Question
// I just need codes for an assignment operator (=), and a destructor working with the main() and the class Polynomial that I am providing
// I just need codes for an assignment operator ("="), and a destructor working with the main() and the class Polynomial that I am providing below.. Thanks in advance..
#include
#include
#include
using namespace std;
struct Node{
int data;
Node *next;
};
Node* newNode(int data){
Node* node = new Node();
node->data = data;
node->next = NULL;
return node;
}
class Polynomial{
private:
Node* head;
int space;
public:
Polynomial(){
head = newNode(0);
}
Polynomial(initializer_list
initializer_list
initializer_list
last = c.end()-1;
head = newNode(int(*last));
Node* temp = head;
if(c.size() >= 2){
for ( it=c.end()-2; ; it--){
Node* node = newNode(int(*it));
temp->next = node;
temp = node;
space++;
if(it == c.begin()){
break;
}
}
}
}
int getspace()const{
return space;
}
Polynomial(const Polynomial &rhs){ //copy constructor
space = rhs.space;
if(space==0){
head=NULL;
return;
}
else{
Node *t = new Node();
head = t;
t->data=rhs.head->data;
Node *x = rhs.head->next;
while(x!=NULL){
Node *f = new Node();
f->data=x->data;
t->next=f;
t=t->next;
x=x->next;
}
}
}
Node* getHead() const{
return head;
}
void insertBack(int data){
Node* temp = new Node;
temp = head;
while(temp && temp->next){
temp = temp->next;
}
Node *node = newNode(data);
temp->next = node;
}
Polynomial& operator+=(const Polynomial& rhs){
Node *temp1 = rhs.getHead();
Node *temp2 = head;
while(temp1 && temp2){
temp2->data += temp1->data;
temp1 = temp1->next;
temp2= temp2->next;
}
while(temp2){
temp2 = temp2->next;
}
return *this;
}
int evaluate(int val){
Node* g = head;
int x = 1;
int k = 0;
while(g){
g->data *=x;
k += g->data;
g = g->next;
x*=val;
}
return k;
}
friend ostream & operator << (ostream &out, const Polynomial &p);
friend Polynomial operator + (Polynomial const &, Polynomial const &);
};
bool operator==(Polynomial& lhs, Polynomial& rhs) {
if (lhs.getspace() != rhs.getspace()) return false;
Node* lcurrent = lhs.getHead();
Node* rcurrent = rhs.getHead();
while (lcurrent != nullptr && rcurrent != nullptr) {
if (lcurrent->data != rcurrent->data) return false;
lcurrent = lcurrent->next;
rcurrent = rcurrent->next;
}
return true;
}
bool operator!=(Polynomial& lhs, Polynomial& rhs) {
return !(lhs==rhs);
}
ostream & operator << (ostream &out, const Polynomial& p){
Node* temp = new Node;
temp = p.getHead();
int i = 0;
while(temp != NULL){
if(p.getHead()->data==0){
cout<<"0";
}
else if(temp->data!=0){
if(i == 0){
out << temp->data;
i++;
}
else if(i == 1){
out << temp->data <<"x";
i++;
}
else{
out << temp->data << "x" << "^" << i++;
}
}
else {
out<<"";
i++;
}
temp = temp->next;
if(temp&&temp->data!=0){
out << " + ";
}
}
return out;
}
Polynomial operator + (Polynomial const &p1, Polynomial const &p2){
Polynomial p(p1);
Node *temp1 = p.getHead();
Node *temp2 = p2.getHead();
while(temp1 && temp2){
temp1->data += temp2->data;
temp1 = temp1->next;
temp2= temp2->next;
}
while(temp2){
p.insertBack(temp2->data);
temp2 = temp2->next;
}
return p;
}
void doNothing(Polynomial temp) {}
int main() {
//test constructor
Polynomial p1({17});
Polynomial p2({1, 2});
Polynomial p3({-1, 5});
Polynomial p4({5, 4, 3, 2, 1});
Polynomial has_a_zero({4, 0, 1, 7});
cout << "p1: " << p1 << endl;
cout << "p2: " << p2 << endl;
cout << "p3: " << p3 << endl;
cout << "p4: " << p4 << endl;
cout << "has_a_zero: " << has_a_zero << endl;
cout << "p2 + p3: " << (p2+p3) << endl;
cout << "p2 + p4: " << (p2+p4) << endl;
cout << "p4 + p2: " << (p4+p2) << endl;
//test copy constructor - the statement below uses the copy constructor
//to initialize poly3 with the same values as poly4
Polynomial p5(p4);
p5 += p3;
cout << "Polynomial p5(p4); "
<< "p5 += p3; ";
cout << "p4: " << p4 << endl;
cout << "p5: " << p5 << endl;
cout << "Calling doNothing(p5) ";
doNothing(p5);
cout << "p5: " << p5 << endl;
//tests the assignment operator
Polynomial p6;
cout << "p6: " << p6 << endl;
cout << boolalpha; // Causes bools true and false to be printed that way.
cout << "(p4 == p6) is " << (p4 == p6) << endl;
p6 = p4;
cout << "p6: " << p6 << endl;
cout << boolalpha;
cout << "(p4 == p6) is " << (p4 == p6) << endl;
//test the evaluaton
int x = 5;
cout << "Evaluating p1 at " << x << " yields: " << p1.evaluate(5) << endl;
cout << "Evaluating p2 at " << x << " yields: " << p2.evaluate(5) << endl;
Polynomial p7({3, 2, 1}); // 3x^2 + 2x + 1
cout << "p7: " << p7 << endl;
cout << "Evaluating p7 at " << x << " yields: " << p7.evaluate(5) << endl;
cout << boolalpha;
cout << "(p1 == p2) is " << (p1 == p2) << endl;
cout << "(p1 != p2) is " << (p1 != p2) << endl;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
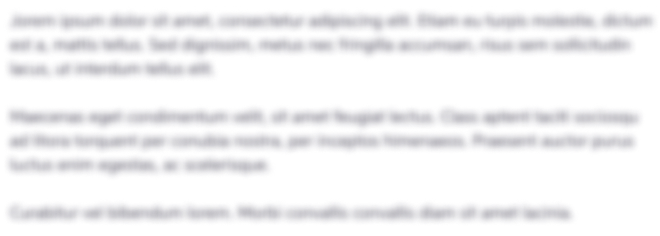
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started