Question
I NEED HELP MAKING THIS PROJECT AND I HAVE TO USE A LINKED LIST AND NOT A ARRAY. I HAVE THE MAIN FILE TO THAT
I NEED HELP MAKING THIS PROJECT AND I HAVE TO USE A LINKED LIST AND NOT A ARRAY.
I HAVE THE MAIN FILE TO THAT RUNS THE PROGRAM, I JUST NEED HELP WITH THE PROGRAMMING PART
THIS IS MY sequence.cpp file
#include "sequence.h" #include "stdafx.h"
Sequence::Sequence(size_type sz) {}
Sequence::Sequence(const Sequence& s) {}
Sequence::~Sequence() {}
Sequence& Sequence::operator=(const Sequence& s) {}
Sequence::value_type& Sequence::operator[](size_type position) {}
Sequence::value_type& Sequence::at(size_type position) {}
void Sequence::push_back(const value_type& value) {}
void Sequence::pop_back() {}
void Sequence::insert(size_type position, value_type value) {}
const Sequence::value_type& Sequence::front() const {}
const Sequence::value_type& Sequence::back() const {}
bool Sequence::empty() const {}
Sequence::size_type Sequence::size() const {}
void Sequence::clear() {}
void Sequence::erase(size_type position, size_type count) {}
ostream& Sequence::print(ostream& os) {} ostream& operator<<(ostream& os, Sequence& s) {}
THIS IS MY sequence.h file
#ifndef SEQUENCE_H #define SEQUENCE_H
#include #include
using namespace std;
class Sequence { // The following declarations describe the functions that you must implement. // You SHOULD NOT CHANGE any of the following PUBLIC declarations. public: typedef int value_type; // data type of sequence items typedef unsigned int size_type; // data type of sequence counts and / or sizes
Sequence(size_type sz = 0); // creates a sequence indexed from 0 ... sz
Sequence(const Sequence& s); // create a sequence from the existing sequence s ~Sequence(); // destroys the sequence
Sequence& operator=(const Sequence& s); // assign sequence s to the sequence
value_type& operator[](size_type p); // return a reference to the item at index position p value_type& at(size_type p); // return a reference to the item at index position p
void push_back(const value_type& v); // add v to the end of the sequence void pop_back(); // remove item at the end of the sequence
void insert(size_type p, value_type v); // insert the item v in the sequence at index position p
const value_type& front() const; // returns a reference to the first item in the sequence const value_type& back() const; // returns a reference to the last item in the sequence
bool empty() const; // returns true if the sequence is empty size_type size() const;
void clear(); // clears the sequence returning it to the empty state void erase(size_type p, size_type n = 1); // deletes n number items starting a index position p
ostream& print(ostream& = cout); // prints the items as a comma seperated sequence
// YOU CAN MODIFY the following private declarations as needed to implement your sequence class private:
// If you choose to use a linked list, use the following // private inner class for linked list nodes // All data elements are public, since only class sequence can see SequenceNodes class SequenceNode { public: SequenceNode * next; SequenceNode *prev; value_type elt;
SequenceNode() { next = nullptr; prev = nullptr; }
SequenceNode(value_type item) { next = nullptr; prev = nullptr; elt = item; }
~SequenceNode() {};
};
// MEMBER DATA. These are the data items that each sequence object will contain. For a // doubly-linked list, each sequence will have a head and tail pointer, and numElts SequenceNode *head; SequenceNode *tail; size_type numElts; // Number of elements in the sequence
}; // End of class Sequence
// You must also implement the << operator. Do not change this declaration: ostream& operator<<(ostream&, Sequence&);
THIS IS SOME OF THE MAIN PROGRAM TO TEST
#include
using namespace std;
void testCopyConstructor(Sequence);
int main() { // Create a sequence of length four, store some values, and print try { cout << "Testing sequence creation and printing" << endl; cout << "--------------------------------------" << endl; Sequence data(4); data[0] = 100; data[1] = 200; data[2] = 300; cout << "Sequence: " << data << endl; cout << "Should be: <100, 200, 300, ???>" << endl << endl; }
catch (exception& e) { cout << "Exception: " << e.what() << endl << endl; }
// Test for independent sequences try { cout << "Testing multiple sequences" << endl; cout << "--------------------------" << endl; Sequence s1(3); Sequence s2(3);
for (int i = 0; i < 3; i++) { s1[i] = i; s2[i] = 100 + i; } cout << "Sequence1: " << s1 << endl; cout << "Sequence2: " << s2 << endl; cout << "Should be: <0, 1, 2>" << endl << " <100, 101, 102>" << endl << endl; }
catch (exception& e) { cout << "Exception: " << e.what() << endl << endl; }
// Test push_back try { cout << "Testing push_back()" << endl; cout << "-------------------" << endl; Sequence data(3); data[0] = 100; data[1] = 200; data[2] = 300; data.push_back(400); data.push_back(500); cout << "Sequence: " << data << endl; cout << "Should be: <100, 200, 300, 400, 500>" << endl << endl; }
catch (exception& e) { cout << "Exception: " << e.what() << endl << endl; }
// Test push_back to an empty sequence try { cout << "Testing push_back() on an empty sequence" << endl; cout << "-------------------" << endl; Sequence data(0); data.push_back(100); data.push_back(200); data.push_back(300); data.push_back(400); data.push_back(500); cout << "Sequence: " << data << endl; cout << "Should be: <100, 200, 300, 400, 500>" << endl << endl; }
catch (exception& e) { cout << "Exception: " << e.what() << endl << endl; }
// Test pop_back try { cout << "Testing pop_back()" << endl; cout << "------------------" << endl; Sequence data(5); for (int i = 0; i < 5; i++) { data[i] = (i + 1) * 100; } data.pop_back(); data.pop_back(); cout << "Sequence: " << data << endl; cout << "Should be: <100, 200, 300>" << endl << endl;
}
catch (exception& e) { cout << "Exception: " << e.what() << endl << endl; }
// Test pop_back on empty sequence try { cout << "Testing pop_back() on an empty sequence" << endl; cout << "------------------" << endl; Sequence data(3); for (int i = 0; i < 3; i++) { data[i] = (i + 1) * 100; } data.pop_back(); data.pop_back(); data.pop_back(); data.pop_back(); cout << "ERROR: Pop_back() DID NOT throw an exception" << endl << endl;
}
catch (exception& e) { cout << "CORRECT: Threw exception: " << e.what() << endl << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
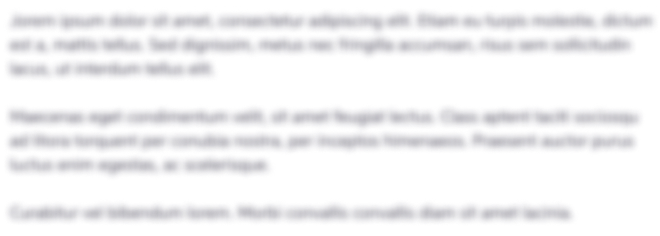
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started