Question
I need help fixing my code for this problem: Complete the following programming project Rocket......which deals a great deal with functional decomposition. This program is
I need help fixing my code for this problem:
Complete the following programming project "Rocket"......which deals a great deal with "functional decomposition".
This program is broken down into phases for your convenience only. Please turn in only your final product. Note: You are encouraged to use as much code from lesson#5 notes as you can for this project! DO NOT USE global variables. Phase 1: Write a program that draws a rocket shape on the screen based on user input of three values, height, width and stages. The type of box generated (ie. an outline or filled-in) is based on a check for odd or even values input by the user for the box height (or number of rows). Here is the general specification given user input for the height of the box..... Draw an outline of a box for each stage if the value for the height of the box (or number of rows) is an even number. Draw a filled-in box for each stage if the value for the height of the box (or number of rows) is an odd number. INPUT VALIDATION: Do not accept values less than 3 or greater than 15 for height and width and the input for stages must be a nonnegative value.
ROCKET SAMPLE#1 WITH 2 STAGES ROCKET BODY HAS TWO EVEN HEIGHT BOX SHAPES (i.e., each with height = 6 or number of rows = 6) NOTE THAT THE BOX SHAPE HAS A CARGO BAY THAT IS EMPTY * * * * * ***** < top row * * * * <= box outline for stage#1 * * * * ***** < bottom row ***** < top row * * * * <= box outline for stage#2 * * * * ***** < bottom row * * * * * | ROCKET SAMPLE#2 WITH 2 STAGES ROCKET BODY HAS TWO ODD HEIGHT BOX SHAPES (i.e., each with height = 7 or number of rows = 7) NOTE THAT THE BOX SHAPE HAS A CARGO BAY THAT IS FILLED * * * * * ***** < top row ***** ***** ***** <= box filled for stage#1 ***** ***** ***** |
Your main function in phase 1 may look like either of these:
function decomposition #1 - seperate functions to generate an outline or filled in box shape void drawCone();//function prototype void drawEvenBox(); //function prototype void drawOddBox(); //function prototype | functional decomposition#2 - one drawBox function but with procedures within the functions to generate both an outline and a filled in box shape void drawCone();//function prototype void drawBox(); //function prototype | |
int main() { //functions for sample#1 drawCone(); drawEvenBox(); drawEvenBox(); drawCone(); //functions for sample#2 drawCone(); drawOddBox(); drawOddBox(); drawCone(); } //define functions below drawCone(){ } drawEvenBox(){ } drawOddBox(){ } | int main() { //functions for sample#1 and sample#2 drawCone(); drawBox(); //procedures within the function to generate both box shapes drawCone(); } //define functions below drawCone(){ } drawBox(){ } |
The functions in this phase do not necessarily need external data. You may use simple cout statements (no loops are necessary) in your drawCone function, but you must write your drawBox function or the drawEvenBox and drawOddBox functions using loops. For example, you can set-up a drawBox function with a procedure within it that uses nested loops to generate a 5 x 6 or a 5 x 7 box shape as shown in the sample figures above.You may not use the setw() function in this project! Phase 2: In this phase inside int main() you will allow the user to specify three things and validate input to make sure that the values are not less than 3 or greater than 15. If needed, allow the user to re-input values that meet the program specification.: 1. The height of each stage 2. The width of each stage 3. How many stages in the rocket [A "stage" in your rocket is one box] Your program will look a lot like it did before except that you will add 3 cout/cin statements including a data validation routine before you call the functions. In addition, you will now have some arguments in your function calls, and some other changes to call the appropriate functions (HINT: based on the height of each stage) to generate the correct type of rocket shape. Notice that in addition to generating two possible rocket types (based on stage height) if you run the program and choose a different width for your stages, the cone won't really fit correctly anymore. I won't make you fix this, but you can fix it for 10 points of extra credit if you like. However, I will not help you with the extra credit. In order to get the extra credit, the number of rows of your cone must be equal to the width of the stages divided by 2 (plus 1 for odd widths). If the stage width is an even number, your cone must have two stars in the top row instead of one. If you do this perfectly and use good decomposition in the process you'll get 10 extra credit points. Phase 3: In this phase you won't change what the program produces. We're just going to improve on the organization a bit. Change your program so that the main function looks like this: (note: no function prototypes are shown below)
int main() { |
Please make sure to place a comment near each of your function definitions, use good decomposition, separate your functions with at least 1 inches of whitespace and DO NOT use global variables. I suggest that now would be a good time to reread the How To Get Good Grades on Your Programs and Function Documentation: Assertions and Dataflow comments. Note: Projects submitted without appropriate function related documentation will not be graded and you will need to resubmit your source code including all function related documentation.
--------------------
Here is my code so far. It runs, but doesn't do anything.
//Project 1 - Rocket
#include
void getDimensions (int &width, int &height, int &stages); //User input on rocket size void drawRocket (int width, int height, int stages); void drawCone (int width, int stages); void drawBox (int width, int height, int stages); void drawStageWidth (int numStars); //Top and Bottom of Stage void drawStageSides (int numSpaces, int numRows); //Sides of stage void drawOneRow (int numSpace); //Rows of stage with whitespace?
int main() { int width, height, stages; //Determine whether stage is even (outline) or odd (filled). while (height >= 3 && height <= 15 && width >= 3 && width <= 15) { getDimensions (width, height, stages); drawRocket (width, height, stages); } }
void getDimensions (int &width, int &height, int &stages) { cout << "Enter width: "; cin >> width; cout << "Enter height: "; cin >> height; cout << "Enter number of stages: "; cin >> stages; cout << endl; }
void drawRocket (int width, int height, int stages) { drawBox (width, height, stages); }
void drawBox (int height, int width, int stages) { //Hollow Box if (height %2 == 0) { drawStageWidth(width); drawStageSides(width - 2, height - 2); drawStageWidth(width); } //Filled Box else { for (int row = 0; row < width; row++) for (int column = 0; column < height; column++) { cout << '*'; } cout << endl; }
}
void drawStageWidth(int numStars) { int count; for (count = 0; count < numStars; count++) { cout << "*"; } cout << endl; }
void drawStageSides(int numSpaces, int numRows) { int rowCount; for (rowCount = 0; rowCount < numRows; rowCount++) { drawOneRow(numSpaces); } }
void drawOneRow(int numSpaces) { int spaceCount; cout << "*"; for (spaceCount = 0; spaceCount < numSpaces; spaceCount++) { cout << " "; } cout << "*" << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
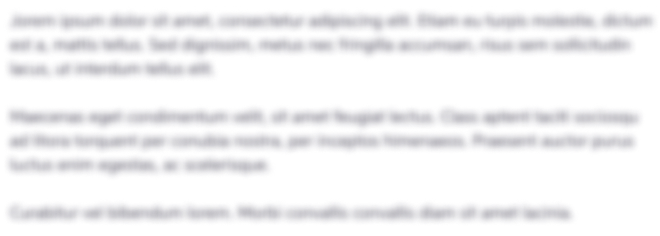
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started