Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I mplement the A* algorithm with the manhattan distance heuristic to solve the sliding tile puzzle game. Your goal is to return the instructions for
Implement the A* algorithm with the manhattan distance heuristic to solve the sliding tile puzzle game. Your goal is to return the instructions for solving the puzzle and show the configuration after each move. Goal state is a 3 by 3 with top 0 1 2 middle 3 4 5 and bottom 6 7 8. A majority of the code is written, I need help computing 3 functions in the PuzzleState class from the source code I provided below (see where comments ""TODO"" are)
import numpy as np import queue class PuzzleState(): SOLVED_PUZZLE = np.arange(9).reshape((3, 3)) def __init__(self,conf,g,predState): self.puzzle = conf # Configuration of the state self.gcost = g # Path cost self._compute_heuristic_cost() # Set heuristic cost self.fcost = self.gcost + self.hcost self.pred = predState # Predecesor state self.zeroloc = np.argwhere(self.puzzle == 0)[0] self.action_from_pred = None def __hash__(self): return tuple(self.puzzle.ravel()).__hash__() def _compute_heuristic_cost(self): """ TODO """ def is_goal(self): return np.array_equal(PuzzleState.SOLVED_PUZZLE,self.puzzle) def __eq__(self, other): return np.array_equal(self.puzzle, other.puzzle) def __lt__(self, other): return self.fcost < other.fcost def __str__(self): return np.str(self.puzzle) move = 0 def show_path(self): if self.pred is not None: self.pred.show_path() if PuzzleState.move==0: print('START') else: print('Move',PuzzleState.move, 'ACTION:', self.action_from_pred) PuzzleState.move = PuzzleState.move + 1 print(self) def can_move(self, direction): """ TODO """ def gen_next_state(self, direction): """ TODO """ print('Artificial Intelligence') print('MP1: A* for Sliding Puzzle') print('SEMESTER: [put semester and year here]') print('NAME: [your name here]') print() # load random start state onto frontier priority queue frontier = queue.PriorityQueue() a = np.loadtxt('mp1input.txt', dtype=np.int32) start_state = PuzzleState(a,0,None) frontier.put(start_state) closed_set = set() num_states = 0 while not frontier.empty(): # choose state at front of priority queue next_state = frontier.get() # if goal then quit and return path if next_state.is_goal(): next_state.show_path() break # Add state chosen for expansion to closed_set closed_set.add(next_state) num_states = num_states + 1 # Expand state (up to 4 moves possible) possible_moves = ['up','down','left','right'] for move in possible_moves: if next_state.can_move(move): neighbor = next_state.gen_next_state(move) if neighbor in closed_set: continue if neighbor not in frontier.queue: frontier.put(neighbor) # If it's already in the frontier, it's guaranteed to have lower cost, so no need to update print(' Number of states visited =',num_states)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
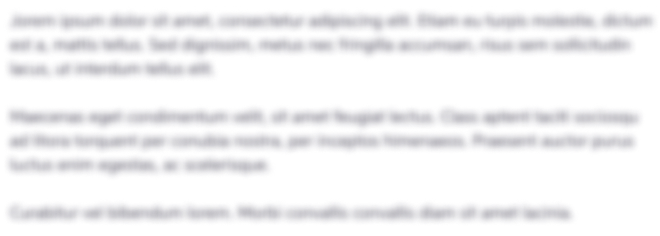
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started