Question
I just need to create the main method but im not good at calling the methods to do this :generate a random list of 20
I just need to create the main method but im not good at calling the methods to do this :generate a random list of 20 cubes (in an array), printing out the list before you sort it, and then printing out the list again after it is sorted using BubbleSort. For an identical list of 20 cubes (copied right after the generation of the array that was given to BubbleSort as an input), show the list before and after the use of the SelectionSort method.
//Cube.java
public class Cube {
public double length; //stores length
public double width; //stores width
public double height; //stores height
//calculate volume of cube & return it
public double calculateVol(){
double vol = length*width*height; //stores volume
return vol;
}
}
//Runner1.java
import java.util.Random;
public class Runner1 {
Cube cube[]; //declaration of variable
Cube newcube[];
//return array of cubes
public Cube[] generateRandom(int size){
cube = new Cube[size];
Random rn = new Random(); //for generation of random number
for(int i=0; i cube[i] = new Cube(); cube[i].length = rn.nextDouble()*100; //random length cube[i].width = (rn.nextDouble()*100 + rn.nextDouble()*100)%101; //random width cube[i].height = rn.nextDouble()*100; //random height } return cube; } //prints cube's length, height, width and volume. public void printCubes(){ for(int i=0; i System.out.print("Cube "+i+": "+"length: "+cube[i].length+" width: "+cube[i].width+" height: "+cube[i].height); System.out.print(" Volume: "+cube[i].calculateVol()); //print volume of cube System.out.println();//print new line } } //method to create new array public void copyArray(){ newcube = new Cube[cube.length]; for(int i=0; i newcube[i] = new Cube(); //creating object of cube class newcube[i].length = cube[i].length; newcube[i].width = cube[i].width; newcube[i].height = cube[i].height; } } //Bubble sort method public void bubbleSort(){ System.out.println("Before Bubble Sort: "); for(int i=0; i System.out.print("Cube "+i+": "+"length: "+cube[i].length+" width: "+cube[i].width+" height: "+cube[i].height); System.out.print(" Volume: "+cube[i].calculateVol()); //print volume of cube System.out.println("");//print double new line } System.out.println(""); Cube temp = new Cube(); boolean swapped; for(int i=0; i swapped = false; for(int j=0; j if(cube[j].calculateVol() > cube[j+1].calculateVol()){ //swapping in element > next element temp = cube[j]; cube[j].length = cube[j+1].length; cube[j].height = cube[j+1].height; cube[j].width = cube[j+1].width; cube[j+1].length = temp.length; cube[j+1].height = temp.height; cube[j+1].width = temp.width; swapped = true; } } // IF no two elements were swapped by inner loop, then break if (swapped == false) break; } System.out.println("After Bubble Sorting: "); for(int i=0; i System.out.print("Cube "+i+": "+"length: "+cube[i].length+" width: "+cube[i].width+" height: "+cube[i].height); System.out.print(" Volume: "+cube[i].calculateVol()); //print volume of cube System.out.println("");//print new line } System.out.println(""); }//end of bubble sort //selection sort method public void selectionSort(){ System.out.println("Before Selection Sort: "); for(int i=0; i System.out.print("Cube "+i+": "+"length: "+newcube[i].length+" width: "+newcube[i].width+" height: "+newcube[i].height); System.out.print(" Volume: "+newcube[i].calculateVol()); //print volume of cube System.out.println("");//print new line } System.out.println(""); Cube temp = new Cube(); int min_idx; //stores index of cube of min volume for(int i=0; i //Find the minimum element in unsorted array min_idx = i; for (int j = i+1; j < newcube[i].length; j++) if (newcube[i].calculateVol() < newcube[min_idx].calculateVol()) min_idx = j; // Swap the found minimum element with the first element temp = newcube[min_idx]; newcube[i].length = newcube[min_idx].length; newcube[i].height = newcube[min_idx].height; newcube[i].width = newcube[min_idx].width; newcube[min_idx].length = temp.length; newcube[min_idx].height = temp.height; newcube[min_idx].width = temp.width; } System.out.println("After Selection Sort: "); for(int i=0; i System.out.print("Cube "+i+": "+"length: "+newcube[i].length+" width: "+newcube[i].width+" height: "+newcube[i].height); System.out.print(" Volume: "+newcube[i].calculateVol()); //print volume of cube System.out.println("");//print new line } System.out.println(""); }//end of selection sort }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
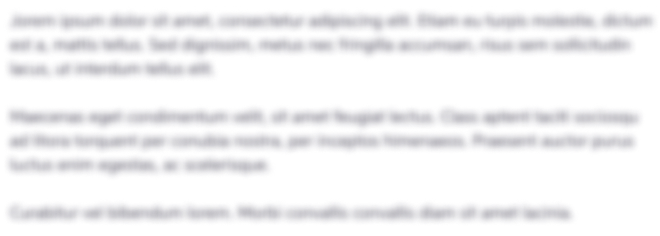
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started