Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I need help fixing my Android App to display Area and Perimter results to only 2 Decimal Places.I also need my app to display the
I need help fixing my Android App to display Area and Perimter results to only 2 Decimal Places.I also need my app to display the Results Area and Perimeter in TextView and not EditView like I have it now. Pease show me how.
//RectangleCalculator app
package com.example.ryan_.rectanglecalculatorapp; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import static java.lang.Math.*; public class MainActivity extends AppCompatActivity { //Defining the widgets EditText widthInput, heightInput, areaResult, perimeterResult; Button button; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); //This is used to set the activity laypout for the app setContentView(R.layout.activity_main); //Using @+idNumber tags to get and set values widthInput = (EditText) findViewById(R.id.Number1); heightInput = (EditText) findViewById(R.id.Number2); areaResult = (EditText) findViewById(R.id.Area); perimeterResult = (EditText)findViewById(R.id.Perimeter); button = (Button)findViewById(R.id.button); //Set the listner to the button button.setOnClickListener(new View.OnClickListener(){ public void onClick(View v){ //Take the user input and calculate the recatnagle features float width = Float.valueOf(widthInput.getText().toString()); float height = Float.valueOf(heightInput.getText().toString()); final float area = width * height; final float perimeter = 2* width + 2 * height; //Display the results areaResult.setText(Float.toString(area)); perimeterResult.setText(Float.toString(perimeter)); } }); } }
//activity_main.xml
xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="24dp" android:text="@string/width" android:textStyle="bold" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/Number1" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.154" /> <EditText android:id="@+id/Number1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:inputType="numberDecimal" app:layout_constraintBottom_toTopOf="@+id/Number2" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toEndOf="@+id/textView3" app:layout_constraintTop_toTopOf="parent" /> <TextView android:id="@+id/textView4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/height" android:textStyle="bold" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/Number2" app:layout_constraintHorizontal_bias="0.482" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.363" /> <EditText android:id="@+id/Number2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:inputType="numberDecimal" app:layout_constraintBottom_toTopOf="@+id/Area" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.739" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/Number1" /> <EditText android:id="@+id/Perimeter" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:inputType="numberDecimal" app:layout_constraintBottom_toTopOf="@+id/button" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.59" app:layout_constraintStart_toEndOf="@+id/TextView4" app:layout_constraintTop_toBottomOf="@+id/Area" app:layout_constraintVertical_bias="0.78" /> <EditText android:id="@+id/Area" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:inputType="numberDecimal" app:layout_constraintBottom_toTopOf="@+id/Perimeter" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.435" app:layout_constraintStart_toEndOf="@+id/TextView3" app:layout_constraintTop_toBottomOf="@+id/Number2" /> <TextView android:id="@+id/TextView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Area" android:textStyle="bold" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/Area" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.563" /> <TextView android:id="@+id/TextView4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Perimeter" android:textStyle="bold" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toStartOf="@+id/Perimeter" app:layout_constraintHorizontal_bias="0.5" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.737" /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/calculate" android:textStyle="bold" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.501" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.965" /> android.support.constraint.ConstraintLayout>
Step by Step Solution
There are 3 Steps involved in it
Step: 1
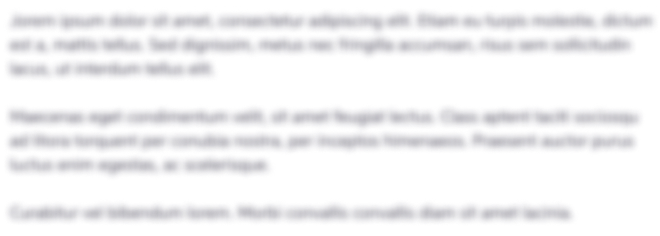
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started