Question
I need help creating a GUI Program for the following code: import java.util.ArrayList; /** * * */ public class Customer { private String ssn; private
I need help creating a GUI Program for the following code:
import java.util.ArrayList;
/** * * */ public class Customer {
private String ssn; private String name; private ArrayList accountList = new ArrayList();
//official constructor for Customer public Customer(String name, String ssn) { this.name = name; this.ssn = ssn; }
//constructor taking in an ssn //used for checking if SSN exists public Customer(String ssn) { this.ssn = ssn; }
//adds account to the list public void addAccount(Account acc) { accountList.add(acc); }
//returns the accountList as an ArrayList. never used public ArrayList getAccountList() { return accountList; }
//checks if the ssn exists public boolean checkIfSSNExists(String s) //s = ssn //return true if exists { if (this.ssn.equals(s)) { return true; } return false; }
//override for the .equals method @Override public boolean equals(Object o) { if (o instanceof Customer) { Customer c = (Customer) o; if (this.ssn.equals(c.ssn)) { return true; } } return false; }
//TestBed public static void main(String[] args) { String ssn = "7777777"; Customer c1 = new Customer("Tony Stark", ssn); if (c1.checkIfSSNExists(ssn)) { System.out.println("Constructor(name, ssn) has passed!"); System.out.println("CheckIfSSNExists method has passed!"); System.out.println(); } else { System.out.println("Constructor(name, ssn) has failed!"); System.out.println("CheckIfSSNExists method has failed!"); System.out.println(); }
Customer c2 = new Customer(ssn); if (c2.checkIfSSNExists(ssn)) { System.out.println("Constructor(ssn) has passed!"); System.out.println("CheckIfSSNExists method has passed!"); System.out.println(); } else { System.out.println("Constructor(ssn) has failed!"); System.out.println("CheckIfSSNExists method has failed!"); System.out.println(); }
if (c1.equals(c2)) { System.out.println("equals method has passed!"); System.out.println(); } else { System.out.println("equals method has failed!"); System.out.println(); }
ArrayList c1_accounts = c1.getAccountList(); if (c1_accounts != null) { System.out.println("accounts list initialised properly!"); System.out.println("getAccountList method has passed!"); System.out.println(); } else { System.out.println("accounts list not initialised properly!"); System.out.println("getAccountList method has failed!"); System.out.println(); }
SavingsAccount sa = new SavingsAccount("c1 saving account", 777, 1); c2.addAccount(sa);
ArrayList c2_accounts = c2.getAccountList(); if (c2_accounts.get(0).equals(sa)) { System.out.println("addAccount has passed!"); System.out.println("Account equals method has passed!"); System.out.println("getAccountList method has passed!"); System.out.println(); } else { System.out.println("addAccount has failed!"); System.out.println("Account equals method has failed!"); System.out.println("getAccountList method has failed!"); System.out.println(); } }
}
-----------------------------------------------------------------------------------------------
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * * */ public abstract class Account implements Comparable
private static int totalAccountNumber; //indicates total number of accounts
protected String accountHolderName; //Account Holder protected int accountNumber; //Account Number //Account type enum
protected enum accountType { SavingsAccount, CheckingAccount, InvalidType }; protected float balance; //Account Balance protected accountType acc; //Account Type Variable - From Enum
//full constructor for account public Account(String name, int accNumber, int accType) { this.accountHolderName = name; this.accountNumber = accNumber;
if (accType == 1) //savings account { acc = accountType.SavingsAccount; } else if (accType == 2) //checking account { acc = accountType.CheckingAccount; }
balance = 0; }
//constructor only taking 1 parameter of account number public Account(int accNum) { this.accountNumber = accNum; }
public abstract float getAccountBalance();
public abstract int returnAccountNumber();
//returns 1 if the account is of type : savings public int getAccountType() { if (acc == accountType.SavingsAccount) { return 1; } else { return 0; } }
//withdraws a float amount from balance public boolean withdraw(float amount) { float temp = balance - amount; if (temp < 0) { return false; } else { balance = temp; } return true; }
//deposits a float amount into balance public boolean deposit(float amount) { balance = balance + amount; return true; }
//used for CheckAccount and SavingsAccount (polymorphism) public String getAccountSummary() { return ""; }
//override using comparable
//override the .equals method. returns true if equal @Override public boolean equals(Object o) { if (o instanceof Account) { Account acc = (Account) o; if (this.accountNumber == acc.accountNumber) { return true; } } return false; }
}
-------------------------------------------------------------------------------------------
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
import java.text.DecimalFormat; import java.util.ArrayList; import java.util.InputMismatchException; import java.util.Scanner;
/** * * */ public class BankAccountManagement {
//maintains list of all accounts and a list of customers private ArrayList
//main method public void run() { System.out.println("Banking System is running...!");
String input = ""; while (!input.equals("q")) { System.out.println("Please enter C to create a new account, " + "M to manage an existing account, " + "L to list all account in the order by balance" + " from lowest to highest, " + "Q to quit");
input = stdin.nextLine();
if (input.equals("c") || input.equals("C")) { createNewAccount(); } else if (input.equals("m") || input.equals("M")) { manageAccount(); } else if (input.equals("l") || input.equals("L")) { listAllAccounts(); } else if (input.equals("q")) { } else { System.out.println("Invalid command."); }
}
System.out.println("Thanks for using Banking System. Bye!"); }
//creates new Account. Called from run() public void createNewAccount() { //gets name of the customer System.out.println("Please enter the name of the customer:"); String name = stdin.nextLine();
//checks and gets a value for SSN String ssn = ""; boolean ssnValid = false; //checks if SSN is valid boolean ssnExists = false; //check if SSN already exists System.out.println ("Please enter the SSN of the customer (***-**-****):"); while (!ssnValid || ssnExists) { try {
ssn = stdin.nextLine(); ssnValid = ssnValid(ssn); //throws illegal argument exception ssnExists = ssnExists(ssn); } catch (Exception e) { System.out.println("Invalid Social Security Number. " + e); System.out.println("Please enter valid SSN:"); } }
Customer newCust = new Customer(name, ssn); customerList.add(newCust);
int accountType = getAccountType(); int accountNumber = getAccountNumber();
if (accountType == 1) //savings account { SavingsAccount newAcc = new SavingsAccount(name, accountNumber, accountType); accountList.add(newAcc); newCust.addAccount(newAcc);
DecimalFormat f = new DecimalFormat("##.##"); String stringSummary = ("Account holder is " + name + "; Account number is " + accountNumber + "; Account type is savings" + "; Account balance is " + f.format(newAcc.getAccountBalance()));
System.out.println ("The new account has been created. Account summary: " + stringSummary); } else if (accountType == 2) //checking account { CheckingAccount newAcc = new CheckingAccount(name, accountNumber, accountType); accountList.add(newAcc); newCust.addAccount(newAcc);
DecimalFormat f = new DecimalFormat("##.##"); String stringSummary = ("Account holder is " + name + "; Account number is " + accountNumber + "; Account type is checking" + "; Account balance is " + f.format(newAcc.getAccountBalance()));
System.out.println ("The new account has been created. Account summary: " + stringSummary); }
}
//gets an account number and returns it //used for createNewAccount() public int getAccountNumber() { int accountNumber = 0; boolean checkBool = false; int accNum = 0; System.out.println("Please enter account number with five digits:"); while (!checkBool) { try { accNum = stdin.nextInt(); if (accNum < 10000 || accNum > 99999) { System.out.println ("Invalid account number. Please try again"); } else { checkBool = true; } } catch (Exception e) { System.out.println ("Invalid Input. " + e + " Please try again"); } finally { stdin.nextLine(); } } accountNumber = accNum; return accountNumber; }
//gets an account number and returns it //used for manageAccount() public int getAccountNumber2() { int accountNumber = 0; boolean checkBool = false; boolean check = false; int accNum = 0; System.out.println("Please enter your account number:"); while (!checkBool) { try { accNum = stdin.nextInt(); if (accNum < 10000 || accNum > 99999) { System.out.println("Invalid number. Please try again"); } else { checkBool = true; }
if (checkBool) { check = checkIfAccountExists(accNum); //if check is false, the account doesn't exist if (!check) { checkBool = false; } } } catch (Exception e) { System.out.println("Invalid Input. " + e + " Please try again"); } finally { stdin.nextLine(); } }
accountNumber = accNum; return accountNumber; }
//gets the account type and returns it as an int. //1 is savings, 2 is checking //used for createNewAccount public int getAccountType() { int accountType = 0; boolean checkBool = false; String temp = ""; int type = 0; System.out.println("Please select account type: 1.Savings, 2.Checking"); while (!checkBool) { try { type = stdin.nextInt(); if (type == 1 || type == 2) { checkBool = true; } else { System.out.println("Invalid type. Please try again"); } } catch (Exception e) { System.out.println("Invalid Input. " + e + " Please try again"); } finally { stdin.nextLine(); } } accountType = type; return accountType; }
//checks if the string is an integer //no longer used by this program
public boolean checkIfNumber(String s) { boolean notNumbers = false; char[] c = s.toCharArray(); for (int z = 0; z < c.length; z++) { if (c[z] > '9' || c[z] < '0') { notNumbers = true; throw new InputMismatchException(); } } return true; }
//checks if the ssn inputted is in the valid format //used for createNewAccount() public boolean ssnValid(String ssn) { char[] c = ssn.toCharArray(); if (c.length != 11) { throw new IllegalArgumentException ("The length of ssn has to be 11."); } if (Character.isDigit(c[0]) && Character.isDigit(c[1]) && Character.isDigit(c[2]) && (c[3] == '-') && Character.isDigit(c[4]) && Character.isDigit(c[5]) && (c[6] == '-') && Character.isDigit(c[7]) && Character.isDigit(c[8]) && Character.isDigit(c[9]) && Character.isDigit(c[10])) { return true; }
throw new IllegalArgumentException("wrong format"); }
//checks if the ssn already exists //used for createNewAccount() public boolean ssnExists(String ssn) { for (int i = 0; i < customerList.size(); i++) { Customer tempCust = new Customer(ssn); if (customerList.contains(tempCust)) { return true; } } return false; }
//checks if the id inputted is valid. takes a string, returns a boolean //used for createNewAccount() public static boolean isIdValid(String s) throws InputMismatchException { char[] c = s.toCharArray(); if (c.length != 5) { throw (new InputMismatchException()); } for (int i = 0; i < 5; i++) { if (Character.isLetter(c[i])) { throw (new InputMismatchException()); } } return true; }
//manage account. called from run(). has a menu system public void manageAccount() { //System.out.println("Please enter your account number:"); if (accountList.size() == 0) { System.out.println ("The number of accounts is 0. Please create an account first."); return; }
int accountNumber = getAccountNumber2();
Account savAcc = getAccountFromNumber(accountNumber); //Account
System.out.println("Please select account type:" + " 1.View account summary," + " 2.Withdraw, 3.Deposit, 4.Main menu"); String input = ""; int inp = 0; boolean bool = false; while (!bool) { try { inp = stdin.nextInt();
if (inp == 1 || inp == 2 || inp == 3 || inp == 4) { bool = true; } else { bool = false; System.out.println("Invalid type. Please try again"); } } catch (Exception e) { System.out.println("Invalid Input. " + e + " Please try again"); } finally { stdin.nextLine(); } }
//if (input.equals("1")) if (inp == 1) { MA_ViewAccount(accountNumber); } //else if (input.equals("2")) else if (inp == 2) { MA_Withdraw(savAcc); } //else if (input.equals("3")) else if (inp == 3) { MA_Deposit(savAcc); } //else if (input.equals("4")) else if (inp == 4) { return; } else { System.out.println("Invalid type. Please try again"); }
}
//returns an account from a valid account number public Account getAccountFromNumber(int an) { String tempstr = ""; int temp = 0; SavingsAccount sa = new SavingsAccount(tempstr, an, temp); CheckingAccount ca = new CheckingAccount(tempstr, an, temp); Account savAcc; //this is the account we are using. if (accountList.contains(sa)) { int index = accountList.indexOf(ca); savAcc = accountList.get(index); return savAcc; } else if (accountList.contains(ca)) { int index = accountList.indexOf(ca); savAcc = accountList.get(index); return savAcc; } return null; }
//checks if the account already exists //used for ViewingAccount public boolean checkIfAccountExists(int an) //an = account number { String tempstr = ""; int temp = 0; SavingsAccount sa = new SavingsAccount(tempstr, an, temp); CheckingAccount ca = new CheckingAccount(tempstr, an, temp); if (accountList.contains(sa)) { return true; } else if (accountList.contains(ca)) { return true; } else { System.out.println("The account number you entered does not exist. Please try again"); } return false; }
//prints out account information //called from manage() public void MA_ViewAccount(int an) //an = account number { String tempstr = ""; int temp = 0; SavingsAccount sa = new SavingsAccount(tempstr, an, temp); CheckingAccount ca = new CheckingAccount(tempstr, an, temp); if (accountList.contains(sa)) { int index = accountList.indexOf(ca); Account savAcc = accountList.get(index); System.out.println(savAcc.getAccountSummary()); } else if (accountList.contains(ca)) { int index = accountList.indexOf(ca); Account savAcc = accountList.get(index); System.out.println(savAcc.getAccountSummary()); } else { System.out.println("failed in MA_ViewAccount. couldn't find account"); } }
//withdraws money from Account acc //called from manage() public void MA_Withdraw(Account acc) { float accBalance = acc.getAccountBalance();
if (acc instanceof SavingsAccount) { SavingsAccount sava = (SavingsAccount) acc; sava.addMonthlyInterest(); }
if (accBalance == 0) { System.out.println ("The balance is 0.0, Please deposit before withdraw."); } else { float moneyInputted = getMoneyInputted(); if (moneyInputted > accBalance) { System.out.println ("You don't have enough balance. Please try a different amount."); } else { acc.withdraw(moneyInputted); DecimalFormat f = new DecimalFormat("##.##");
System.out.println("Your withdrawal has been accepted " + "with an amount of " + f.format(moneyInputted)); }
} }
//deposits money into Account acc //called from manage() public void MA_Deposit(Account acc) { float moneyInputted = getMoneyInputted();
acc.deposit(moneyInputted);
DecimalFormat f = new DecimalFormat("##.##");
System.out.println ("Your deposit has been received with " + "an amount of " + f.format(moneyInputted)); }
//returns a float value for the value inputted //used in MA_Withdraw() and MA_Deposit() public float getMoneyInputted() { float numberInputted = 0.0f; boolean checkBool = false; System.out.println("Please enter the amount of money:"); while (!checkBool) { try { numberInputted = stdin.nextFloat(); checkBool = true; } catch (Exception e) { System.out.println("Invalid Input. " + e + " Please try again"); } finally { stdin.nextLine(); } } return numberInputted; }
//checks if the string inputted is a float //no longer used in this program public boolean checkIfFloat(String s) { char[] c = s.toCharArray(); for (int z = 0; z < c.length; z++) { if (c[z] > '9' && c[z] != '.' || c[z] < '0' && c[z] != '.') { throw new NumberFormatException(); } } return true; }
//lists all accounts public void listAllAccounts() {
Object[] objArray = accountList.toArray();
System.out.println ("The total number of accounts is " + objArray.length);
if (objArray.length == 0) { return; }
objArray = insertionSortInArrayList(objArray);
Account[] accArray = insertionSortInArrayList(objArray); for (int i = 0; i < objArray.length; i++) { if (objArray[i] instanceof Account) { Account acc = (Account) objArray[i];
//if (acc.getAccountType() == 1) //{ //SavingsAccount sacc = (SavingsAccount) acc; //sacc.addMonthlyInterest(); //} System.out.println(acc.getAccountSummary()); } } }
//insertion sort code. inputs an object array //returns a sorted account array private Account[] insertionSortInArrayList(Object[] objArray) { Account[] accArray = new Account[objArray.length]; for (int z = 0; z < objArray.length; z++) { accArray[z] = (Account) objArray[z]; } for (int i = 1; i < accArray.length; i++) { Account temp = accArray[i]; int j; for (j = i - 1; j >= 0 && accArray[j].compareTo(temp) > 0; j--) { accArray[j + 1] = accArray[j]; } accArray[j + 1] = temp; } return accArray; }
//TestBed Main public static void main(String[] args) { BankAccountManagement bam = new BankAccountManagement(); if (bam.checkIfNumber("111")) { System.out.println ("checkIfNumber method passed with numerical input!"); System.out.println(); } else { System.out.println ("checkIfNumber method failed with numerical input!"); System.out.println(); }
if (!bam.checkIfNumber("test")) { System.out.println ("checkIfNumber method passed with non-numerical input!"); System.out.println(); } else { System.out.println ("checkIfNumber method failed with non-numerical input!"); System.out.println(); }
if (bam.ssnValid("111-11-1111")) { System.out.println("ssnValid method passed!"); } else { System.out.println("ssnValid method failed!"); } System.out.println();
String ssn = "7777777"; Customer c1 = new Customer("Tony Stark", ssn); bam.customerList.add(c1); if (bam.ssnExists(ssn)) { System.out.println("ssnExists method has passed!"); } else { System.out.println("ssnExists method has failed!"); } System.out.println();
if (isIdValid("12345")) { System.out.println("isIdValid method has passed"); } else { System.out.println("isIdValid method has failed!"); } System.out.println();
String acc_name = "Sherlock Holmes"; int acc_num = 17171717; int acc_type = 2; SavingsAccount sa = new SavingsAccount(acc_name, acc_num, acc_type); bam.accountList.add(sa); Account acc = bam.getAccountFromNumber(acc_num);
if (acc.equals(sa)) { System.out.println("getAccountFromNumber method has passed!"); } else { System.out.println("getAccountFromNumber method has failed!"); } System.out.println();
if (bam.checkIfAccountExists(acc_num)) { System.out.println("checkIfAccountExists method has passed!"); } else { System.out.println("checkIfAccountExists method has failed!"); } System.out.println();
if (bam.checkIfFloat("7.7")) { System.out.println("checkIfFloat method has passed!"); } else { System.out.println("checkIfFloat method has failed!"); } System.out.println(); }
}
-------------------------------------------------------------------------
package Pro; import java.text.DecimalFormat;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * */ public class CheckingAccount extends Account {
public CheckingAccount(String name, int accNumber, int accType) { super(name, accNumber, accType); }
@Override public int returnAccountNumber() { return accountNumber; }
@Override public float getAccountBalance() { return balance; }
@Override public String getAccountSummary() { DecimalFormat f = new DecimalFormat("##.##");
String toReturn = ("Account holder is " + accountHolderName + "; Account number is " + accountNumber + "; Account type is checking" + "; Account balance is " + f.format(balance));
return toReturn; }
//TestBed public static void main(String[] args) { String acc_name = "Sherlock Holmes"; int acc_num = 17171717; int acc_type = 2; SavingsAccount sa = new SavingsAccount(acc_name, acc_num, acc_type);
if (sa.returnAccountNumber() == acc_num) { System.out.println("Constructor has passed!"); System.out.println("returnAccountNumber method has passed!"); System.out.println(); } else { System.out.println("Constructor has failed!"); System.out.println("returnAccountNumber method has failed!"); System.out.println(); }
if (sa.getAccountBalance() == 0.0) { System.out.println("Constructor has passed!"); System.out.println("getAccountBalance method has passed!"); System.out.println(); } else { System.out.println("Constructor has failed!"); System.out.println("getAccountBalance method has failed!"); System.out.println(); }
String acc_str = "Account holder is " + acc_name + "; Account number is " + acc_num + "; Account type is checking" + "; Account balance is 0";
if (sa.getAccountSummary().equals(acc_str)) { System.out.println("getAccountSummary method has passed!"); System.out.println(); } else { System.out.println("getAccountSummary method has failed!"); System.out.println(); }
SavingsAccount sa_2 = new SavingsAccount(acc_name, acc_num, acc_type); if (sa.equals(sa_2)) { System.out.println("Account equals method has passed!"); System.out.println(); } else { System.out.println("Account equals method has failed!"); System.out.println(); }
sa.deposit(111); if (sa.getAccountBalance() == 111.0) { System.out.println("Account deposit method has passed!"); System.out.println("getAccountBalance method has passed!"); System.out.println(); } else { System.out.println("Account deposit method has failed!"); System.out.println("getAccountBalance method has failed!"); System.out.println(); }
sa.withdraw(61); if (sa.getAccountBalance() == 50.0) { System.out.println("Account withdraw method has passed!"); System.out.println("getAccountBalance method has passed!"); System.out.println(); } else { System.out.println("Account withdraw method has failed!"); System.out.println("getAccountBalance method has failed!"); System.out.println(); }
if (sa.compareTo(sa_2) == 1) { System.out.println("Account compareTo method has passed!"); System.out.println(); } else { System.out.println("Account compareTo method has failed!"); System.out.println(); } } }
-----------------------------------------------
import java.text.DecimalFormat;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ /** * */ public class SavingsAccount extends Account {
//savings account brings interest, a checking account does not. private final float annualInterestRate = 0.01f;
public SavingsAccount(String name, int accNumber, int accType) { super(name, accNumber, accType); }
public void addMonthlyInterest() //updates balance { for (int i = 0; i < 6; i++) { balance = balance * ((annualInterestRate / 12) + 1); } }
@Override public int returnAccountNumber() { return accountNumber; }
@Override public float getAccountBalance() { return balance; }
@Override public String getAccountSummary() { addMonthlyInterest();
DecimalFormat f = new DecimalFormat("##.##");
String toReturn = ("Account holder is " + accountHolderName + "; Account number is " + accountNumber + "; Account type is savings" + "; Account balance is " + f.format(balance));
return toReturn; }
//TestBed public static void main(String[] args) { String acc_name = "Tony Stark"; int acc_num = 7777777; int acc_type = 1;
SavingsAccount sa = new SavingsAccount(acc_name, acc_num, acc_type);
if (sa.returnAccountNumber() == acc_num) { System.out.println("Constructor has passed!"); System.out.println("returnAccountNumber method has passed!"); System.out.println(); } else { System.out.println("Constructor has failed!"); System.out.println("returnAccountNumber method has failed!"); System.out.println(); }
if (sa.getAccountBalance() == 0.0) { System.out.println("Constructor has passed!"); System.out.println("getAccountBalance method has passed!"); System.out.println(); } else { System.out.println("Constructor has failed!"); System.out.println("getAccountBalance method has failed!"); System.out.println(); }
String acc_str = "Account holder is " + acc_name + "; Account number is " + acc_num + "; Account type is savings" + "; Account balance is 0"; if (sa.getAccountSummary().equals(acc_str)) { System.out.println("getAccountSummary method has passed!"); System.out.println(); } else { System.out.println("getAccountSummary method has failed!"); System.out.println(); } } }
------------------------------
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * * */ public class Prog5 {
//runs BankAccountManagement public static void main(String[] args) { BankAccountManagement bam = new BankAccountManagement(); bam.run(); }
}
--------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
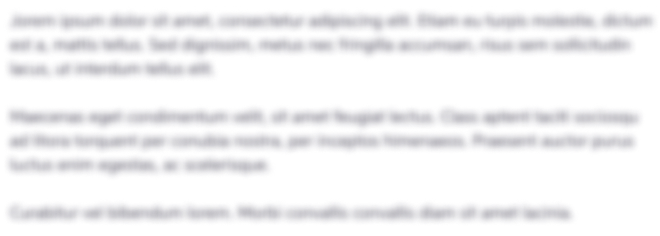
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started