Question
I need help editing my former project 1. output ./stocks stocks.csv needs to look like this but with example below, and need to do this
I need help editing my former project
1. output ./stocks stocks.csv needs to look like this but with example below, and need to do this with a dynamic allocation memory 2-d array. needs the read any csv file, so I can add my csv file to it please. This should be on an excel spread sheet
Date Open Close High Low Volume
------------------------------------------------------------------------------------------------------------------
10-7-2016 Min ### ### ### ### ###
-------------------------------------------------------------------------------------------------------------------
Max
---------------------------------------------------------------------------------------------------------------------
Average
-----------------------------------------------------------------------------------------------------------------------
Median
----------------------------------------------------------------------------------------------------------------------
Stdev
--------------------------------------------------------------------------------------------------------------------
Here is the algorithm thats needs to be followed
This require me to add a csv file to this require to read the rows and columns
All the files below should not be edited except stocks.c and common.h
Please show results before anwsering
Here is the code
stocks.c
#include
#include
#include "io.h"
#include "stats.h"
#include "utils.h"
#include "common.h"
// this function counts the number of columns and valid lines in
// fname and assigns then to cols and rows, respectively
void get_num_lines(const char *fname, const char delim, int *rows, int *cols);
// this function assigns the stock values and volume to their respective arrays
// you need to make sure you're not parsing blank lines. use rows and cols as
// an extra safety measure to prevent you from accessing unallocated memory
void get_1d_array_values(const char *fname, float *open, float *close, float *high, float *low, float *volume, char delim, int rows, int cols);
// the function assigns the stock values and dates to 2d-arrays. stocks_array should have a size
// columns x rows. volume should be included in stocks_array and treated as a float since all the
// statistics done on the volume array will be of type float
void get_2d_array_values(const char *fname, float **stocks_array, char **date, char delim, int rows, int cols);
// this function takes in a stats_array of structures containing statistics for each of the data
// arrays in our file: open, high, low close, volume and prints a pretty-formatted tabled with the results
// like the one shown in the project writeup
void print_stats_table(const Stats *stats_array, int size);
int main(int argc, char* argv[])
{
int size, i;
char delim = ',';
// structure that holds the stats for an array
Stats stats,
*stats_array;
// individual arrays that hold the stock values
float *stocks, // stock values from options 1-4
*open, // open stock price from option 5
*close, // close stock price from option 5
*high, // high stock price from option 5
*low, // low stock price from option 5
*volume; // trade volume of stock from option 5
char **date; // array of strings to hold the date from option 5
float **stocks_array; // 2d array to hold the open, close, high, low , & volume stock prices from option 5
int option = 0, rows = 0, cols = 0;
FILE *fname;
if (argc == 1)
{
// greet and get the stock values
print_greeting();
printf("How many stocks prices would you like to analyze? ");
scanf("%d", &size);
stocks = (float *) malloc(size * sizeof(float));
if(stocks == NULL)
{
printf("Error! Cannot allocate memory for stocks - Exiting... ");
exit(0);
}
read_array(stocks, size);
option = 1
}
else if (argc == 2)
{
char *ptr = NULL;
ptr = strchr(argv[1], delim);
// does the string contain the delimiter???
if (ptr == NULL) // this is case $ ./stocks.x 4
{
size = atoi(argv[1]);
stocks = (float *) malloc(size * sizeof(float));
if(stocks == NULL)
{
printf("Error! Cannot allocate memory for stocks - Exiting... ");
exit(0);
}
read_array(stocks, size);
option = 1;
}
else // this is case $ ./stocks 1,2,3,4
{
//
size = get_num_tokens(argv[1], delim);
stocks = (float *) malloc(size * sizeof(float));
if(stocks == NULL)
{
printf("Error! Cannot allocate memory for stocks - Exiting... ");
exit(0);
}
get_tokens_array(argv[1], stocks, size, delim);
option = 1;
}
}
else if (argc > 2) // this is case $ ./stocks.x 1 2 3 4
{
// ignore the executable
size = argc - 1;
stocks = (float *) malloc(size * sizeof(float));
if(stocks == NULL)
{
printf("Error! Cannot allocate memory for stocks - Exiting... ");
exit(0);
}
// assign all the values but the executable to the array
for (int i = 0; i
stocks[i] = atof(argv[i+1]);
option = 1;
}
if (option == 1)
{
// get stats and print results
get_stats(&stats, stocks, size);
print_results(&stats, stocks, size);
// free memory
free(stocks);
}
return 0;
}
void get_num_lines(const char *fname, char delim, int *rows, int *cols)
{
}
void get_1d_array_values(const char *fname, float *open, float *close, float *high, float *low, float *volume, char delim, int rows, int cols)
{
}
void get_2d_array_values(const char *fname, float **stocks_array, char **date, char delim, int rows, int cols)
{
}
void print_stats_table(const Stats *stats_array, int size)
{
}
io.c
#include
// prompt the user for input and read the values into an array void read_array(float *array, int size) { int i = 0; for (i = 0; i
// say hi to the user void print_greeting(void) { printf("Hello this program will ask for your stock prices and do calcuations to them. "); }
// display array values void print_array(const float array[], int size) { int i = 0;
for (i = 0; i
printf(" ");
}
// print the stat results including input data void print_results(const Stats *stats, const float array[], int size) {
printf(" Say something here about the ouput "); print_array(array, size); print_stats(stats);
}
// print the stat results including input data void print_stats(const Stats *stats) {
printf(" Here are the results: "); printf("%-10s $%.2f ", "min:", stats->min); printf("%-10s $%.2f ", "max:", stats->max); printf("%-10s $%.2f ", "mean:", stats->mean); printf("%-10s $%.2f ", "stddev:", stats->stddev); printf("%-10s $%.2f ", "median:", stats->median);
}
io.h
#ifndef IO_H #define IO_H
void read_array(float array[], int size); void print_greeting(void); void print_array(const float array[], int size); void print_results(const float array[], int size, float median, float min, float max, float mean, float variance);
#endif
stats.c
#include
#include "stats.h"
// sorts the values of an array according to order
void sort (float output[], const int size, char order)
{
int i, j;
float temp;
if (order == 'a' || order == 'A')
{
for ( i = 0; i
for ( j = i + 1; j
if ( output[i] > output[j] )
{
temp = output[i];
output[i] = output[j];
output[j] = temp;
}
}
else if (order == 'd' || order == 'D')
{
for ( i = 0; i
for ( j = i + 1; j
if ( output[i]
{
temp = output[i];
output[i] = output[j];
output[j] = temp;
}
}
else
return;
}
// calculates the mean of the elements of an array
float get_average(const float array[], int size)
{
int i;
float sum = 0.0;
for (i = 0; i
sum += array[i];
sum /= size;
return sum;
}
// calculates the variance of the emelemts of an array
// this function calls the get_average to get the mean
float get_variance(const float array[], int size)
{
int i;
float sum = 0.0;
float mean = get_average(array, size);
for (i = 0; i
sum += array[i] * array[i];
sum = sum/size - mean*mean;
return sum;
}
// gets the median of an array after it sorts it
float get_median(const float array[], int size)
{
int i;
float temp_array[size]; // temp array tp be manipulated
float median;
// copy oroginal array to the temp array
for (i = 0; i
temp_array[i] = array[i];
sort(temp_array, size, 'a');
if (size % 2 == 0)
median = (temp_array[size/2] + temp_array[size/2-1])/2.0;
else
median = temp_array[size/2];
return median;
}
// finds the maximum value of the elements of an array
float get_max(const float array[], int size)
{
int i;
float max = array[0];
for (i = 0; i
if (array[i] >= max)
max = array[i];
return max;
}
// finds the minimum value of the elements of an array
float get_min(const float array[], int size)
{
int i;
float min = array[0];
for (i = 0; i
if (array[i]
min = array[i];
return min;
}
stats.h
#ifndef STATS_H #define STATS_H
void sort (float output[], int size, char order); float get_average(const float array[], int size); float get_variance(const float array[], int size); float get_median(const float array[], int size); float get_max(const float array[], int size); float get_min(const float array[], int size);
#endif
utils.c
#include
int get_num_tokens(const char str[], char delim)
{
int i = 0,count = 1;
while (str[i] != '\0')
{
if (str[i++] == delim)
count++;
}
return count;
}
int get_tokens_array(const char str[], float array[], float size, char delim) { int tokens = get_num_tokens(str, delim); char temp[20]; int count = 0, count1 = 0, i = 0; while (str[i] != 0) { if (str[i] == delim) { if (count1 0) { array[count1++] = atof(temp); count = 0; } } } else { if (str[i] != ' ') { temp[count++] = str[i]; } } ++i; } if (count1 0) { array[count1++] = atof(temp); } return count1; }
utils.h
#ifndef UTILS_H #define UTILS_H
int get_num_tokens(const char str[], char delim); void get_tokens_array(const char str[], float array[], float size, char delim);
#endif
common.h
#ifndef COMMON_H #define COMMON_H
typedef struct { float min, max, mean, stddev, median; } Stats;
#endif
Please let me know if there is anything needed. Thank you
legin er the mber af data an cs slu il hol lalso xpulare thSzccure one parlis a tim foreach urrny chen as ilna:Iala in 1hc Ile-11r 'JW2 UI Uh; Jti.fi atay b:1rcsat. Ilx coluimas in leCl Lx he same ol in a simila fashion ther print he eslsi ubl sures x uve pop.lale i farmatred tahles of the resalts lise Volure Open Close High
Step by Step Solution
There are 3 Steps involved in it
Step: 1
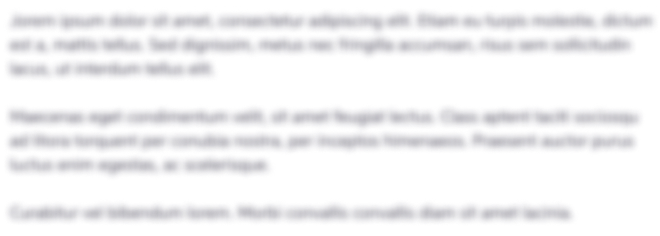
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started