Question
I need help on java aggregate Step1 make Room an aggregate class. Each Room has an AirConditioner . The Air Conditioning Units have the following
I need help on java aggregate Step1
make Room an aggregate class. Each Room has an AirConditioner.
The Air Conditioning Units have the following attributes:
Manufacturer
Type (can be Window Mounted or Portable)
Cooling Capacity (measured in BTUs per Hour)
Create a class named AirConditioner that will be used to model an Air Conditioning Unit. Use the following guidelines to create the AirConditioner class:
Include appropriate fields (Hint: See the list of important Air Conditioner attributes above)
Include a no-arg constructor and a constructor that accepts arguments (it must accept all necessary arguments to fully initialize the AirConditioner object).
Include appropriate setters and getters (i.e., mutator and accessor methods) for each field.
Modify your existing accessor and mutator methods. Use the "this" reference variable to overcome shadowing of a field name. Points will be taken off if you do not complete this step!
Include a toString method
Step 2
Modify the Room class by adding the following methods:
Method 1: hasAdequateCooling
The purpose of this method is to check the rooms air conditioner to see if it has a high enough cooling capacity to adequately cool the room.
For example, a Moderately Shaded room that is 500 square feet requires an air conditioner with a capacity of 10,000 BTUs per Hour. If that room has an air conditioner with a cooling capacity of 8,000 BTUs per Hour, then that room does not have adequate cooling.
The method should not accept any arguments, and should return true if the room has adequate cooling, and false if the room does not.
Method 2: toString
Use this method to display all of the relevant information of the Room class (including its AirConditioner)
Step 3
Minimize the risk of security holes in your program (Note: review the Security Issues with Aggregate Classes section in Chapter 8).
----------------------------------------------------------------
public class AirConditioner { // filed private String Manufacturer; private String type;// windo mounted or portable type private double coolingCapacity;// BTUs per hour // no-args constructer public AirConditioner() { Manufacturer=""; type=""; coolingCapacity=0.0; } // constructer that accept arguments constructer public AirConditioner(String Manufacturer,String type,double coolingCapacity) { this.Manufacturer=Manufacturer; this.type=type; this.coolingCapacity=coolingCapacity; } /** The copy constructer creates a cop of the air conditioner class that pass as a paramter. we use to avoid the scurity hole */ public AirConditioner( AirConditioner object2) { this.Manufacturer=object2.Manufacturer; this.type=object2.type; this.coolingCapacity=object2.coolingCapacity; } public void setManufacturer(String Manufacturer) { this.Manufacturer=Manufacturer; } public void setType(String type) { this.type=type; } public void setCoolingCapacity(double coolingCapacity) { this.coolingCapacity=coolingCapacity; } public String getManufacturer() { return Manufacturer; } public String getType() { return type; } public double getCoolingCapacity() { return coolingCapacity; } /** toString method @return a string containing manufacturer information. */ public String toString() { return "Air Conditioner Manufacturer: "+getManufacturer()+"Air Conditioner Type: " +getType() + "Air Conditioner Cooling Capacity: " +getCoolingCapacity()+ ""; } }
---------------------------------------------------------------------
public class Room { //Filed private String name; //name private double length; //length private double width; //width private String amountOfShade; //amount of shade private double cooling_capacity;// cooling capacity private final double LITTLE_COOLING_CAPACITY=0.15;// little room shade capacity increased by 15% private final double ABUNDANT_COOLING_CAPACITY=0.1;// Abundant room shade capacity decresed by 10% private AirConditioner airConditioner;// instance of the airConditioner class /** Constructor @param nam the name of the room @param len The length of the room. @param w The width of the room. @param cooling_capacity the capacity of the room @param airconditioner a AirCondioner object */ //no-arg constructor public Room() { name=""; length=0; width=0; amountOfShade=""; cooling_capacity=0; } public Room(String name,double length,double width,String amountOfShade,AirConditioner airconditioner) { this.name=name; this.length=length; this.width=width; this.amountOfShade=amountOfShade; airConditioner=new AirConditioner(airconditioner); } /** The setName method stores a value in the name field. @param name The value to store in name. */ public void setName(String name) { this.name=name; } /** The setLength method stores a value in the length field. @param length The value to store in length. */ public void setLength(double length) { this.length=length; } /** The setWidth method stores a value in the width field. @param width The value to store in width. */ public void setWidth(double width) { this.width=width; } /** @param airconditioner-AirConditioner object. */ public void setShade(String amountOfShade) { this.amountOfShade=amountOfShade; } /** The setCoolingCapacity method stores a value in the cooling_capacity field. @param cooling_capacity The value to store in cooling_capacity. */ public void setAirConditioner(AirConditioner airconditioner) { airConditioner=new AirConditioner(airconditioner); } public String getName() { return name; } /** The getLength method returns a Room object's length. @return The value in the length field. */ public double getLength() { return length; } /** The getWidth method returns a Room object's width. @return The value in the width field. */ public double getWidth() { return width; } /** The getShade method returns a Room object's shade. @return The value in the shade field. */ public String getShade() { return amountOfShade; } /** The getCoolingCapacity method returns a Room object's cooling_capacity. @return The value in the cooling_capacity field. */ public double getCoolingCapacity() { return cooling_capacity; } /** The AirCondition method object's area. @return The reference to copy of this Room's AirConditioner object. */ public AirConditioner getAirConditioner() { return new AirConditioner(airCondtioner); } /** The getArea method returns a Room object's area. @return The product of length times width. */ public double getArea() { return (length*width); } /** Method for cooling capacity @return the capacity */ public double calCoolingCapacity() { double area=getArea(); double capacity; if(area<250) { capacity=5500; } else if(area>=250&&area<=500) { capacity=10000; } else if(area>500&&area<1000) { capacity=17500; } else capacity=24000; if(amountOfShade.equalsIgnoreCase("Little")) { capacity=capacity+capacity* LITTLE_COOLING_CAPACITY; } else if(amountOfShade.equalsIgnoreCase("Abundant")) { capacity=capacity-capacity*ABUNDANT_COOLING_CAPACITY; } return capacity; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
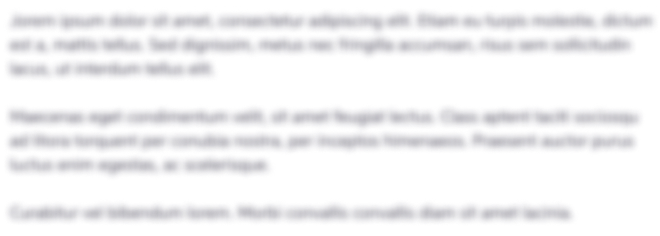
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started