Question
I need an output screenshot for this code. #include using namespace std; struct Node { int info; //element stored in this node Node *next; Node
I need an output screenshot for this code.
#include
using namespace std;
struct Node
{
int info; //element stored in this node
Node *next;
Node *prev;//link to next node
};
class DoublyLinkedList
{
public:
DoublyLinkedList();
void addEnd(int x);
void displayInReverse();
private:
Node *last; //pointer to header (dummy) node
};
DoublyLinkedList::DoublyLinkedList()
{
last = new Node;
last->next = NULL;
last->prev= NULL;
}
////// addEnd function
void DoublyLinkedList::addEnd(int x)
{
Node *p= new Node;
p->info = x;
p->prev = NULL;
if(last->prev != NULL)
{
p->prev = last->prev;
last->prev->next = p;
}
last->prev = p;
p->next = last;
}
// Display Inverse function
void DoublyLinkedList::displayInReverse()
{
Node *p = last;
while(p->prev != NULL)
{
p=p->prev;
cout<
}
cout< } int main() { DoublyLinkedList D; D.addEnd(5); D.addEnd(6); D.addEnd(7); D.displayInReverse(); return 0; } // exercise 3 #include using namespace std; struct Node { char info; //element stored in this node Node *next; }; class Bag { public: Bag(); ~Bag(); bool isEmpty(); void display(); void add(char); void remove(char); void modify(); private: Node *first; //pointer to header (dummy) node }; Bag::Bag() { first = new Node; first->next = NULL; } /* * Destructor. Deallocates all the nodes of the linked list, * including the header node. */ Bag::~Bag() { Node *temp; while (first != NULL) { temp=first; first=first->next; delete temp; } } /* * Determines whether the list is empty. * * Returns true if the list is empty, false otherwise. */ bool Bag::isEmpty() { return first->next == NULL; } /* * Prints the list elements. */ void Bag::display() { Node * current = first->next; while(current != NULL) { cout << current->info << " "; current = current->next; } cout << endl; } /* * Adds the element x to the beginning of the list. * * x: element to be added to the list. */ void Bag::add(char x) { Node *p = new Node; p->info = x; p->next = first->next; first->next = p; } /* * Removes the first occurrence of x from the list. If x is not found, * the list remains unchanged. * * x: element to be removed from the list. */ void Bag::remove(char x) { Node * old = first->next, * p = first; //Finding the address of the node before the one to be deleted bool found = false; while (old != NULL && !found) { if (old->info == x) found = true; else { p = old; old = p->next; } } //if x is in the list, remove it. if (found) { p->next = old->next; delete old; } } void Bag::modify() { int flag[26]; for(int i=0;i<26;i++) { flag[i] = 0; } Node *start = first -> next; Node *prev= start,*temp; while(start != NULL) { if(flag[start->info-'A'] == 0) { flag[start->info-'A'] = 1; prev = start; start = start->next; } else { prev->next = start->next; delete(start); start = prev->next; } } } int main() { Bag B; string s="MISSISSIPPI"; for(int j=0;j { B.add(s[j]); } B.display(); B.modify(); B.display(); return 0; } /// exercise 4 #include #include using namespace std; int main() { list for (int i=0; i<10; i++) numbers.push_back(rand()%21); int table[21]; for(int i=0;i<21;i++) table[i] = 0; list for(it=numbers.begin();it!=numbers.end();it++) { table[*it]++; } for(int i=0;i<21;i++) { cout< } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
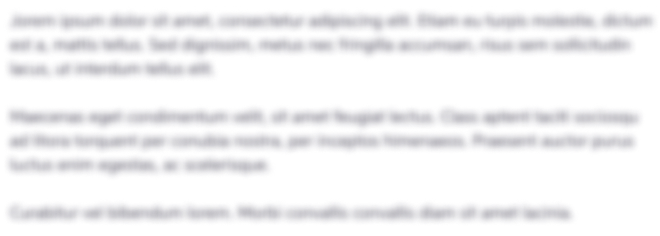
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started