Question
(I need help codding this problem; an explanation would be appreciated. In Python, please, Thank you.) ### Part A Write a function `findAllOddIndices` that takes
(I need help codding this problem; an explanation would be appreciated. In Python, please, Thank you.)
### Part A
Write a function `findAllOddIndices` that takes in a list of integers `lst` of type `List[Int]`
Return a list of indices $[i_1, \ldots, i_k]$ from the original list `lst` such that the list element $e_j$ corresponding to each returned index $i_j$ is odd.
Remember indices start at $0$ and range until the length of list -1.
#### Example
~~~ lst: List( 1, 5, 0, 2, 4, 8, 7) ~~~
should return
~~~ List(0, 1, 6) ~~~
since the elements at indices 0, 1 and 6 are odd.
**Restrictions** are same as problem 1. Do not use list API functions other than `length` and `reverse`. You can use operators `:+`, `++`, and `::` to append elements to a list. - Be careful not to use the list indexing operator `list(i)`.
**Challenge:** Make your code as efficient as possible. Ideally, it should have complexity O(n) though we will not deduct points for inefficient code.
// YOUR CODE HERE ???
//BEGIN TESTS val lst1 = List(1, 3, 0, 2, 4, 6, 5) testWithMessage(findAllOddIndices(lst1), List(0, 1, 6), "Test1")
val lst2 = List(4, 6, -2, 8, 10) testWithMessage(findAllOddIndices(lst2), Nil, "Test2")
val lst3 = List(5, 3, 1, 5, 15, 9 ) testWithMessage(findAllOddIndices(lst3), List(0, 1, 2, 3, 4, 5), "Test3")
val lst4 = List() testWithMessage(findAllOddIndices(lst4), Nil, "Test4")
passed(5) //END TESTS
Is your code above efficient? Try using the following code test. It should run in under a second ideally. We have disabled running this cell just in case you have an inefficent solution that crashes your computer or our autograder. ~~~ val lst = (0 to 100000).toList val ret =findAllOddIndices(lst) assert(ret.length == 50000) ~~~
Step by Step Solution
There are 3 Steps involved in it
Step: 1
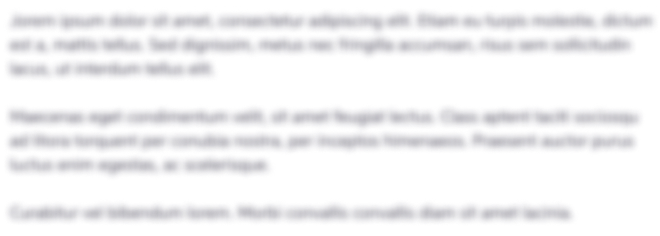
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started