Question
I need help on this C++ program. I have provided sample output below the program. The variable 'N' must be an int datatype but the
I need help on this C++ program. I have provided sample output below the program. The variable 'N' must be an int datatype but the findAverageScore( ) function should return a double as shown in the sample output. How can I accomplish this?
/* EPP Review Part 1 Gymnastics Competition. */
#include
using namespace std;
// length is the number of elements in the array int findAverageScore(int scores[], int length ){ // create variable to store highest and lowest scores int highest = 0; int lowest = scores[0]; int total;
for (int i = 0; i < length; i++) { total += scores[i];
// Check to see if score is greater than the previous highest score if (scores[i] >= highest) { highest = scores[i]; } // Check to see if score is less than the previous lowest score if (scores[i] <= lowest) { lowest = scores[i]; } }
total = total - highest - lowest; return total / (length - 2); }
int main(){ int N; //THIS must be an int but averageScore //should be double. int maxScore = 0; int contestant; int highestContestant;
printf("Enter the number of judges: "); cin >> N; // Array of scores to be stored int* scores = new int[N];
printf("Number of judges entered: %d ", N);
while(true){ puts("Enter contestant number:"); cin >> contestant;
if(contestant > 0){
puts("Enter score: "); for (int i = 0; i < N; i++) { cin >> scores[i]; } int averageScore = findAverageScore(scores, N); if (averageScore>=maxScore) { highestContestant = contestant; maxScore = averageScore; } printf("Average score: %d ", averageScore);
} else { printf("Contestant %d had the highest score. ", highestContestant); break; } }
return 0; }
************* SAMPLE OUTPUT *******************
Number of Judges: 5
34 7 5 3 7 6
Contestant 34 6
28 8 5 8 10 7
Contestant 28 7.666666667
3 5 5 3 4 4
Contestant 3 4.333333333
1
Contestant 28 had the highest score.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
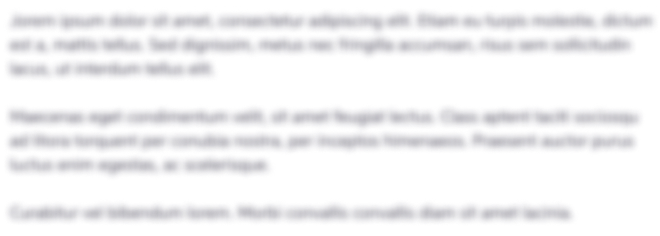
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started