Question
I kind of figured out graph class but It does not pass the complete J-Unit tests and Can someone help me out with J-Unit tests.
I kind of figured out graph class but It does not pass the complete J-Unit tests and Can someone help me out with J-Unit tests. I will appreciate it.
TownGraphManagerTest.java
import static org.junit.Assert.*;
import org.junit.Test;
import static org.junit.Assert.*;
import java.util.ArrayList;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class TownGraphManagerTest {
private TownGraphManagerInterface graph;
private String[] town;
@Before
public void setUp() throws Exception {
graph = new TownGraphManager();
town = new String[12];
for (int i = 1; i < 12; i++) {
town[i] = "Town_" + i;
graph.addTown(town[i]);
}
graph.addRoad(town[1], town[2], 2, "Road_1");
graph.addRoad(town[1], town[3], 4, "Road_2");
graph.addRoad(town[1], town[5], 6, "Road_3");
graph.addRoad(town[3], town[7], 1, "Road_4");
graph.addRoad(town[3], town[8], 2, "Road_5");
graph.addRoad(town[4], town[8], 3, "Road_6");
graph.addRoad(town[6], town[9], 3, "Road_7");
graph.addRoad(town[9], town[10], 4, "Road_8");
graph.addRoad(town[8], town[10], 2, "Road_9");
graph.addRoad(town[5], town[10], 5, "Road_10");
graph.addRoad(town[10], town[11], 3, "Road_11");
graph.addRoad(town[2], town[11], 6, "Road_12");
}
@After
public void tearDown() throws Exception {
graph = null;
}
@Test
public void testAddRoad() {
ArrayList
assertEquals("Road_1", roads.get(0));
assertEquals("Road_10", roads.get(1));
assertEquals("Road_11", roads.get(2));
assertEquals("Road_12", roads.get(3));
graph.addRoad(town[4], town[11], 1,"Road_13");
roads = graph.allRoads();
assertEquals("Road_1", roads.get(0));
assertEquals("Road_10", roads.get(1));
assertEquals("Road_11", roads.get(2));
assertEquals("Road_12", roads.get(3));
assertEquals("Road_13", roads.get(4));
}
@Test
public void testGetRoad() {
assertEquals("Road_12", graph.getRoad(town[2], town[11]));
assertEquals("Road_4", graph.getRoad(town[3], town[7]));
}
@Test
public void testAddTown() {
assertEquals(false, graph.containsTown("Town_12"));
graph.addTown("Town_12");
assertEquals(true, graph.containsTown("Town_12"));
}
@Test
public void testDisjointGraph() {
assertEquals(false, graph.containsTown("Town_12"));
graph.addTown("Town_12");
ArrayList
assertFalse(path.size() > 0);
}
@Test
public void testContainsTown() {
assertEquals(true, graph.containsTown("Town_2"));
assertEquals(false, graph.containsTown("Town_12"));
}
@Test
public void testContainsRoadConnection() {
assertEquals(true, graph.containsRoadConnection(town[2], town[11]));
assertEquals(false, graph.containsRoadConnection(town[3], town[5]));
}
@Test
public void testAllRoads() {
ArrayList
assertEquals("Road_1", roads.get(0));
assertEquals("Road_10", roads.get(1));
assertEquals("Road_11", roads.get(2));
assertEquals("Road_8", roads.get(10));
assertEquals("Road_9", roads.get(11));
}
@Test
public void testDeleteRoadConnection() {
assertEquals(true, graph.containsRoadConnection(town[2], town[11]));
graph.deleteRoadConnection(town[2], town[11], "Road_12");
assertEquals(false, graph.containsRoadConnection(town[2], town[11]));
}
@Test
public void testDeleteTown() {
assertEquals(true, graph.containsTown("Town_2"));
graph.deleteTown(town[2]);
assertEquals(false, graph.containsTown("Town_2"));
}
@Test
public void testDeleteTownSTUDENT() {
fail("Test not yet implemented");
}
@Test
public void testAllTowns() {
ArrayList
assertEquals("Town_1", roads.get(0));
assertEquals("Town_10", roads.get(1));
assertEquals("Town_11", roads.get(2));
assertEquals("Town_2", roads.get(3));
assertEquals("Town_8", roads.get(9));
}
@Test
public void testGetPath() {
ArrayList
assertNotNull(path);
assertTrue(path.size() > 0);
assertEquals("Town_1 via Road_1 to Town_2 2 mi",path.get(0).trim());
assertEquals("Town_2 via Road_12 to Town_11 6 mi",path.get(1).trim());
}
@Test
public void testGetPathA() {
ArrayList
assertNotNull(path);
assertTrue(path.size() > 0);
assertEquals("Town_1 via Road_2 to Town_3 4 mi",path.get(0).trim());
assertEquals("Town_3 via Road_5 to Town_8 2 mi",path.get(1).trim());
assertEquals("Town_8 via Road_9 to Town_10 2 mi",path.get(2).trim());
}
@Test
public void testGetPathB() {
ArrayList
assertNotNull(path);
assertTrue(path.size() > 0);
assertEquals("Town_1 via Road_2 to Town_3 4 mi",path.get(0).trim());
assertEquals("Town_3 via Road_5 to Town_8 2 mi",path.get(1).trim());
assertEquals("Town_8 via Road_9 to Town_10 2 mi",path.get(2).trim());
assertEquals("Town_10 via Road_8 to Town_9 4 mi",path.get(3).trim());
assertEquals("Town_9 via Road_7 to Town_6 3 mi",path.get(4).trim());
}
@Test
public void testGetPathSTUDENT() {
fail("Test not yet implemented");
}}
TestGraphManager.java
import java.util.ArrayList;
public class TownGraphManager implements TownGraphManagerInterface {
@Override
public boolean addRoad(String town1, String town2, int weight, String roadName) {
return false;
}
@Override
public String getRoad(String town1, String town2) {
return null;
}
@Override
public boolean addTown(String v) {
return false;
}
@Override
public boolean containsTown(String v) {
return false;
}
@Override
public boolean containsRoadConnection(String town1, String town2) {
return false;
}
@Override
public ArrayList
return null;
}
@Override
public boolean deleteRoadConnection(String town1, String town2, String road) {
return false;
}
@Override
public boolean deleteTown(String v) {
return false;
}
@Override
public ArrayList
return null;
}
@Override
public ArrayList
return null;
}}
Town.java
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.ArrayList;
public class Town implements Comparable
//private ArrayList towns;
private String name;
private Town templateTown;
private int wt;
//constructor
/**
* @param name
*/
public Town(String name) {
this.name=name;
String[] details = name.split(" of ");
String[] names = details[0].split(" ");
}
public Town(Town templateTown) {
this.setTemplateTown(templateTown);
}
@Override
public int hashCode() {
// TODO Auto-generated method stub
return super.hashCode();
}
@Override
public boolean equals(Object obj) {
// TODO Auto-generated method stub
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Town friend = (Town) obj;
if (name != null ? !name.equals(friend.name) : friend.name != null) return false;
// if (lname != null ? !lname.equals(friend.lname) : friend.lname != null) return false;
// return !(hometown != null ? !hometown.equals(friend.hometown) : friend.hometown != null);
return super.equals(obj);
}
/**
* @param name
*/
public void setName(String name) {
this.name = name;
}
/**
* @return
*/
public String getName() {
// TODO Auto-generated method stub
return name;
}
/* (non-Javadoc)
* @see java.lang.Comparable#compareTo(java.lang.Object)
*/
@Override
public int compareTo(Town o) {
// TODO Auto-generated method stub
/*if(this.name.equals(o.getName()))
{
return 0;
}
/*if(this.weight==(o.weight))
{
return 1;
}*/
/*else return -1;*/
return name.compareTo(o.getName());
}
public String toString()
{
return name;
}
public Town getTemplateTown() {
return templateTown;
}
public void setTemplateTown(Town templateTown) {
this.templateTown = templateTown;
}
public int getWt() {
return wt;
}
/**
* @param i
*/
public void setWt(int i) {
this.wt=i;
}
/*public ArrayList getTowns() {
return towns;
}
public void setTowns(ArrayList towns) {
this.towns = towns;
}
*/
}
Graph.java
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeSet;
public class Graph
private List
private Map adjacencyList ; // [vertices] -> [edge]
private List
//private List > list;//adjacency list
//creates an empty graph
public Graph() {
this.vertices = new ArrayList();
this.edges = new ArrayList
adjacencyList = new HashMap<> ();
}
//creates a graph from vertices and edges stored in a list
public Graph(List
for (int i = 0; i addVertex(vertices.get(i)); } } //creates adjacency list for each vertex public void createAdjacencyList(List for (int i = 0; i addEdge(edges.get(i).getEndPoint1(), edges.get(i).getEndPoint2(), numOfVertices, null); } } /** * Tests the given vertices for null and for membership in the graph. * * @param v1 First vertex to examine. * @param v2 Second vertex to examine. * @throws IllegalArgumentException If any vertex is not part of this graph. * @throws NullPointerException If any vertex is null. */ protected void checkVertices(Town v1, Town v2) { if (!this.containsVertex(v1)) { throw new IllegalArgumentException(); } if (!this.containsVertex(v2)) { throw new IllegalArgumentException(); } } @Override public Road getEdge(Town sourceVertex, Town destinationVertex) { checkVertices(sourceVertex, destinationVertex);//method 1 for check if (destinationVertex == null || sourceVertex == null)//check method 2 { return null; } edges.add((Road) adjacencyList.get(sourceVertex)); //List edge = List.get(sourceVertex.); // List edge = adjacencyList.get(sourceVertex); for (Road e : edges) { if (e.getEndPoint1().equals(sourceVertex) && e.getEndPoint2().equals(destinationVertex)) { return e; } /* if (e.equals(destinationVertex)) { return (Road) edge; }*/ } // return (Road) edge; return null; } @Override public Road addEdge(Town sourceVertex, Town destinationVertex, int weight, String description) { Road e = new Road(sourceVertex, destinationVertex, description); edges.add((Road) e); return (Road) e; /*checkVertices(sourceVertex, destinationVertex);//check 1 if(!containsVertex(sourceVertex)||!containsVertex(destinationVertex)) { throw new IllegalArgumentException(); } if(destinationVertex==null||sourceVertex==null)//check method 2 { throw new NullPointerException(); } if(containsEdge(sourceVertex, destinationVertex)) { Road edge = new Road (sourceVertex, destinationVertex, weight, description); adjacencyList.get(sourceVertex).add(edge); return edge; } //if(edges.get(weight) != null) else return null;*/ } @Override public boolean addVertex(Town v) { /*if(vertices.size()==0){ vertices.add(v); } else { for (int i = 0; i < vertices.size(); i++) { if (vertices.get(i).compareTo(v)>0) { vertices.addAll(i, vertices); return true; } } vertices.addAll(vertices); } return true;*/ adjacencyList.put(v, edges); if (v == null) { throw new NullPointerException(); } //if specified vertex is not already present add new one if (!vertices.contains(vertices.equals(v))) { vertices.add(v); edges.addAll(new ArrayList()); return true; } else { return false; } } @Override public boolean containsEdge(Town sourceVertex, Town destinationVertex) { //List edge = adjacencyList.get(sourceVertex); for (Road e : edges) { if (e.getEndPoint1().equals(sourceVertex) && e.getEndPoint2().equals(destinationVertex)) { return true; } } return false; /*if(getEdge(sourceVertex, destinationVertex)!=null){ return true; } else return false;*/ //return getEdge(sourceVertex, destinationVertex)!=null; } @Override public boolean containsVertex(Town v) { /*if(v==null) { return false; } if(vertices.contains(vertices.equals(v))){ return true; } else return false;*/ adjacencyList.get(v); return vertices.contains(v); } @Override public Set edgeSet() { return null; // TODO Auto-generated method stub //return (Set) adjacencyList.get(vertices); //return new TreeSet(); } @Override public Set edgesOf(Town vertex) { TreeSet h = new TreeSet(); //List edge = adjacencyList.get(vertex); for (Road e : edges) { Town f1 = e.getEndPoint1(); Town f2 = e.getEndPoint2(); if (f1.equals(vertex) || f2.equals(vertex)) { h.add((Road) e); } } return h; } @Override public Road removeEdge(Town sourceVertex, Town destinationVertex, int weight, String description) { //List edge = adjacencyList.get(sourceVertex); for (Road e : edges) { if (((Road) e).getEndPoint1().equals(sourceVertex) && ((Road) e).getEndPoint2().equals(destinationVertex)) { edges.remove(e); return (Road) e; } } return null; } @Override public boolean removeVertex(Town v) { if (v == null) { return false; } vertices.remove(v); return false; } @Override public Set vertexSet() { // TODO Auto-generated method stub return new TreeSet(); } @Override public ArrayList shortestPath(Town sourceVertex, Town destinationVertex) { // TODO Auto-generated method stub return null; } @Override public void dijkstraShortestPath(Town sourceVertex) { // TODO Auto-generated method stub } /*public List getVertices() { return vertices; } public void setVertices(List vertices) { this.vertices = vertices; } public List getEdges() { return edges; } public void setEdges(List edges) { this.edges = edges; } public List > getList() { return list; } public void setList(List > list) { this.list = list; } */ } Road.java public class Road implements Comparable private Town endPoint1, endPoint2; private int weight;//distance between vertices private String roadName; /** * Constructor * @param source- One town on the road * @param destination - Another town on the road * @param degrees - Weight of the edge, i.e., distance from one town to the other * @param name - Name of the road */ public Road(Town source, Town destination, int i, String name) { super(); this.endPoint1 = source; this.endPoint2 = destination; this.roadName=name; this.weight = i; } /** * Constructor with weight preset at 1 * @param source * @param destination * @param name */ public Road(Town source, Town destination, String name) { this.endPoint1=source; this.endPoint2=destination; this.weight= 1; this.roadName=name; } public Road(String town1, String town2, int weight, String roadName2) { this.weight = weight; } /** * @return-the road name */ public String getName() { return roadName; } //returns 0 if the road names are the same, a positive or negative number if the road names are not the same @Override public int compareTo(Road o) { if(roadName.compareTo(roadName)>1) return 1; //return endPoint1.compareTo(endPoint2); return 0; } /** * @return- the first town on the road */ public Town getEndPoint1() { return endPoint1; } public void setEndPoint1(Town endPoint1) { this.endPoint1 = endPoint1; } /** * @return- the second town on the road */ public Town getEndPoint2() { return endPoint2; } public void setEndPoint2(Town endPoint2) { this.endPoint2 = endPoint2; } public void setName(String name) { this.roadName = name; } /** * @return-the distance of the road */ public int getWeight() { return weight; } public void setWeight(int weight) { this.weight = weight; } @Override public String toString() { // TODO Auto-generated method stub return "Edge [source=" + endPoint1 + ", destination=" + endPoint2 + ", distance=" + weight + "]"; } @Override public boolean equals(Object r) { /*Returns true if each of the ends of the road r is the same as the ends of this road. Remember that a road that goes from point A to point B is the same as a road that goes from point B to point A. * */ //if(r.toString().equals(endPoint1) if(this.endPoint1.equals(this.endPoint2)) { //r.getClass(); return true; } /*if(this.weight==(o.weight)) { return 1; }*/ return super.equals(r); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
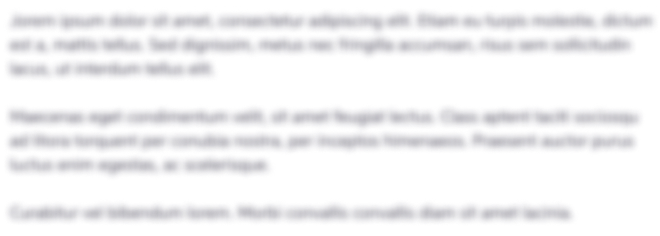
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started