Question
i need help on how to fix the error in this java program and for each error please tell me how to fix it package
i need help on how to fix the error in this java program and for each error please tell me how to fix it
package cs185debuggingq;
public class CS185Debugginq {
public static void main(String[] args) { //Explain to the user what the program does System.out.println("This program creates any number of House Objects");
Residents Resident1 = new Residents ("Rousseau", "Joseph", 18, 1, true, 1, 1); Residents Resident2 = new Residents ("Bemis", "Shari", 19, 2, true, 1, 1); Residents Resident3 = new Residents ("Mouse", "Mickey", 90, 1, true, 1, 1);
//Instantiate several house object House house1 = new House(4, 3.5, 3000.00, 1.5, "green", "101 Evergreen Circle", '1', Resident1); House house2 = new House(3, 2.5, 2500.00, 1.25, "white", "103 Evergreen Circle", '2', Resident2); House house3 = new House(2, 1.0, 2000.00, 1.0, "brown", "105 Evergreen Circle", '3', Resident3);
//Print information for each house System.out.println("House 1: " +house1); System.out.println("House 2: " +house2); System.out.println("House 3: " +house3);
//Change the color of house1 house1.setColor("blue");
//Change the number of baths for house2 house2.setNumBaths(2.0);
//Change the number of acreage for house3 house3.setAcreage(2.0);
//Print just the number of baths for house2 System.out.println("House2 now has " +house2.getNumBaths()+ " bathrooms.");
//Print just the color of house1 System.out.println("House1 is now the color " +house1.getColor( )+ ".");
//Reprint information for each house System.out.println("House 1: " +house); System.out.println("House 2: " +house); System.out.println("House 3: " +house); } // END OF DRIVER METHOD, main() } // END OF DRIVER CLASS. package cs185debuggingq;
public class House
{ //Instance Data private int intNumBedrooms; private double dblNumBaths; private double dblSquareft; private double dblAcreage; private String strColor; private String strAddress; private int intLotNum; private Residents Resident;
//Contructor Method
public House(int bedrooms, double bauths, double squareft, double acreage, String color, String address, int lotNum, Residents Resident) { this.intNumBedrooms = intNumBedrooms; this.dblNumBaths = dblNumBaths; this.dblSquareft = dblSquareft; this.dblAcreage = dblAcreage; this.strColor = color; this.strAddress = address; this.intLotNum = lotNum; this.Resident = Resident;
}
//Getters and Setters
public int getNumBedrooms() { return intNumBedrooms; } public void setNumBedrooms(int numBedrooms) { this.intNumBedrooms = numBedrooms; }
public double getNumBaths() { return dblNumBaths; }
public void setNumBaths(double numBaths) { this.dblNumBaths = numBaths; }
public double getSquareft() { return dblSquareft; }
public void setSquareft(double squareft) { this.dblSquareft = squareft; }
public double getAcreage() { return dblAcreage; }
public void setAcreage(double acreage) { this.dblAcreage = acreage; }
public String getColor() { return strColor; }
public void setColor(String color) { this.strColor = color; }
public String getAddress() { return strAddress; }
public void setAddress(String address) { this.strAddress = address; }
public int getLotNum() { return intLotNum; }
public void setLotNum(int lotNum) { this.intLotNum = lotNum; }
//toString
@Override public String toString() { return "House{" + "numBedrooms=" + numBedrooms + ", numBaths=" + numBaths + ", squareft=" + squareft + ", acreage=" + acreage + ", color=" + color + ", address=" + address + ", lotNum=" + lotNum + " " +Resident+ '}'; }
}
} package cs185debuggingq;
public class Residents
{ //Instance Data
private String lastName; private String firstName; private int age; private int numberofPets; private boolean owner; private int numberofOccupants;
//Constructor Method
public Residents(String lastName, String firstName, int age, int numberofPets, boolean owner, int numberofOccupants) { this.lastName = lastName; this.firstName = firstName; this.age = age; this.numberofPets = numberofPets; this.owner = owner; this.numberofOccupants = numberofOccupants; }
//Getters and Setters
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public int getNumberofPets() { return numberofPets; }
public void setNumberofPets(int numberofPets) { this.numberofPets = numberofPets; }
public boolean isOwner() { return owner; }
public void setOwner(boolean owner) { this.owner = owner; }
public int getNumberofOccupants() { return numberofOccupants; }
public void setNumberofOccupants(int numberofOccupants) { this.numberofOccupants = numberofOccupants; }
//toString
@Override public String toString() { return "Residents{" + "lastName=" + lastName + ", firstName=" + firstName + ", age=" + age + ", numberofPets=" + numberofPets + ", owner=" + owner ", numberofOccupants=" + numberofOccupants + '}'; }
} // END OF CLASS, Residents.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
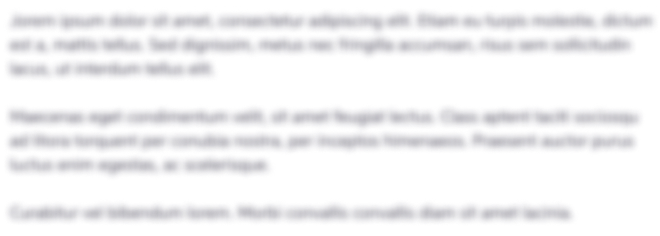
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started