Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I need a pseudocode and algorithm for this following code. along with three output class Node { String key; Node left, right; public Node (
I need a pseudocode and algorithm for this following code. along with three output
class Node
String key;
Node left, right;
public NodeString item
key item;
left right null;
public class BinarySearchTree
Node root;
public BinarySearchTree
root null;
Function to insert a new student into the BST
public void insertString key
root insertRecroot key;
private Node insertRecNode root, String key
if root null
root new Nodekey;
return root;
if keycompareTorootkey
root.left insertRecrootleft, key;
else if keycompareTorootkey
root.right insertRecrootright, key;
return root;
Function to search for a student in the BST
public boolean searchString key
return searchRecroot key;
private boolean searchRecNode root, String key
if root null root.key.equalskey
return root null;
if keycompareTorootkey
return searchRecrootleft, key;
else
return searchRecrootright, key;
Function to delete a student from the BST
public void deleteString key
root deleteRecroot key;
private Node deleteRecNode root, String key
if root null
return root;
if keycompareTorootkey
root.left deleteRecrootleft, key;
else if keycompareTorootkey
root.right deleteRecrootright, key;
else
Node with only one child or no child
if rootleft null
return root.right;
else if rootright null
return root.left;
Node with two children: Get the inorder successor smallest in the right subtree
root.key minValuerootright;
Delete the inorder successor
root.right deleteRecrootright, root.key;
return root;
private String minValueNode root
String minValue root.key;
while rootleft null
minValue root.left.key;
root root.left;
return minValue;
Traversal methods
public void preOrderTraversal
preOrderTraversalRecroot;
private void preOrderTraversalRecNode root
if root null
System.out.printrootkey ;
preOrderTraversalRecrootleft;
preOrderTraversalRecrootright;
public void inOrderTraversal
inOrderTraversalRecroot;
private void inOrderTraversalRecNode root
if root null
inOrderTraversalRecrootleft;
System.out.printrootkey ;
inOrderTraversalRecrootright;
public void postOrderTraversal
postOrderTraversalRecroot;
private void postOrderTraversalRecNode root
if root null
postOrderTraversalRecrootleft;
postOrderTraversalRecrootright;
System.out.printrootkey ;
public static void mainString args
BinarySearchTree bst new BinarySearchTree;
Inserting students
bstinsertJohn;
bstinsertAlice;
bstinsertBob;
bstinsertCharlie;
Performing search operation
System.out.printlnIs Bob present in the tree? bstsearchBob;
Performing deletions
bstdeleteBob; Deleting a leaf node
bstdeleteAlice; Deleting a node with one child
bstdeleteJohn; Deleting a node with two children
Performing traversals
System.out.printlnPreorder traversal:";
bstpreOrderTraversal;
System.out.println;
System.out.printlnInorder traversal:";
bstinOrderTraversal;
System.out.println;
System.out.printlnPostorder traversal:";
bstpostOrderTraversal;
System.out.println;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
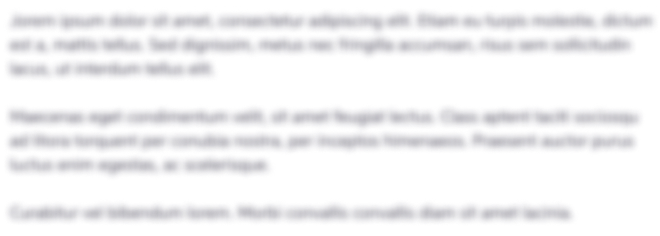
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started