Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I need help for this code to pass these test specs class LinkedListNode { constructor(value, next) { this.value = value; this.next = next; } }
I need help for this code to pass these test specs
class LinkedListNode { constructor(value, next) { this.value = value; this.next = next; } } class LinkedList { constructor() { // Default to empty this.head = null; this.tail = null; this.length = 0; } enqueue(value) { const newNode = new LinkedListNode(value); if (!this.head) { this.head = newNode; this.tail = newNode; } else { this.tail.next = newNode; this.tail = newNode; } this.length++; } dequeue() { if (!this.head) { return null; } const returnValue = this.head.value; this.head = this.head.next; if (!this.head) { this.tail = null; } this.length--; return returnValue; } print() { let current = this.head; while (current) { process.stdout.write(`${current.value} -> `); current = current.next; } console.log("NULL"); } } class User { constructor (name) { this.name = name; } } class UserRegistration { constructor (maxUsers) { this.users = []; this.nameList = []; this.maxUsers = maxUsers; } registerUser(username) { if (this.nameList.indexOf(username) === -1) { const newUser = new User(username); this.users.push(newUser); this.nameList.push(username); if (this.users.length > this.maxUsers) { const userToRemove = this.users.shift(); return(`${userToRemove.name} unregistered, ${username} registered`); } return(`${username} registered`); } else { return(`${username} failed to register`); } } } module.exports = { LinkedList, UserRegistration };
const chai = require('chai'); const expect = chai.expect; const { LinkedList, UserRegistration } = require('../problems/user-registration.js'); describe('UserRegistration implementation', function() { it ('registerUser registers unique usernames', function() { const registration = new UserRegistration(3); expect(registration.registerUser('User 1')).to.eql('User 1 registered'); expect(registration.registerUser('User 2')).to.eql('User 2 registered'); expect(registration.registerUser('User 3')).to.eql('User 3 registered'); }); it ('registerUser fails to register a repeat username', function() { const registration = new UserRegistration(3); expect(registration.registerUser('User 1')).to.eql('User 1 registered'); expect(registration.registerUser('User 1')).to.eql('User 1 failed to register'); }); it ('registerUser deregisters a username if maximum user count is reached', function() { const registration = new UserRegistration(3); expect(registration.registerUser('User 1')).to.eql('User 1 registered'); expect(registration.registerUser('User 2')).to.eql('User 2 registered'); expect(registration.registerUser('User 3')).to.eql('User 3 registered'); expect(registration.registerUser('User 4')).to.eql('User 1 unregistered, User 4 registered'); }); it ('users property is not an Array and is an instance of a Set OR a LinkedList', function () { const registration = new UserRegistration(3); expect(Array.isArray(registration.users)).to.be.false; expect( registration.users instanceof Set || registration.users instanceof LinkedList ).to.be.true; }); it ('nameList property is not an Array and is an instance of a Set OR a LinkedList', function () { const registration = new UserRegistration(3); expect(Array.isArray(registration.nameList)).to.be.false; expect( registration.nameList instanceof Set || registration.nameList instanceof LinkedList ).to.be.true; }); });
const chai = require('chai'); const expect = chai.expect; const { UserRegistration } = require('../problems/user-registration.js'); describe('UserRegistration timing', function() { this.timeout(4000); // The performance expectations for this assessment are measured // relative to this benchmark. It runs in ~200ms on an Intel // MacBook Pro. This system accounts for differences in processor // speed. let timeout = 0; let benchmark = 0; const timingBuffer = 1.0; before (function() { let startTime = Date.now(); // Fill an array with 30000 values const arr = []; for (let i = 0 ; i < 30000; i++) { arr.unshift(i); } benchmark = Date.now() - startTime; timeout = benchmark * timingBuffer; console.log(`nBENCHMARK time is: ${timeout}msn`); }); it(`Registers 10,000 users, max 1,000 within the benchmark time`, function() { const userCount = 10000; const userMax = 1000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); it(`Registers 30,000 users, max 1,000 within the benchmark time`, function() { const userCount = 30000; const userMax = 1000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); it(`Registers 50,000 users, max 1,000 within the benchmark time`, function() { const userCount = 50000; const userMax = 1000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); it(`Registers 80,000 users, max 1,000 within the benchmark time`, function() { const userCount = 80000; const userMax = 1000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); it(`Registers 80,000 users, max 5,000 within the benchmark time`, function() { const userCount = 80000; const userMax = 5000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); it(`Registers 80,000 users, max 10,000 within the benchmark time`, function() { const userCount = 80000; const userMax = 10000; // Timing performance test const registerTime = registerUsersTiming(userCount, userMax, timeout); expect(registerTime).to.be.true; }); }); // Register users, return false if it times out, true otherwise function registerUsersTiming(userCount, userMax, timeout) { reg = new UserRegistration(userMax); startTime = Date.now(); numUsers = userCount; for (let i = 1 ; i <= userCount ; i++) { reg.registerUser(`User ${i}`); if (Date.now() - startTime > timeout) { console.log(`Timeout: Registered ${userCount} - max ${userMax}`); return false; } } console.log(`Registered ${userCount} - max ${userMax} in ${Date.now() - startTime}ms`); return true; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
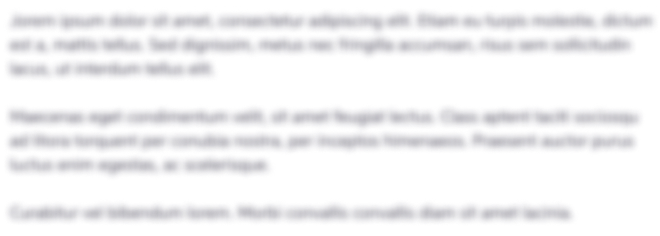
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started