Question
I need help on implementing linked list on my program. My program works but I need to implement linked list and then quicksort or merge
I need help on implementing linked list on my program. My program works but I need to implement linked list and then quicksort or merge sort. But the most important think is the linked list. Please help me complete the program
//diet.h
class Diet
{
public:
Diet();
~Diet();
void BMI(double weight, double height);
void recDiet(double BMI);
void getDATA();
void DietList();
void Menu();
void Exit();
void ArkinsDiet();
void TheZoneDiet();
void KetoDiet();
void VegeterianDiet();
void VeganDiet();
void WeightWatchersdiet();
void SouthBeachDiet();
void RawFoodDiet();
void MediterraneanDiet();
void spacer();
};
//diet.cpp
#include "stdafx.h"
#include "Diet.h"
#include "stdafx.h"
#include
#include
using namespace std;
Diet::Diet()
{
}
Diet::~Diet()
{
}
void Diet::BMI(double weight, double height)
{
double BMI;
BMI = (weight / (height * height)); // Calculates the bmi
cout << BMI << endl;
recDiet(BMI);
}
void Diet::recDiet(double BMI)
{
char a;
if (BMI < 18.5) {
cout << "You are Underweight. ";
cout << "Your recomended Diet is Mediteranian" << endl;
cout << "You nedd to eat Healthy Foods!" << endl;
cout << "Do you want to see what diet is recomended for you? Y/N : ";
cin >> a;
if (a == 'y' || a == 'Y')
{
spacer();
MediterraneanDiet();
spacer();
}else;
}
else if (BMI >= 18.5 && BMI <= 24.9) {
cout << "The diet you need is Vegan Diet"< cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); VeganDiet(); spacer(); } else; } else if (BMI >= 25 && BMI <= 29.9) { cout << "You are Overweight. " << endl; cout << "You need to have a DIET!"< cout << "Your Recomended Diet is Vegan" << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); VeganDiet(); spacer(); } else; } else if (BMI >= 30) { cout << "You are obese. " << endl; cout << "You seriously to have a DIET!" << endl; cout << "Your Recomended Diet is Weight Watcher Diet" << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); WeightWatchersdiet(); spacer(); } else; } else if (BMI <= 24.9) { cout << "The diet you need is Raw food diet" << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); RawFoodDiet(); spacer(); } else; } else if (BMI = 18.5) { cout << "The diet you need is Ketogenic Diet" << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); KetoDiet(); spacer(); } else; } else if (BMI <= 18.5) { cout << "The diet you need is Mediterranean diet" << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); MediterraneanDiet(); spacer(); } else; } else if (BMI >= 18.5 && BMI <= 24.9) { cout << "The diet you need is South beach diet, or The Zone Diet"; int choice1; char choice2; cout << "what diet do you prefer?" << endl; cout << " 1. South Beach Diet" << endl; cout << " 2. The Zone Diet" << endl; if (choice1 == 1) { SouthBeachDiet(); cout << "Do you want to view the other Diet Program? Y/N : "; cin >> choice2; if (choice2 == 'Y' || choice2 == 'y') { spacer(); TheZoneDiet(); spacer(); } else; } else if (choice2 == 2) { TheZoneDiet(); cout << "Do you want to view the other Diet Program? Y/N : "; cin >> choice2; if (choice2 == 'Y' || choice2 == 'y') { spacer(); SouthBeachDiet(); spacer(); } else; } else; } else if (BMI >= 25 && BMI <= 29.9) { cout << "The diet you need is Vegan Diet. " << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); VeganDiet(); spacer(); } else; } else if (BMI >= 30) { cout << "The diet you need is Weight watcher diet " << endl; cout << "Do you want to see what diet is recomended for you? Y/N : "; cin >> a; if (a == 'y' || a == 'Y') { spacer(); WeightWatchersdiet(); spacer(); } else; } Menu(); } void Diet::getDATA() { double weight; double height; double bmi; char choice; cout << "Enter weight in kilograms: "; cin >> weight; cout << "Enter height in meter: "; cin >> height; cout << "Your BMI is "; BMI(weight, height); cout << "Do you want to see other Diet Plans? (Y/N)"; cin >> choice; if (choice == 'y' || choice == 'Y') DietList(); else; } void Diet::DietList() { int ch; cout << "Listed Bellow are the most popular diets of this year"; cout << endl; cout << endl; cout << " 1. Atkins diet"< cout << " 2. The Zone diet" << endl; cout << " 3. Ketogenic diet" << endl; cout << " 4. Vegetarian diet" << endl; cout << " 5. Vegan diet" << endl; cout << " 6. Weight Watchers diet" << endl; cout << " 7. South Beach diet" << endl; cout << " 8. Raw food diet" << endl; cout << " 9. Mediterranean diet" << endl; cout << endl; cout << "Please Enter Number Of Choice : "; cin >> ch; if (ch == 1) { ArkinsDiet(); } else if (ch == 2) { TheZoneDiet(); } else if (ch == 3) { KetoDiet(); } else if (ch == 4) { VegeterianDiet(); } else if (ch == 5) { VeganDiet(); } else if (ch == 6) { WeightWatchersdiet(); } else if (ch == 7) { SouthBeachDiet(); } else if (ch == 8) { RawFoodDiet(); } else if (ch == 9) { MediterraneanDiet(); } else { cout << "You have entered a wrong Choice!!!!"; } Menu(); } void Diet::Menu() { int choice; cout << "WELCOME!!!"< cout << "This Program was made by GRUPO NG PROGRAMERS of COE116 Lab section B1" << endl; cout << " 1. Compute BMI" << endl; cout << " 2. Show List of DIets "< cout << " 3. Exit" << endl; cout << "Please Enter a choice : "; cin >> choice; if (choice == 1) getDATA(); else if (choice == 2) DietList(); else if (choice == 3) Exit(); else cout << "Wrong Choice Enter Valid choice!!!!"; } void Diet::Exit() { cout << "Thank You For Using Our Program" << endl; cout << "Grupo ng programmers"< cout << endl; cout << " Guevarra, Simon" << endl; cout << " Ablaza, Dexter" << endl; cout << " Balmaceda, William Daniel" << endl; cout << " Gonzales, Jomar" << endl; } void Diet::ArkinsDiet() { cout << "The Atkins Diet Is a 4-Phase Plan"< cout << "1. Phase 1 (induction): Under 20 grams of carbs per day for 2 weeks. " " Eat high-fat, high-protein, with low-carb vegetables like leafy greens. " " This kick-starts the weight loss."<< endl; cout << "" << endl; cout << "2. Phase 2 (balancing): Slowly add more nuts, low-carb vegetables and small amounts of fruit back to your diet." << endl; cout << "" << endl; cout << "3. Phase 3 (fine-tuning): When you are very close to your goal weight, add more carbs to your diet until weight loss slows down." << endl; cout << "" << endl; cout << "4. Phase 4 (maintenance): Here you can eat as many healthy carbs as your body can tolerate without regaining weight." << endl; cout << "" << endl; cout << "" << endl; cout << "Foods to Avoid" << endl; cout << "" << endl; cout << " Sugar: Soft drinks, fruit juices, cakes, candy, ice cream, etc." << endl; cout << " Grains: Wheat, spelt, rye, barley, rice." << endl; cout << " Vegetable oils: Soybean oil, corn oil, cottonseed oil, canola oil and a few others." << endl; cout << " Trans fats: Usually found in processed foods with the word hydrogenated on the ingredients list." << endl; cout << " Diet and low - fat foods: These are usually very high in sugar." << endl; cout << " High-carb vegetables: Carrots, turnips, etc (induction only)." << endl; cout << " High-carb fruits: Bananas, apples, oranges, pears, grapes (induction only)." << endl; cout << " Starches: Potatoes, sweet potatoes (induction only)." << endl; cout << " Legumes: Lentils, beans, chickpeas, etc (induction only)." << endl; cout << "" << endl; cout << "" << endl; cout << "Foods to Eat" << endl; cout << " Meats: Beef, pork, lamb, chicken, bacon and others." << endl; cout << " Fatty fish and seafood: Salmon, trout, sardines, etc. Fatty fish and seafood: Salmon, trout, sardines, etc." << endl; cout << " Eggs: The healthiest eggs are Omega-3 enriched or pastured." << endl; cout << " Low-carb vegetables: Kale, spinach, broccoli, asparagus and others." << endl; cout << " Full-fat dairy: Butter, cheese, cream, full-fat yoghurt." << endl; cout << " Nuts and seeds: Almonds, macadamia nuts, walnuts, sunflower seeds, etc." << endl; cout << " Healthy fats: Extra virgin olive oil, coconut oil, avocados and avocado oil." << endl; cout << "" << endl; cout << "" << endl; } void Diet::TheZoneDiet() { cout << "The Zone Diet: A Complete Overview" << endl; cout << "" << endl; cout << "The Zone Diet has been popular for several decades." << endl; cout << "It encourages followers to eat a certain amount of protein, carbs and fat at every meal in order to reduce inflammation in the body, among other health benefits." << endl; cout << "However, critics have targeted some of its health claims." << endl; cout << "" << endl; cout << "What Is the Zone Diet?" << endl; cout << "The Zone Diet instructs its followers to stick to eating a specific ratio of 40% carbs, 30% protein and 30% fat." << endl; cout << "As part of the diet, carbs should have a low glycemic index, which means they provide a slow release of sugar into the blood to keep you fuller for longer. " << endl; cout << "Protein should be lean and fat should be mostly monounsaturated." << endl; cout << "The Zone Diet was developed more than 30 years ago by Dr. Barry Sears, an American biochemist. His best-selling book The Zone was published in 1995." << endl; cout << "Dr. Sears developed this diet after losing family members to early deaths from heart attacks, and felt that he was at risk unless he found a way to fight it." << endl; cout << "The Zone Diet claims to reduce the inflammation in your body. Dr. Sears proposed inflammation was the reason people gain weight, become sick and age faster." << endl; cout << "Proponents of the diet claim that once you reduce inflammation, you will lose fat at the fastest rate possible, slow down aging, reduce your risk of chronic disease and improve your performance." << endl; cout << "" << endl; cout << "How Do You Follow the Zone Diet?" << endl; cout << "The Zone Diet has no specific phases and is designed to be followed for a lifetime." << endl; cout << "There are two ways to follow the Zone Diet: the hand-eye method, or using Zone food blocks." << endl; cout << "Most people start with the hand-eye method and progress to using Zone food blocks later, since it is more advanced. You can switch between both methods whenever you feel like, since they each have their own benefits." << endl; cout << "" << endl; cout << "The Hand-Eye Method" << endl; cout << "" << endl; cout << "The hand-eye method is the easiest way to start the Zone Diet." << endl; cout << "As the name suggests, your hand and eye are the only tools you need to get started, although wearing a watch is also recommended to keep an eye on when to eat." << endl; cout << "In this method, your hand takes on several uses. You use it to determine your portion sizes." << endl; cout << "Your five fingers remind you to eat five times a day and never go without food for five hours." << endl; cout << "Meanwhile, you use your eye to estimate portions on your plate. To design a Zone-friendly plate, you need to first divide your plate into thirds." << endl; cout << " One-third lean protein: One-third of your plate should have a source of lean protein, roughly the size and thickness of your palm." << endl; cout << " Two-thirds carbs: Two-thirds of your plate should be filled with carbs with a low glycemic index." << endl; cout << " A little fat: Add a dash of monounsaturated fat to your plate, such as olive oil, avocado or almonds." << endl; cout << "The hand-eye method is designed to be a simple way for a beginner to follow the Zone Diet." << endl; cout << "It is also flexible and allows you to eat out at restaurants while on the Zone Diet, by using your hand and eyes as tools to choose options that fit Zone recommendations." << endl; cout << "" << endl; cout << "What Foods Can You Eat on the Zone Diet?" << endl; cout << "Protein" << endl; cout << "Protein options in the Zone Diet should be lean. Good options include:" << endl; cout << " Lean beef, pork, lamb, veal and game" << endl; cout << " Skinless chicken and turkey breast" << endl; cout << " Fish and shellfish" << endl; cout << " Vegetarian protein, tofu, other soy products" << endl; cout << " Egg whites" << endl; cout << " Low-fat cheeses" << endl; cout << " Low-fat milk and yogurt" << endl; cout << "" << endl; cout << "Fat" << endl; cout << "The Zone Diet encourages choosing a type of monounsaturated fat. Good options include:" << endl; cout << " Avocados" << endl; cout << " Nuts, such as macadamia, peanuts, cashews, almonds or pistachios" << endl; cout << " Peanut butter" << endl; cout << " Tahini" << endl; cout << " Oils such as canola oil, sesame oil, peanut oil and olive oil" << endl; cout << "" << endl; cout << "Carbs" << endl; cout << "The Zone Diet encourages its followers to choose vegetables with a low glycemic index and a little fruit." << endl; cout << " Fruit such as berries, apples, oranges, plums and more" << endl; cout << " Vegetables such as cucumbers, peppers, spinach, tomatoes, mushrooms, yellow squash, chickpeas and more" << endl; cout << " Grains, such as oatmeal and barley" << endl; cout << "" << endl; cout << "What Can't You Eat on the Zone Diet?" << endl; cout << "Nothing is strictly banned on the Zone Diet. However, certain food choices are considered unfavorable because they promote inflammation." << endl; cout << " High-sugar fruits: Such as bananas, grapes, raisins, dried fruits and mangoes." << endl; cout << " High-sugar or starchy vegetables: Like peas, corn, carrots and potatoes." << endl; cout << " Refined and processed carbs: Bread, bagels, pasta, noodles and other white-flour products." << endl; cout << " Other processed foods: Including breakfast cereals and muffins." << endl; cout << " Foods with added sugar: Such as candy, cakes and cookies." << endl; cout << " Foods with added sugar: Such as candy, cakes and cookies." << endl; cout << " Coffee and tea: Keep these to a minimum, since water is the beverage of choice." << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; cout << "" << endl; } void Diet::KetoDiet() { cout << "KETOGENIC DIET BASICS" << endl; cout << "Basically, the purpose of the ketogenic diet is to force the body into burning fats instead of carbohydrates. " << endl; cout << "Those who follow it eat a diet that contains high amounts of fat, moderate amounts of protein, and low levels of carbohydrates." << endl; cout << "Through this breakdown of macronutrients, youre able to change how the body uses energy to produce some pretty awesome benefits. " << endl; cout << "But to fully understand how it works, its important to have a grasp on exactly how the body uses energy in the first place." << endl; cout << "" << endl; cout << "LOW CARB DIETS VS KETOGENIC DIETS" << endl; cout << "The keto diet often gets lumped in with any type of low-carb diet, but there are differences that should be noted. " << endl; cout << "Some low-carb diets are not ketogenic, and the biggest difference is the level of carbohydrate intake." << endl; cout << "For example, a low-carb diet might involve a moderate decrease in carbohydrates but not a large enough decrease to send you into ketosis. " << endl; cout << "For example, the more modern version of Atkins often involves people adding in more carbs over time, which may be too much to go into ketosis or stay in ketosis for long periods of time." << endl; cout << "" << endl; cout << "RECOMMENDED FOODS FOR KETOGENIC DIET" << endl; cout << " Meats including: beef, chicken and other poultry, pork, lamb, goat, turkey, veal, and fish sources like salmon, sardines, catfish, tuna, trout, etc." << endl; cout << " Fats and oils including: nuts and seeds (whole or as butters), oils like olive oil, sesame oil, or high oleic sunflower and safflower oils, ghee, and grass-fed butter." << endl; cout << " Eggs(preferably free-range)." << endl; cout << " Dairy products including: cheeses, sour cream, yogurt, and heavy creams." << endl; cout << " Low-carb vegetables including: spinach, kale, broccoli, Brussels sprouts, asparagus, peppers, and onions." << endl; cout << " ONLY lower-sugar fruits including: blueberries, strawberries, raspberries, and avocados (and only in small amounts)." << endl; cout << " Herbs and spices: as long as they have no added sugars." << endl; cout << "" << endl; cout << "FOOD TO ELIMINATE ON KETOGENIC DIET" << endl; cout << " Beans and legumes including: kidney beans, chickpeas, black beans, lentils, and green peas." << endl; cout << " Grains including: whole grains and breads and pastas made from grains like oats, wheat, barley, rice, rye, and corn." << endl; cout << " Fruits besides small portions of berries." << endl; cout << " Starches including: potatoes, sweet potatoes, parsnips, and carrots." << endl; cout << " Low-fat diet products that are packaged and processed." << endl; cout << " Sugar-laden products including: smoothies, sodas, fruit juices, ice cream, cookies, cakes, and candies." << endl; cout << " Unhealthy oils including: mayonnaise products and processed vegetable oils" << endl; cout << " Alcohols, as they can take you out of ketosis from the high carb content." << endl; cout << " Artificial sweeteners, which can sometimes affect blood sugar levels." << endl; cout << " Condiments that contain added sugar or unhealthy oils." << endl; cout << "" << endl; cout << "HEALTH BENEFITS ON A KETOGENIC DIET" << endl; cout << "Since a low-carb diet has been shown to have greater effects on weight loss than other diets, " << endl; cout << "its a good option for obese people who are looking to reduce their weight, blood pressure, and cholesterol " << endl; cout << "over a short period of time. Plus, a ketogenic diet may also help improve insulin resistance and lower glucose levels." << endl; cout << "" << endl; } void Diet::VegeterianDiet() { cout << "What Is the Vegan Diet?" << endl; cout << "Veganism is defined as a way of living that attempts to exclude all forms of animal exploitation and cruelty, whether for food, clothing or any other purpose." << endl; cout << "For these reasons, the vegan diet is devoid of all animal products, including meat, eggs and dairy." << endl; cout << "People choose to follow a vegan diet for various reasons." << endl; cout << "These usually range from ethics to environmental concerns, but they can also stem from a desire to improve health." << endl; cout << "BOTTOM LINE:A vegan diet excludes all animal products. Many people choose to eat this way for ethical, environmental or health reasons." << endl; cout << "" << endl; cout << "Different Types of Vegan Diets" << endl; cout << " Whole-food vegan diet: A diet based on a wide variety of whole plant foods such as fruits, vegetables, whole grains, legumes, nuts and seeds." << endl; cout << " Raw-food vegan diet: A vegan diet based on raw fruits, vegetables, nuts, seeds or plant foods cooked at temperatures below 118F (48C) (1)." << endl; cout << " 80/10/10: The 80/10/10 diet is a raw-food vegan diet that limits fat-rich plants such as nuts and avocados and relies mainly on raw fruits and soft greens instead. Also referred to as the low-fat, raw-food vegan diet or fruitarian diet." << endl; cout << " The starch solution: A low-fat, high-carb vegan diet similar to the 80/10/10 but that focuses on cooked starches like potatoes, rice and corn instead of fruit." << endl; cout << " Raw till 4: A low-fat vegan diet inspired by the 80/10/10 and starch solution. Raw foods are consumed until 4 p.m., with the option of a cooked plant-based meal for dinner." << endl; cout << " The thrive diet: The thrive diet is a raw-food vegan diet. Followers eat plant-based, whole foods that are raw or minimally cooked at low temperatures." << endl; cout << " Junk-food vegan diet: A vegan diet lacking in whole plant foods that relies heavily on mock meats and cheeses, fries, vegan desserts and other heavily processed vegan foods." << endl; cout << "Although several variations of the vegan diet exist, most scientific research rarely differentiates between different types of vegan diets." << endl; cout << "Therefore, the information provided in this article relates to vegan diets as a whole." << endl; cout << "" << endl; cout << "Other Health Benefits of Vegan Diets" << endl; cout << " Cancer risk: Vegans may benefit from a 15% lower risk of developing or dying from cancer " << endl; cout << " Arthritis: Vegan diets seem particularly effective at reducing symptoms of arthritis such as pain, joint swelling and morning stiffness " << endl; cout << " Kidney function: Diabetics who substitute meat for plant protein may reduce their risk of poor kidney function" << endl; cout << " Alzheimer's disease: Observational studies show that aspects of the vegan diet may help reduce the risk of developing Alzheimer's disease" << endl; cout << "That said, keep in mind that most of the studies supporting these benefits are observational." << endl; cout << "This makes it difficult to determine whether the vegan diet directly caused the benefits." << endl; cout << "" << endl; cout << "Foods to Avoid" << endl; cout << " Meat and poultry: Beef, lamb, pork, veal, horse, organ meat, wild meat, chicken, turkey, goose, duck, quail, etc." << endl; cout << " Fish and seafood: All types of fish, anchovies, shrimp, squid, scallops, calamari, mussels, crab, lobster, etc." << endl; cout << " Dairy: Milk, yogurt, cheese, butter, cream, ice cream, etc." << endl; cout << " Eggs: From chickens, quails, ostriches, fish, etc." << endl; cout << " Bee products: Honey, bee pollen, royal jelly, etc." << endl; cout << " Animal-based ingredients: Whey, casein, lactose, egg white albumen, gelatin, cochineal or carmine, isinglass, shellac, L-cysteine, animal-derived vitamin D3 and fish-derived omega-3 fatty acids." << endl; cout << "" << endl; cout << "Foods to Eat" << endl; cout << " Tofu, tempeh and seitan: These provide a versatile protein-rich alternative to meat, fish, poultry and eggs in many recipes." << endl; cout << " Legumes: Foods such as beans, lentils and peas are excellent sources of many nutrients and beneficial plant compounds. Sprouting, fermenting and proper cooking can increase nutrient absorption " << endl; cout << " Nuts and nut butters: Especially unblanched and unroasted varieties, which are good sources of iron, fiber, magnesium, zinc, selenium and vitamin E " << endl; cout << " Seeds: Especially hemp, chia and flaxseeds, which contain a good amount of protein and beneficial omega-3 fatty acids " << endl; cout << " Algae: Spirulina and chlorella are good sources of complete protein. Other varieties are great sources of iodine." << endl; cout << " Nutritional yeast: This is an easy way to increase the protein content of vegan dishes and add an interesting cheesy flavor. Pick vitamin B12-fortified varieties whenever possible." << endl; cout << " Whole grains, cereals and pseudocereals: These are a great source of complex carbs, fiber, iron, B-vitamins and several minerals. " << endl; cout << " Sprouted and fermented plant foods: Ezekiel bread, tempeh, miso, natto, sauerkraut, pickles, kimchi and kombucha often contain probiotics and vitamin K2. " << endl; cout << " Fruits and vegetables: Both are great foods to increase your nutrient intake." << endl; cout << "" << endl; } void Diet::VeganDiet() { cout << "What Is the Vegan Diet?" << endl; cout << "Veganism is defined as a way of living that attempts to exclude all forms of animal exploitation and cruelty, whether for food, clothing or any other purpose." << endl; cout << "For these reasons, the vegan diet is devoid of all animal products, including meat, eggs and dairy." << endl; cout << "People choose to follow a vegan diet for various reasons." << endl; cout << "These usually range from ethics to environmental concerns, but they can also stem from a desire to improve health." << endl; cout << "BOTTOM LINE:A vegan diet excludes all animal products. Many people choose to eat this way for ethical, environmental or health reasons." << endl; cout << "" << endl; cout << "Different Types of Vegan Diets" << endl; cout << " Whole-food vegan diet: A diet based on a wide variety of whole plant foods such as fruits, vegetables, whole grains, legumes, nuts and seeds." << endl; cout << " Raw-food vegan diet: A vegan diet based on raw fruits, vegetables, nuts, seeds or plant foods cooked at temperatures below 118F (48C) (1)." << endl; cout << " 80/10/10: The 80/10/10 diet is a raw-food vegan diet that limits fat-rich plants such as nuts and avocados and relies mainly on raw fruits and soft greens instead. Also referred to as the low-fat, raw-food vegan diet or fruitarian diet." << endl; cout << " The starch solution: A low-fat, high-carb vegan diet similar to the 80/10/10 but that focuses on cooked starches like potatoes, rice and corn instead of fruit." << endl; cout << " Raw till 4: A low-fat vegan diet inspired by the 80/10/10 and starch solution. Raw foods are consumed until 4 p.m., with the option of a cooked plant-based meal for dinner." << endl; cout << " The thrive diet: The thrive diet is a raw-food vegan diet. Followers eat plant-based, whole foods that are raw or minimally cooked at low temperatures." << endl; cout << " Junk-food vegan diet: A vegan diet lacking in whole plant foods that relies heavily on mock meats and cheeses, fries, vegan desserts and other heavily processed vegan foods." << endl; cout << "Although several variations of the vegan diet exist, most scientific research rarely differentiates between different types of vegan diets." << endl; cout << "Therefore, the information provided in this article relates to vegan diets as a whole." << endl; cout << "" << endl; cout << "Other Health Benefits of Vegan Diets" << endl; cout << " Cancer risk: Vegans may benefit from a 15% lower risk of developing or dying from cancer " << endl; cout << " Arthritis: Vegan diets seem particularly effective at reducing symptoms of arthritis such as pain, joint swelling and morning stiffness " << endl; cout << " Kidney function: Diabetics who substitute meat for plant protein may reduce their risk of poor kidney function" << endl; cout << " Alzheimer's disease: Observational studies show that aspects of the vegan diet may help reduce the risk of developing Alzheimer's disease" << endl; cout << "That said, keep in mind that most of the studies supporting these benefits are observational." << endl; cout << "This makes it difficult to determine whether the vegan diet directly caused the benefits." << endl; cout << "" << endl; cout << "Foods to Avoid" << endl; cout << " Meat and poultry: Beef, lamb, pork, veal, horse, organ meat, wild meat, chicken, turkey, goose, duck, quail, etc." << endl; cout << " Fish and seafood: All types of fish, anchovies, shrimp, squid, scallops, calamari, mussels, crab, lobster, etc." << endl; cout << " Dairy: Milk, yogurt, cheese, butter, cream, ice cream, etc." << endl; cout << " Eggs: From chickens, quails, ostriches, fish, etc." << endl; cout << " Bee products: Honey, bee pollen, royal jelly, etc." << endl; cout << " Animal-based ingredients: Whey, casein, lactose, egg white albumen, gelatin, cochineal or carmine, isinglass, shellac, L-cysteine, animal-derived vitamin D3 and fish-derived omega-3 fatty acids." << endl; cout << "" << endl; cout << "Foods to Eat" << endl; cout << " Tofu, tempeh and seitan: These provide a versatile protein-rich alternative to meat, fish, poultry and eggs in many recipes." << endl; cout << " Legumes: Foods such as beans, lentils and peas are excellent sources of many nutrients and beneficial plant compounds. Sprouting, fermenting and proper cooking can increase nutrient absorption " << endl; cout << " Nuts and nut butters: Especially unblanched and unroasted varieties, which are good sources of iron, fiber, magnesium, zinc, selenium and vitamin E " << endl; cout << " Seeds: Especially hemp, chia and flaxseeds, which contain a good amount of protein and beneficial omega-3 fatty acids " << endl; cout << " Algae: Spirulina and chlorella are good sources of complete protein. Other varieties are great sources of iodine." << endl; cout << " Nutritional yeast: This is an easy way to increase the protein content of vegan dishes and add an interesting cheesy flavor. Pick vitamin B12-fortified varieties whenever possible." << endl; cout << " Whole grains, cereals and pseudocereals: These are a great source of complex carbs, fiber, iron, B-vitamins and several minerals. " << endl; cout << " Sprouted and fermented plant foods: Ezekiel bread, tempeh, miso, natto, sauerkraut, pickles, kimchi and kombucha often contain probiotics and vitamin K2. " << endl; cout << " Fruits and vegetables: Both are great foods to increase your nutrient intake." << endl; cout << "" << endl; } void Diet::WeightWatchersdiet() { cout << "Weight Watchers" << endl; cout << "The Promise" << endl; cout << "Pasta, steak, cheese, ice cream ... you can eat what you want on Weight Watchers." << endl; cout << "While the popular weight-loss plan has been revamped, the basic principle of eating what you love remains," << endl; cout << "though the program steers you toward healthier foods with its points system." << endl; cout << "" << endl; cout << "In its new program, called WW Freestyle, you can roll over some of your points to another day. " << endl; cout << "And there are also more than 200 zero-point foods that you dont need to track at all." << endl; cout << "Those items include beans, chicken breast (skinless), eggs, and fish." << endl; cout << "Weight Watchers isnt so much a diet as a lifestyle-change program. " << endl; cout << "It can help you learn how to eat healthier and get more physical activity, so you lose the weight for good." << endl; cout << "You can follow the plan online on your own. You'll track your food choices and exercise, chart progress, and find recipes and workouts." << endl; cout << "Theres a coaching option if you prefer one-on-one consultations by phone, email, and text. " << endl; cout << "Or you can go to in-person group meetings where youll weigh in." << endl; cout << "" << endl; cout << "A Consumer Reports survey found that people who went to " << endl; cout << "meetings were more satisfied with the program and lost " << endl; cout << "more weight than people who used only the online tools." << endl; cout << "" << endl; cout << "What You Can Eat and What You Can't" << endl; cout << "No food is forbidden when you follow this plan, which doesnt make you buy any prepackaged meals." << endl; cout << "Weight Watchers assigns different foods a SmartPoints value. Nutritious foods that fill you up have fewer points than junk with empty calories. " << endl; cout << "The eating plan factors sugar, fat, and protein into its points calculations to steer you toward fruits, veggies," << endl; cout << "Youll have a SmartPoints target that's set up based on your body and goals.and lean protein, and away from stuff that's high in sugar and saturated fat." << endl; cout << "As long as you stay within your daily target, you can spend those SmartPoints however youd like," << endl; cout << "even on alcohol or dessert, or save them to use another day." << endl; cout << "But healthier, lower-calorie foods cost fewer points. And some items now have 0 points." << endl; cout << "" << endl; } void Diet::SouthBeachDiet() { cout << "How Does the South Beach Diet Work Exactly?" << endl; cout << "An important emphasis of the South Beach Diet is controlling hunger by eating before it strikes. " << endl; cout << "To that end, the South Beach Diet includes three different phases." << endl; cout << "Phase 1 is one week long and aims to reset your body to help burn fat and increase your metabolism" << endl; cout << "as well as reduce sugar and starch cravings." << endl; cout << "Phase 2 is for steady weight loss, where you add in good carbs to your diet. " << endl; cout << "Phase 3 is the weight-maintenance phase, where you learn to maintain your new weight without deprivation or hunger, according to the South Beach Diet website." << endl; cout << "The phases help jump-start some weight loss, Stephens explains. Its a mental thing: When patients see early success, " << endl; cout << "theyre more likely to stick to the plan." << endl; cout << "She notes that the different phases also help acclimate people to a new lifestyle, since phase three is essentially a lifelong choice rather than a diet." << endl; cout << "" << endl; cout << "A Sample Menu of Phase 1 of the South Beach Diet" << endl; cout << "Breakfast South Beach Complete shake" << endl; cout << "Snack Plain Greek yogurt with fresh dill and lemon juice, along with celery sticks and cherry tomatoes" << endl; cout << "Lunch Grilled chicken, an avocado, and cooked broccoli" << endl; cout << "Snack South Beach Diet Peanut Chocolate Bar" << endl; cout << "Dinner Baked salmon, white beans, sauted cabbage and garlic" << endl; cout << "Evening Snack Almonds" << endl; cout << "" << endl; cout << "A Sample Menu of Phase 2 of the South Beach Diet" << endl; cout << "Breakfast Breakfast pita with spinach, eggs, and feta cheese, vegetable juice, and tea or coffee" << endl; cout << "Snack Assorted vegetables with a cilantro and pesto dip" << endl; cout << "Lunch Curried turkey and greens salad" << endl; cout << "Snack Apple and peanut butter sandwiches" << endl; cout << "Dinner Edamame appetizer, Louisiana-style shrimp and rice, baked tomatoes topped with Parmesan cheese" << endl; cout << "Dessert: South Beach Dietstyle tiramisu" << endl; cout << "" << endl; cout << "A Sample Menu of Phase 3 of the South Beach Diet" << endl; cout << "Breakfast Mini crustless quiches (eggs with peppers, onions, and cheese baked in a muffin tin) with a slice of whole-grain toast like Ezekiel bread, a cup of berries, and a cup of black coffee or tea" << endl; cout << "Lunch Salad greens with salmon or chicken and olive oil and vinegar dressing" << endl; cout << "Snack Greek yogurt with berries" << endl; cout << "Dinner A plate filled with half-roasted vegetables (zucchini, squash, red onion), a small, 4-ounce portion of lean meat (such as salmon, chicken, or beef tenderloin), and 1/3 cup of a hearty grain (faro, quinoa, barley)" << endl; cout << "Dessert Mascarpone cheese or Greek yogurt with peaches, topped with slivered almonds and cocoa powder" << endl; cout << "" << endl; } void Diet::RawFoodDiet() { cout << "The Raw Food Diet: A Beginner's Guide and Review" << endl; cout << "The raw food diet has been around since the 1800s, but has surged in popularity in recent years." << endl; cout << "Its supporters believe that consuming mostly raw foods is ideal for human health and has many benefits, including weight loss and better overall health." << endl; cout << "However, health experts warn that eating a mostly raw diet may lead to negative health consequences." << endl; cout << "This article reviews the good and bad of the raw food diet, as well as how it works." << endl; cout << "" << endl; cout << "What Is the Raw Food Diet?" << endl; cout << "The raw food diet, often called raw foodism or raw veganism, is composed of mostly or completely raw and unprocessed foods." << endl; cout << "A food is considered raw if it has never been heated over 104118F (4048C)." << endl; cout << "It should also not be refined, pasteurized, treated with pesticides or otherwise processed in any way." << endl; cout << "Instead, the diet allows several alternative preparation methods, such as juicing, blending, dehydrating, soaking and sprouting." << endl; cout << "Similar to veganism, the raw food diet is usually plant-based, being made up mostly of fruits, vegetables, nuts and seeds." << endl; cout << "While most raw food diets are completely plant-based, some people also consume raw eggs and dairy." << endl; cout << "Less commonly, raw fish and meat may be included as well." << endl; cout << "" << endl; cout << "Additionally, taking supplements is typically discouraged on the raw food diet. " << endl; cout << "Proponents often claim that the diet will give you all the nutrients you need." << endl; cout << "" << endl; cout << "Supporters also believe that cooking foods is harmful to human health because it destroys the natural enzymes in foods, " << endl; cout << "reduces their nutrient content and reduces the life force That they believe to exist in all raw or living foods." << endl; cout << "" << endl; cout << "People follow the raw food diet for the benefits they believe it has, including weight loss, improved vitality," << endl; cout << "increased energy, improvement to chronic diseases, improved overall health and a reduced impact on the environment." << endl; cout << "" << endl; cout << "" << endl; cout << "Foods to Eat" << endl; cout << " All fresh fruits"<< endl; cout << " All raw vegetables" << endl; cout << " Raw nuts and seeds" << endl; cout << " Raw grains and legumes, sprouted or soaked" << endl; cout << " Dried fruits and meats" << endl; cout << " Nut milks" << endl; cout << " Raw nut butters" << endl; cout << " Cold-pressed olive and coconut oils" << endl; cout << " Fermented foods like kimchi and sauerkraut" << endl; cout << " Seaweed" << endl; cout << " Sprouts" << endl; cout << " Raw eggs or dairy, if desired" << endl; cout << " Raw meat or fish, if desired" << endl; cout << "" << endl; cout << "" << endl; cout << "Foods to Avoid" << endl; cout << " Cooked fruits, vegetables, meats and grains" << endl; cout << " Baked items" << endl; cout << " Roasted nuts and seeds" << endl; cout << " Refined oils" << endl; cout << " Table salt" << endl; cout << " Refined sugars and flour" << endl; cout << " Pasteurized juices and dairy" << endl; cout << " Coffee and tea" << endl; cout << " Pasta" << endl; cout << " Alcohol" << endl; cout << " Pastries" << endl; cout << " Chips" << endl; cout << "" << endl; } void Diet::MediterraneanDiet() { cout << "A Mediterranean Diet Meal Plan" << endl; cout << "There is no one right way to do this diet. There are many countries around the Mediterranean sea and they didn't all eat the same things." << endl; cout << "This article describes the diet that was typically prescribed in the studies that showed it to be an effective way of eating." << endl; cout << "" << endl; cout << "The Basics" << endl; cout << " Eat: Vegetables, fruits, nuts, seeds, legumes, potatoes, whole grains, breads, herbs, spices, fish, seafood and extra virgin olive oil." << endl; cout << " Eat in moderation: Poultry, eggs, cheese and yogurt." << endl; cout << " Eat only rarely: Red meat." << endl; cout << " Don't eat: Sugar-sweetened beverages, added sugars, processed meat, refined grains, refined oils and other highly processed foods." << endl; cout << "" << endl; cout << "Avoid These Unhealthy Foods" << endl; cout << " Added sugar: Soda, candies, ice cream, table sugar and many others." << endl; cout << " Refined grains: White bread, pasta made with refined wheat, etc." << endl; cout << " Trans fats: Found in margarine and various processed foods." << endl; cout << " Refined Oils: Soybean oil, canola oil, cottonseed oil and others." << endl; cout << " Processed meat: Processed sausages, hot dogs, etc." << endl; cout << " Highly processed foods: Everything labelled low - fat or diet or looks like it was made in a factory." << endl; cout << "" << endl; cout << "Foods to Eat" << endl; cout << " Vegetables: Tomatoes, broccoli, kale, spinach, onions, cauliflower, carrots, Brussels sprouts, cucumbers, etc." << endl; cout << " Fruits: Apples, bananas, oranges, pears, strawberries, grapes, dates, figs, melons, peaches, etc." << endl; cout << " Nuts and Seeds: Almonds, walnuts, Macadamia nuts, hazelnuts, cashews, sunflower seeds, pumpkin seeds and more." << endl; cout << " Legumes: Beans, peas, lentils, pulses, peanuts, chickpeas, etc." << endl; cout << " Tubers: Potatoes, sweet potatoes, turnips, yams, etc." << endl; cout << " Whole Grains: Whole oats, brown rice, rye, barley, corn, buckwheat, whole wheat, whole grain bread and pasta." << endl; cout << " Fish and Seafood: Salmon, sardines, trout, tuna, mackerel, shrimp, oysters, clams, crab, mussels, etc." << endl; cout << " Poultry: Chicken, duck, turkey and more." << endl; cout << " Eggs: Chicken, quail and duck eggs." << endl; cout << " Dairy: Cheese, yogurt, Greek yogurt, etc." << endl; cout << " Herbs and Spices: Garlic, basil, mint, rosemary, sage, nutmeg, cinnamon, pepper, etc." << endl; cout << " Healthy Fats: Extra virgin olive oil, olives, avocados and avocado oil." << endl; cout << "" << endl; cout << "What to Drink" << endl; cout << "Water should be your go-to beverage on a Mediterranean diet." << endl; cout << "This diet also includes moderate amounts of red wine, around 1 glass per day." << endl; cout << "However, this is completely optional and wine should be avoided by anyone who has alcoholism or problems controlling their consumption." << endl; cout << "Coffee and tea are also completely acceptable, but avoid sugar-sweetened beverages and fruit juices, which are very high in sugar." << endl; cout << "" << endl; } void Diet::spacer() { cout << endl; cout << "-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-0-" << endl; cout << endl; } //int main #include "stdafx.h" #include "Diet.h" #include #include using namespace std; int main() { Diet a; a.Menu(); system("pause"); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
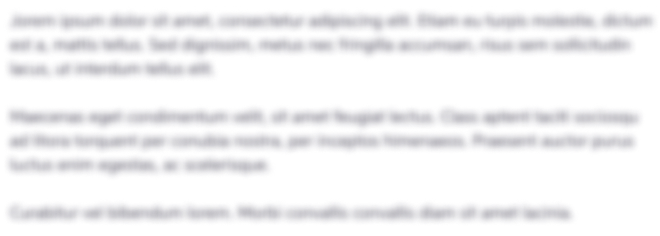
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started