Question
I need help completing the methods intersection, frequentWords, and anagrams in the class HW2.java. HW2 reads lists of words from the textfiles english_words.txt and french_words.txt.
I need help completing the methods intersection, frequentWords, and anagrams in the class HW2.java. HW2 reads lists of words from the textfiles english_words.txt and french_words.txt. The method headers are not allowed to be changed. Thank you for any assistance.
import java.io.*;
import java.util.*;
public class HW2 {
// Prints a list of words that appear in both files.
public static void intersection(String filename1, String filename2) {
try (Scanner in1 = new Scanner(new FileReader(filename1));
Scanner in2 = new Scanner(new FileReader(filename2))) {
// Fill in.
//Reads the two files and prints every word that occurs at least once //in both(the order is not important).
//The runtime must be O(n) where n is the total number of words in //both files.
//Do not sort the words because it is too slow. Use a HashSet to store //the words. //You may assume that a string can be inserted into a hash table in //O(1) time.
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
// Prints all words in the file that occur at least k times
// (print the word followed by the number of occurrences in parentheses).
// Each line in the file contains only one word.
public static void frequentWords(String filename, int k) {
try (Scanner in = new Scanner(new FileReader(filename))) {
// Fill in.
//The runtime must be O(n) where n is the total number of words in the file.
//Do not sort the words. Use a HashMap to count the occurrences of each word // (the key is the word, the value is the number of occurrences).
//If the key is already in the map, update its value (using the get and put //methods), otherwise add a new entry to the map.
//Then iterate through the map to find the frequent words.
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
// Returns the string with the characters sorted alphabetically.
private static String sortString(String s) {
char[] array = s.toCharArray();
Arrays.sort(array);
return new String(array);
}
// Prints all sets of anagrams, one set per line.
// Each line in the file contains only one word.
public static void anagrams(String filename) {
try (Scanner in = new Scanner(new FileReader(filename))) {
// Fill in.
//Reads the file and prints all sets of anagrams, one set per line (the order //is not important). Only print sets that contain 2 or more words.
//In a set of anagrams, you can rearrange the letters of one word to form any //other word, for example [now, own, won].
//You have been given a sortString method that takes a string as a parameter, //sorts the characters alphabetically, and returns the result.
//If two strings are equal to each other after sorting both strings, they are //anagrams.
// I think the easiest solution is to use a map (the key is the sorted word, //the value is either a list or a set of all words that are anagrams with the //sorted word).
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
System.out.println("***Intersection***");
intersection("english_words.txt", "french_words.txt");
System.out.println("***Frequent words***");
frequentWords("english_words.txt", 2);
System.out.println("***Anagrams***");
anagrams("english_words.txt");
}
}
____________________________________________________________________________________
These are examples of the text files.
english_words.txt :
that
pour
peach
you
a
now
you
that
son
grand
grown
on
part
cheap
you
point
wrong
place
now
grand
french_words.txt:
pour
du
une
est
point
son
que
on
part
a
place
a
grand
semble
clients
serait
_____________________________
These are examples of the output.
intersection:
pour
a
son
on
part
point
place
grand
___________
frequentWords:
a (2)
now (2)
that (2)
you (3)
__________________________
anagrams:
[peach, cheap]
[grown, wrong]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
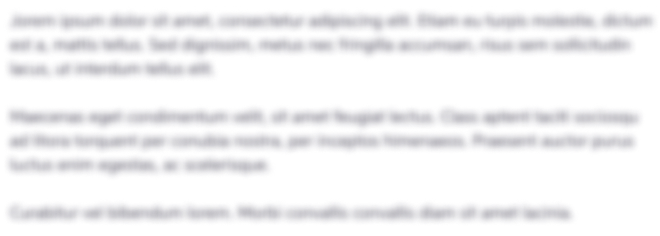
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started