Question
I need help implementing the following methods in the class LinkedStack which implements the stack interface A method with the following header public static boolean
I need help implementing the following methods in the class LinkedStack which implements the stack interface
A method with the following header
public static boolean palindrome(String str)
This method returns true if string is a palindrome or null, otherwise false. A palindrome is a string of characters (a word, phrase, or sentence) that is the same regardless of whether you read it forward or backward - assuming that you ignore spaces, punctuation, and case. For example, Race car is a palindrome. So is A man, a plan, a canal: Panama. You need to use a stack to implement this method.
A method with the following header
public static boolean equals01(String str)
This method returns true if string is composed of equal number of 0s and 1s, or null, otherwise it returns false. Suppose that you read a binary string - that is, a string of 0s and 1s - one character at a time. This method needs to use a stack but no arithmetic to see whether the number of 0s is equal to the number of 1s.
A method with the following header
public static
This method displays a comma separated string value of all the objects in a stack in the order in which they were pushed onto it. After all the objects are displayed, the stack ls should have the same contents as when it was passed into this method. You need to use a stack in this method.
Here is the stack interface
/**
* An interface for the ADT stack.
*/
public interface StackInterface
{
/**
* Adds a new entry to the top of this stack.
* @param newEntry: An object to be added to the stack.
*/
public void push(T newEntry);
/**
* Removes and returns this stack's top entry.
* @return The object at the top of the stack.
* @throws EmptyStackException if the stack is empty before the operation.
*/
public T pop();
/**
* Retrieves this stack's top entry.
* @return The object at the top of the stack.
* @throws EmptyStackException if the stack is empty.
*/
public T peek();
/**
* Detects whether this stack is empty.
* @return True if the stack is empty.
*/
public boolean isEmpty();
/**
* Removes all entries from this stack.
*/
public void clear();
} // end StackInterface
Here is the code for the linked stack class
/**
* A class of stacks whose entries are stored in a chain of nodes.
* @author Darnesha Randle
*/
public class LinkedStack
{
private Node topNode;
public LinkedStack()
{
topNode = null;
}
public void push(T newEntry)
{
Node newNode = new Node(newEntry, topNode);
topNode = newNode;
}
public T peek()
{
if (isEmpty())
{
throw new EmptyStackException();
}
else
{
return topNode.getData();
}
}
public T pop()
{
T top = peek(); // Might throw EmptyStackException
assert (topNode != null);
topNode = topNode.getNextNode();
return top;
}
/**
* Sees whether this bag is empty.
* @return True if this bag is empty, or false if not.
*/
public boolean isEmpty()
{
return topNode == null;
}
/**
* Removes all entries from this bag.
*/
public void clear()
{
topNode = null;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
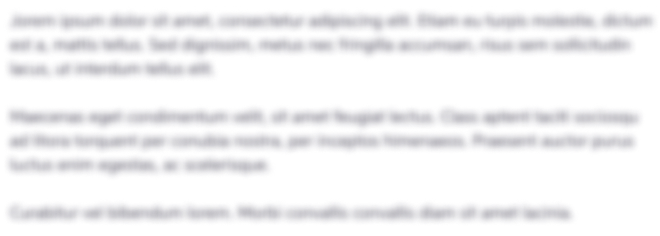
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started