Question
I need help fixing my ShoppingCartManager.java. I can't loop the menu import java.util.Scanner; public class ShoppingCartManager { public static void main(String[] args){ //prompt the user
I need help fixing my ShoppingCartManager.java. I can't loop the menu
import java.util.Scanner;
public class ShoppingCartManager { public static void main(String[] args){ //prompt the user for a customer's name and today's date Scanner scan = new Scanner(System.in); System.out.println("Enter Customer's Name: "); String customerName = scan.nextLine(); System.out.println("Enter Today's Date: "); String currentDate = scan.nextLine(); ShoppingCart cart = new ShoppingCart(customerName, currentDate); System.out.println(); //space //Output the name and date System.out.println("Customer Name: " + cart.getCustomerName()); System.out.println("Today's Date: " + currentDate); } public static void printMenu(ShoppingCart shoppingCart, Scanner scan){ //string of menu String menu = " MENU " + "a - Add item to cart " + "d - Remove item from cart " + "c - Change item quantity " + "i - Output items' descriptions " + "o - Output shopping cart " + "q - Quit Choose an option:"; Scanner scanner = new Scanner(System.in); //User chooses option char input = scanner.next().charAt(0); scanner.nextLine(); //user input //Add item to cart if(input == 'a'){ System.out.println("ADD ITEM TO CART"); System.out.println("Enter Item Name: "); String itemName = scanner.nextLine(); System.out.println("Enter Item Description: "); String itemDescritpion = scanner.nextLine(); System.out.println("Enter Item Price: "); int itemPrice = scanner.nextInt(); System.out.println("Enter Item Quantity: "); int quantity = scanner.nextInt(); scanner.nextLine(); } //Remove item from cart else if(input == 'd'){ System.out.println("REMOVE ITEM FROM CART"); System.out.println("Enter name of item to remove: "); String name = scanner.nextLine(); } //Change item quantity else if(input == 'c'){ System.out.println("CHANGE ITEM QUANTITY"); System.out.println("Enter the item name: "); String name = scanner.nextLine(); System.out.println("Enter the new quantity: "); int quantity = scanner.nextInt(); ItemToPurchase item = new ItemToPurchase(); item.setName(name); item.setQuantity(quantity); } //Output items' descriptions else if(input == 'i'){ System.out.println("OUTPUT ITEMS' DESCRIPTIONS"); } //Output shopping cart else if(input == 'o'){ System.out.println("OUTPUT SHOPPING CART"); } //Quit else if(input == 'q'){ } else { System.out.println(menu); } } }
here is ItemsToPurchase.java
public class ItemToPurchase { //Private fields private String itemName; private String itemDescription; private int itemPrice; private int itemQuantity; public ItemToPurchase() { //Default constructors this.itemDescription = "none"; this.itemName = "none"; this.itemQuantity = 0; this.itemPrice = 0; } //Parameterized constructor to assign name,descptn, price, quanitity public ItemToPurchase(String itemName, String itemDescription, int itemPrice, int itemQuantity) { //"this" refers to the object that is calling the method in the first place/reference this.itemDescription = itemDescription; this.itemName = itemName; this.itemPrice = itemPrice; this.itemQuantity = itemQuantity; } //Public member methods public String itemDescription() { return itemDescription; } //Outputs the item name followed by the quantity, price, and subtotal public void printItemCost() { System.out.println(itemName + " " + itemQuantity + " @ " + itemPrice + " = $" + (itemQuantity * itemPrice)); } public void printItemDescription() { System.out.println(itemName + " " + itemDescription); } public void setDescription(String itemDescription) { this.itemDescription = itemDescription; } public String getDescription(){ return itemDescription; } public String getName() { return itemName; } public void setName(String itemName) { this.itemName = itemName; } public int getPrice() { return itemPrice; } public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } public int getQuantity() { return itemQuantity; } public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } }
here is ShoppingCart.java
import java.util.ArrayList; import java.util.Scanner;
public class ShoppingCart { //Private fields private String customerName; private String currentDate; private ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
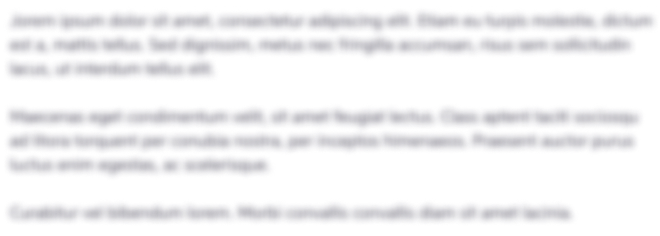
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started