Question
I need help fixing these errors in my code. Basically it deletes spaces from the beginning of the sentence in FileIn.txt and counts the #
I need help fixing these errors in my code. Basically it deletes spaces from the beginning of the sentence in FileIn.txt and counts the # of spaces deleted (only shows # 0) and displays it at beginning of the sentence but it's not showing the correct # of spaces being deleted please help. (And it outputs at the FileOut.txt)
Main Class:
class Main {
public static void main(String[] args) {
Code fe = new Code();
fe.readWrite("FileIn.txt","FileOut.txt");
}
}
Squeeze Class:
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class Squeeze {
// this line was missing in the original code
public void readWrite(String fileIn, String fileOut) {
// Read file using scanner
Scanner sc;
// Write file using writer
FileWriter writer;
try
{
sc = new Scanner(new File(fileIn));
writer = new FileWriter(new File(fileOut));
while (sc.hasNext()) {
int tabCount = 0;
String line = sc.nextLine();
for (int i = 0; i < line.length(); i++) {
if (line.charAt(i) == '\t') {
tabCount++;
} else {
break;
}
}
// Write line
writer.write(tabCount + " " + line.trim());
writer.write(" ");
}
writer.close();
} catch(FileNotFoundException e){
e.printStackTrace();
} catch(IOException e) {
e.printStackTrace();
}
}
}
FileIn.txt:
public class Fraction {
private int numerator; private int denominator;
public Fraction() {
numerator = 0; denominator = 0;
}
// returns the denominator when called.
public int getDenominator() { return this.denominator;
}
// returns the numerator when called.
public int getNumerator() { return this.numerator;
}
public Fraction(int numerator, int denominator) { this.numerator = numerator; this.denominator = denominator; }
// Returns the string representation of the object with conditional statements.
public String toString() {
simplify(); // used simplify() method before printing
int d = denominator; int n = numerator; if (d == 0) { return "DNE"; } else if (d == 1 && n != 0) { return "" + n; } else if (n == 0) { return "0"; }
return "(" + this.numerator + "/" + this.denominator + ")";
}
// overloaded method that uses two conditional statements if the denominator of the two fractions being subtracted is different and does the regular subtraction operation if it's the same. It also creates a new intance of Fraction and stores its values in the numerator and denominator.
public Fraction sum(Fraction other) { if (denominator != other.getDenominator()) { int SumN = numerator * other.getDenominator() + this.denominator * other.getNumerator(); int SumD = denominator * other.getDenominator(); return new Fraction(SumN, SumD);
} else {
int SumNum = numerator + other.getNumerator(); int SumDen = denominator; return new Fraction(SumNum, SumDen);
}
}
// overloaded method that uses two conditional statements if the denominator of the two fractions being subtracted is different and does the regular subtraction operation if it's the same. It also creates a new intance of Fraction and stores its values in the numerator and denominator.
public Fraction diff(Fraction other) {
if (denominator != other.getDenominator()) { int DiffN = numerator * other.getDenominator() - this.denominator * other.getNumerator(); int DiffD = denominator * other.getDenominator(); return new Fraction(DiffN, DiffD);
} else {
int DiffNum = numerator - other.getNumerator(); int DiffDen = denominator; return new Fraction(DiffNum, DiffDen);
}
}
// overloaded method that multiplies numerator to other.getNumerator() and denominator to getDenominator(). It also creates a new intance of Fraction and stores its values in the numerator and denominator.
public Fraction mult(Fraction other) {
int MultNum = numerator * other.getNumerator(); int MultDen = denominator * other.getDenominator(); return new Fraction(MultNum, MultDen);
}
// overloaded method that multiplies the recipricol numerator to other.getDenominator() and denominator to getNumerator(). It also creates a new intance of Fraction and stores its values in the numerator and denominator.
public Fraction div(Fraction other) {
int DivNum = numerator * other.getDenominator(); int DivDen = denominator * other.getNumerator(); return new Fraction(DivNum, DivDen);
}
// simplifies the numerator and denominator to the lowest terms. private void simplify() { int gcd = 1; int num; int den; if(numerator<0) num = -numerator; else{ num = numerator; } if (denominator<0) den = -denominator; den = denominator; for(int f = 2; f<=num && f<=den ; f++){ if(num%f==0 && den%f==0) gcd = f; } numerator/=gcd; denominator/=gcd;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
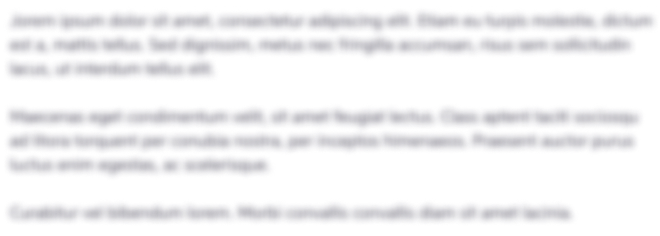
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started