Question
I need help completing functions def linear_probe and def hash in python program. Please do not add any new functions or change any other functions
I need help completing functions def linear_probe and def hash in python program. Please do not add any new functions or change any other functions as they are already completed. Only focus on both linear_probe function and hash function. the purpose of this is the to implement linear probing in to a hash function below I tried coding this myself however I could not get past this issue of unindent error, and itt was making impossible so I figured it was something with my syntax if you can help me out I would greatly appreciate it
# hash table
class hash_table:
# constructor
# inputs: size (defaults to 8 if no arguments are provided)
def __init__(self, size = 8):
# self.table: empty hash table of indicated size
self.table = (None,) * size
# self.size: number of positions in table
self.size = size
# Already completed function!
# INSERTS value INTO HASHTABLE AT index
# example: insert(5, 10) will place 5 into index#10
def insert(self, value, index):
temp = list(self.table)
temp[index] = value
self.table = tuple(temp)
# function name: linear_probe
# input: value- value to be inserted
#start_index- where linear probing starts
# output: returns the index of the hash_table that the value should be
# inserted after linear probing
# assumptions: value will always be an integer
# your table will always be big enough
def linear_probe(self, value, start_index):
# TODO
# hint: empty spots in tuples are labeled as None
pass
# function name: insert
# input: value- value to be inserted
# output: Do not return anything. Just insert value into the proper position
# in self.table. Utilize linear_probe and insert in this function
# assumptions: value will always be an integer
# your table will always be big enough
def hash(self, value):
# TODO
# hint: empty spots in tuples are labeled as None
pass
# Already completed function!
def get_table(self):
return self.table
# Already completed function!
def __str__(self):
return str(self.table)
"""**********************************************************************"""
# test cases
"""**********************************************************************"""
def checker(expected, actual):
if expected == actual:
print("CORRECT!")
else:
print("expected " + str(expected) + ", but got " + str(actual))
"""**********************************************************************"""
test1 = hash_table(5)
test1.hash(9)
test1.hash(25)
test1.hash(10)
test1.hash(14)
expected1 = (25, 10, 14, None, 9)
checker(expected1, test1.get_table())
"""**********************************************************************"""
test2 = hash_table(8)
test2.hash(5)
test2.hash(30)
test2.hash(52)
test2.hash(95)
test2.hash(45)
expected2 = (45, None, None, None, 52, 5, 30, 95)
checker(expected2, test2.get_table())
Step by Step Solution
There are 3 Steps involved in it
Step: 1
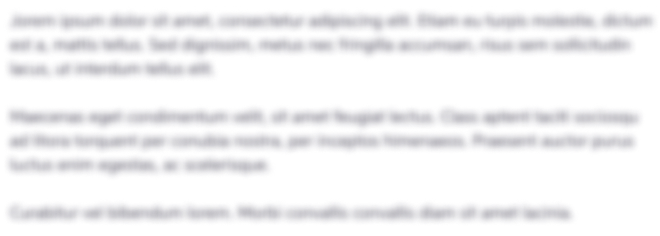
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started