just need appointmentDiary.java
there are more files related, time, timeslot, patient, but couldn't attach them for space issues.
Doctor.java:
package option1.stage1;
import java.util.ArrayList;
import java.util.Arrays;
public class Doctor {
private String name;
private String speciality;
/*
* note specialities is static means that only one instance is created
* irrespective of number of Doctor instances and that one instance of
* specialities is shared by all instances. it can be accessed by Doctor.specialities
*/
public static ArrayList specialities = new ArrayList(Arrays.asList("General Practitioner", "Dentist", "Surgeon",
"Pediatrician", "Podiatrician", "Dermatologist",
"Radiologist", "Pathologist", "Physician",
"Obstetrician", "Gynaecologist", "Oncologist"));
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSpeciality() {
return speciality;
}
/**
* set speciality of the calling object to the passed value IF the value
* exists (case insensitive) in the ArrayList specialities.
* If the passed value doesn't exist in the ArrayList specialities, set
* the speciality of the calling object to "General Practitioner".
* For example, if spec = "oncologist" or if spec = "Oncologist" or spec = "ONCOLOGIST",
* instance variable speciality should become "Oncologist"
* @param spec
*/
public void setSpeciality(String spec) {
speciality="General Practitioner"; //sets speciality to general practitioner initially
for (int k=0; k
if (specialities.get(k).equalsIgnoreCase(spec)) { //checks if passed value exists in array list
speciality=specialities.get(k); //resets speciality to passed value
}
}
//TO BE COMPLETED
}
public Doctor(String name, String spec) {
setName(name);
setSpeciality(spec);
}
public String toString() {
return "Dr. "+name+" ("+speciality+")";
}
/**
*
* @param other
* @return true if the names and specialities of the calling object and the parameter object
* are the same (case insensitive)
*/
public boolean equals(Object other) {
if(other instanceof Doctor)
return name.equalsIgnoreCase(((Doctor)other).name) && speciality.equalsIgnoreCase(((Doctor)other).speciality);
else
return false;
}
}
(different file)Appointment.java:
package option1.stage3;
import option1.stage1.Doctor;
import option1.stage1.Patient;
import option1.stage2.TimeSlot;
public class Appointment {
private Doctor doctor;
private Patient patient;
private TimeSlot timeSlot;
public Doctor getDoctor() {
return doctor;
}
public void setDoctor(Doctor doctor) {
this.doctor = doctor;
}
public Patient getPatient() {
return patient;
}
public void setPatient(Patient patient) {
this.patient = patient;
}
public TimeSlot getTimeSlot() {
return timeSlot;
}
public void setTimeSlot(TimeSlot timeSlot) {
this.timeSlot = timeSlot;
}
public Appointment(Doctor doctor, Patient patient, TimeSlot timeSlot) {
setDoctor(doctor);
setPatient(patient);
setTimeSlot(timeSlot);
}
public Appointment(Patient patient, TimeSlot timeSlot) {
setDoctor(null);
setPatient(patient);
setTimeSlot(timeSlot);
}
public String toString() {
if(doctor != null)
return patient+" with "+doctor+" for "+timeSlot;
else
return patient+" for "+timeSlot;
}
/**
*
* @param other
* @return true if calling object conflicts with parameter Appointment.
* we say two appointments conflict if they are with the same doctor
* and the timeSlots overlap.
* HINT: Use method equals from Doctor class and method overlapsWith from TimeSlot class
*/
public boolean conflictsWith(Appointment other) {
if(this.doctor.equals(other.doctor) && this.timeSlot.overlapsWith(other.timeSlot)) { //checks if 2 appointments are with the same doctor and timeslots overlap
return true;
}
else return false; //TO BE COMPLETED
}
}
(different file)AppointmentDiary.java:
package option1.stage4;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Scanner;
import option1.stage1.*;
import option1.stage2.*;
import option1.stage3.*;
public class AppointmentDiary {
private String practiceName;
private ArrayList appointments;
private ArrayList doctors;
/**
* DO NOT MODIFY
* initialize an empty appointment diary
*/
public AppointmentDiary() {
practiceName = "TBA";
appointments = new ArrayList();
doctors = new ArrayList();
}
/**
* DO NOT MODIFY
* Read appointment diary from the data files
* @param name
* @param filenameDocs
* @param filenameAppointments
* @throws Exception
*/
public AppointmentDiary(String name, String filenameDocs, String filenameAppointments) throws Exception {
practiceName = name;
appointments = new ArrayList();
doctors = new ArrayList();
Scanner scanner = new Scanner(new FileReader(filenameDocs));
for(int i=0; i < 4; i++) { //ignore first 4 lines
scanner.nextLine();
}
String line;
while(true) {
try {
line = scanner.nextLine();
}
catch(Exception e) {
break;
}
line = line.trim();
String[] tokens = line.split(", ");
doctors.add(new Doctor(tokens[0].trim(), tokens[1].trim()));
}
scanner.close();
scanner = new Scanner(new FileReader(filenameAppointments));
while(true) {
try {
line = scanner.nextLine();
}
catch(Exception e) {
break;
}
line = line.trim();
String[] tokens = line.split(", +");
Patient patient = new Patient(tokens[0].trim());
String specialityRequested = tokens[1].trim();
String[] start = tokens[2].split(":");
String[] end = tokens[3].split(":");
//System.out.println(patient+" "+Arrays.toString(start)+" "+Arrays.toString(end));
Time startTime = new Time(Integer.parseInt(start[0]), Integer.parseInt(start[1]));
Time endTime = new Time(Integer.parseInt(end[0]), Integer.parseInt(end[1]));
//System.out.println(patient+" "+startTime+" "+endTime);
TimeSlot slotRequested = new TimeSlot(startTime, endTime);
for(Doctor doc: doctors) {
if(doc.getSpeciality().equalsIgnoreCase(specialityRequested)) {
boolean free = true;
for(Appointment app: appointments) {
if(app.getDoctor().equals(doc) && app.getTimeSlot().overlapsWith(slotRequested)) {
free = false;
}
}
Appointment appointmentRequested = new Appointment(doc, patient, slotRequested);
if(free) {
appointments.add(appointmentRequested);
//System.out.println(appointmentRequested+" DONE! ");
break;
}
else {
//System.out.println(appointmentRequested+" FAILED. Slot requested, "+slotRequested+", conflicts with existing appointment for "+conflictingSlot+". ");
}
}
}//end doctor traversal
}
scanner.close();
}
/**
* DO NOT MODIFY
*/
public String toString() {
String result = practiceName+" Doctors: ";
for(Doctor doc: doctors) {
result = result + doc + " ";
}
result = result + " Appointments: ";
for(Appointment app: appointments) {
result = result + app + " ";
}
return result;
}
/**
* DO NOT MODIFY
* @return a list of appointments, each on a new line
*/
public String getAppointments() {
String result = "";
for(Appointment appointment: appointments) {
result = result + appointment + " ";
}
return result;
}
/**
* @param speciality
* @return a list of doctors with passed speciality (case insensitive)
*/
public ArrayList getDoctors(String speciality) {
return null; //TO BE COMPLETED
}
/**
*
* @return a list of specialists medical center doesn't have right now.
* note that you can access the class variable (ArrayList) specialities
* using Doctor.specialities
*/
public ArrayList getMissingSpecialists() {
return null; //TO BE COMPLETED
}
/**
*
* @param appointment: appointment to be added.
* add the appointment to the diary.
* note that the appointment booking might fail due to:
* 1. no specialist on board, or,
* 2. appointment with all specialists for requested speciality already
* exist during the time slot requested.
* @return true if the appointment was added successfully, false otherwise.
*/
public boolean add(Appointment appointment) {
return false; //TO BE COMPLETED
}
/**
*
* @param doc
* remove the doctor and all his/her appointments
*/
public void remove(Doctor doc) {
//TO BE COMPLETED
}
/**
* sort the list of appointments in ascending order of start time
*/
public void sort() {
//TO BE COMPLETED
}
/**
*
* @param other
* @return an AppointDiary object with appointments with a conflict between
* the calling object and the parameter object.
* NOTE:
* if appointment X conflicts with appointment Y, add both X and Y to the list.
*/
public ArrayList getConflictingAppointments(AppointmentDiary other) {
return null; //TO BE COMPLETED
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
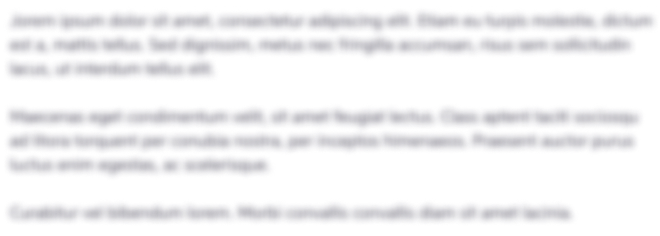
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started